I declared a method in the SOOrderEntry graph as follows.
public void UpdateSalesOrder(PXCache cache, PXFieldUpdatedEventArgs e, string blanketOrderNumber = null)
{
PXTrace.WriteError("Field_updated");
// Tenant selection
if (PX.Data.Update.PXInstanceHelper.CurrentCompany == 3)
{
// Determine the appropriate SOOrder row
SOOrder row = null;
if (!string.IsNullOrEmpty(blanketOrderNumber))
{
// Fetch the specific SOOrder record matching the blanketOrderNumber
row = PXSelect<SOOrder,
Where<SOOrder.orderType, Equal<Required<SOOrder.orderType>>,
And<SOOrder.orderNbr, Equal<Required<SOOrder.orderNbr>>>>>
.Select(cache.Graph, "BL", blanketOrderNumber);
if (row == null)
{
PXTrace.WriteError($"No SOOrder found with OrderNbr: {blanketOrderNumber} and OrderType: BL.");
return;
}
else
{
PXTrace.WriteInformation(row.OrderNbr);
SOLine line = SOLines.SelectSingle(row.OrderNbr);
if (line == null)
{
PXTrace.WriteError("SOLine is null.");
}
else
{
PXTrace.WriteInformation($"SOLine is not null. Open Qty: {line.OpenQty}, Shipped Qty: {line.ShippedQty}");
}
}
}
else
{
// If no blanket order number is provided, use the row from the event args
row = (SOOrder)e.Row;
if (row == null)
{
PXTrace.WriteError("SOOrder row is null.");
return;
}
// Ensure we're working with a blanket order
if (row.OrderType != "BL")
{
PXTrace.WriteError("OrderType is not 'BL'. Exiting method.");
return;
}
}
}
}
Then, I attempted to call this method from the POReceiptEntry graph as shown below, when the POReceipt's status changed to 'Released'.
protected void POReceipt_Status_FieldUpdated(PXCache cache, PXFieldUpdatedEventArgs e)
{
PXTrace.WriteInformation("Entering POReceipt_Status_FieldUpdated");
try
{
var row = (POReceipt)e.Row;
if (row == null)
{
PXTrace.WriteError("POReceipt row is null.");
return;
}
PXTrace.WriteInformation($"POReceipt.Status: {row.Status}");
if (row.Status != "R")
{
PXTrace.WriteInformation("POReceipt.Status is not 'R'. Exiting method.");
return;
}
// Log key variable values
PXTrace.WriteInformation($"Fetching POReceiptLine for ReceiptNbr: {row.ReceiptNbr}");
POReceiptLine poReceiptLine = POReceiptLineView.SelectSingle("RT", row.ReceiptNbr);
if (poReceiptLine == null)
{
PXTrace.WriteError("No matching POReceiptLine found.");
}
else
{
PXTrace.WriteInformation($"Found POReceiptLine: ReceiptType={poReceiptLine.ReceiptType}, LineNbr={poReceiptLine.LineNbr}");
}
PXTrace.WriteInformation($"Fetching POOrder for POType: {poReceiptLine?.POType}, PONbr: {poReceiptLine?.PONbr}");
POOrder poOrder = POOrder.PK.Find(Base, poReceiptLine?.POType, poReceiptLine?.PONbr);
if (poOrder == null)
{
PXTrace.WriteError("No matching POOrder found.");
}
else
{
PXTrace.WriteInformation($"Found POOrder: OrderType={poOrder.OrderType}, OrderNbr={poOrder.OrderNbr}");
}
PXTrace.WriteInformation($"Fetching SOLine for SOOrderNbr: {poOrder?.SOOrderNbr}");
SOLine soLine = SOLineView.SelectSingle("SO", poOrder?.SOOrderNbr);
if (soLine != null && !string.IsNullOrEmpty(soLine.BlanketNbr))
{
PXTrace.WriteInformation($"Found SOLine with BlanketNbr: {soLine.BlanketNbr}");
// Log the transition to the next method
PXTrace.WriteInformation("Calling UpdateSalesOrder");
var soOrderEntryGraph = PXGraph.CreateInstance<SOOrderEntry>();
var soOrderEntryExt = soOrderEntryGraph.GetExtension<SOOrderEntry_Extension>();
soOrderEntryExt.UpdateSalesOrder(Base.Caches[typeof(SOOrder)], null, soLine?.BlanketNbr);
PXTrace.WriteInformation("UpdateSalesOrder completed");
}
else
{
PXTrace.WriteError("No SOLine found or no BlanketNbr present to update.");
}
}
catch (Exception ex)
{
PXTrace.WriteError($"Error in POReceipt_Status_FieldUpdated: {ex.Message}");
PXTrace.WriteError($"StackTrace: {ex.StackTrace}");
throw; // Re-throw the exception after logging
}
finally
{
PXTrace.WriteInformation("Exiting POReceipt_Status_FieldUpdated");
}
}
However, after inserting this custom logic, the POReceipt is not getting released and is showing the following error.”A data corruption state has been detected. You cannot save the changes. Copy the data you have entered and reload the page. Date and Time: 2024-09-02T08:59:44; IncidentID: 7b75179c-407e-4b5c-8987-4356b86ef67e; Name: Aggregate Validation: PX.Objects.SO.SOOrder+openLineCntr. You can view detailed information about the issue on the System Events tab of the System Monitor (SM201530) form.”
