In order to create test data I need to be able to create and Approve POs via code. Eventually I will use this in a Customization Plugin for populating the instance with documents to run Integration tests against. Right now I’m just trying to create 1 PO with 1 line item and get it to the open state. Creating the PO and removing the PO from hold has been pretty straightforward, the piece I’m having difficulty with is Approving the PO.
Here is my test method which I’m trying to get to work.
public PXAction<ISPSOutboundPOLog> createPosTest;
[PXUIField(DisplayName = "Create POs Test", MapEnableRights =
PXCacheRights.Update, MapViewRights = PXCacheRights.Update)]
[PXButton]
public virtual IEnumerable CreatePosTest(PXAdapter adapter)
{
var PoOrderEntryGraph = PXGraph.CreateInstance<POOrderEntry>();
// Create a new Purchase Order header
POOrder newPO = new POOrder
{
OrderType = POOrderType.RegularOrder,
VendorID = 6995, // AAVENDOR
OrderDesc = "New Purchase Order",
OrderDate = PXTimeZoneInfo.Now,
ShipVia = "LOCAL"
};
newPO = PoOrderEntryGraph.Document.Insert(newPO);
// Create a line item for the Purchase Order
POLine newLine = new POLine
{
InventoryID = 692, // AALEGO500
OrderQty = 7,
UOM = "EA"
};
// Insert the line item into the Purchase Order
newLine = PoOrderEntryGraph.Transactions.Insert(newLine);
// Associate the line item with the Purchase Order
if (newLine != null)
{
newLine.OrderNbr = newPO.OrderNbr;
newLine = PoOrderEntryGraph.Transactions.Update(newLine);
}
// release the PO from hold
PoOrderEntryGraph.releaseFromHold.Press();
// Approve the PO [THIS IS THE PART GIVING ME TROUBLE]
newPO.Status = POOrderStatus.Open;
newPO.Approved = true;
PoOrderEntryGraph.Document.Update(newPO);
PoOrderEntryGraph.Actions.PressSave();
return adapter.Get();
}
Everything works as expected until I try to Approve the PO and update the document. Specifically, when PoOrderEntryGraph.Document.Update(newPO) is called, the following popup appears.
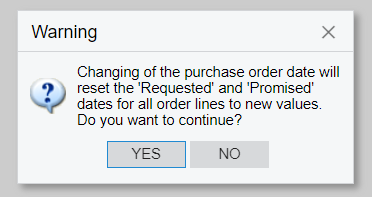
When I click YES or NO the PXAction starts all over from the beginning and that popup window appears again. This goes on forever until I click the exit button. From what I’ve read online and observed debugging the code, it seems the exception that is triggering the popup window is also causing the PXAction to restart.
If I remove the line item the prompt goes away and the PO is created and moved into the Open status correctly. This makes since as the popup window is specifically about changing the lines dates. But I need line items on the PO so that is not a viable solution. Furthermore I want to run this code on Publish via a Customization Plugin, which currently causes an error since it expects user input and that seem to not be allowed when publishing.
Solutions I’ve tried and failed(I might have implemented them incorrectly since most of this is new to me)
- I tried using the PoOrderEntryGraph.Approval.Approve(newPO); function, this only still has the same issue of the popup window but it doesn’t actually change the POs status to Open even if it does run.
- I tried making it a long running process since I thought maybe that would allow me to skip the popup window. Nope.
- I tried changing the values directly via the cache to avoid events, the popup window still came up though.
- I tried setting the POOrder’s Status = POOrderStatus.Open, Hold = false and Approved = true. But this only created the PO in a “Pending Approval” state not “Open” which I am trying to get it to
Any advice or solutions would be greatly appreciated!
Thanks,
Philip Engesser