Please check the below screenshot of the trace also.
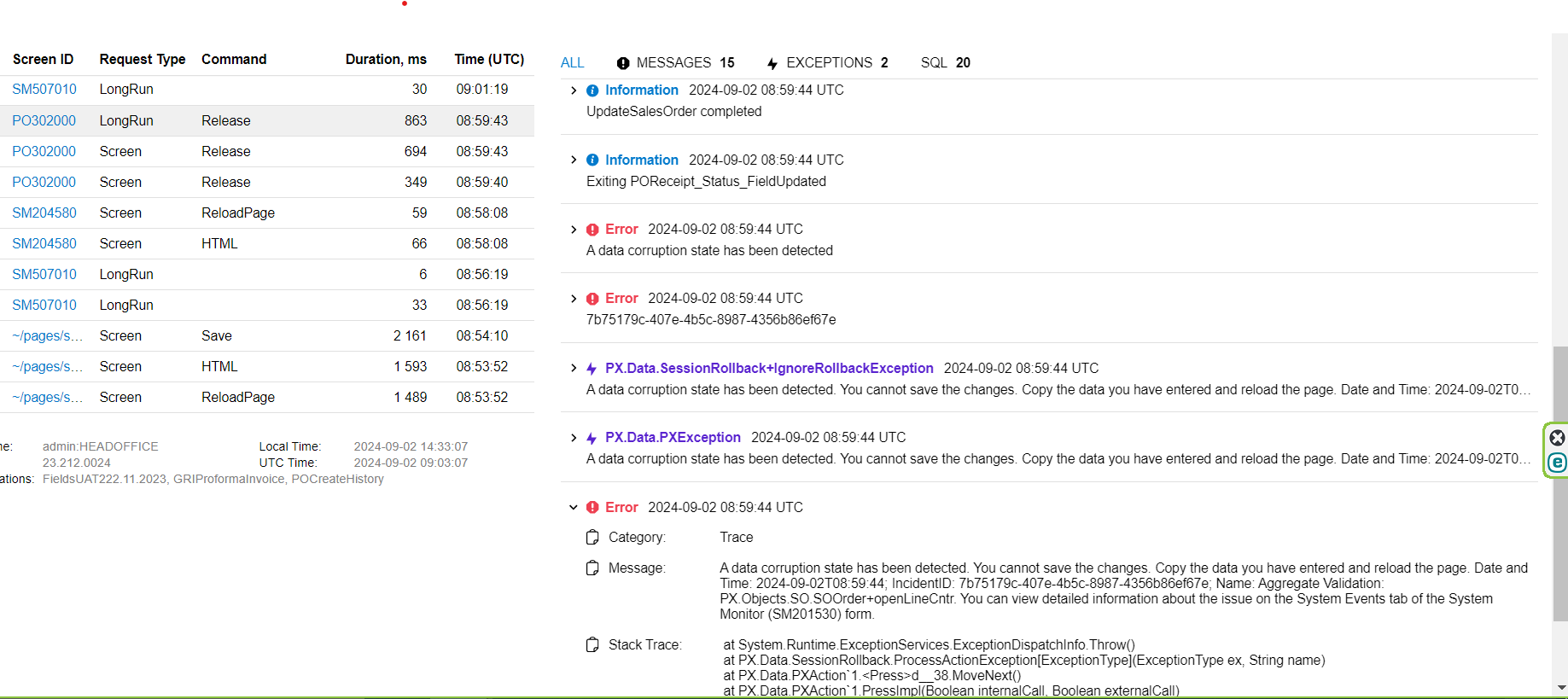
Could I get any suggestions on how to resolve this issue?