Hello again,
I have a custom field INPIDetailExt.usrAbsVarQty that gets populated correctly by both the PI Review and Scan and Count screens. However, a change to the field by Scan and Count does not reflect accurately on PI Review, even though Scan and Count changes the physical quantity on both forms.
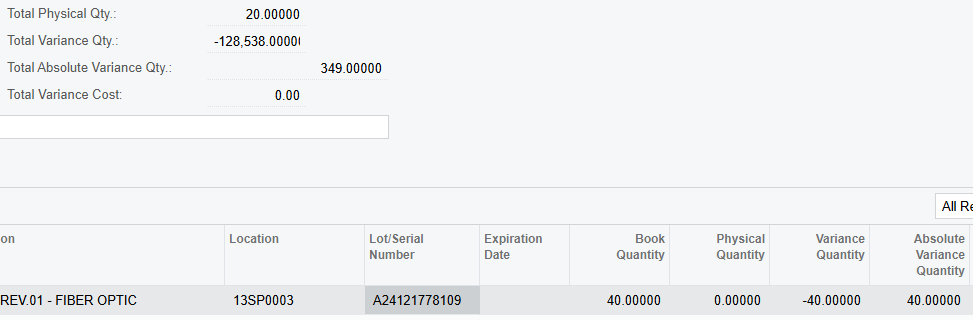
When I put in the data for serial number ---78109 into Scan and Count, this is the result:
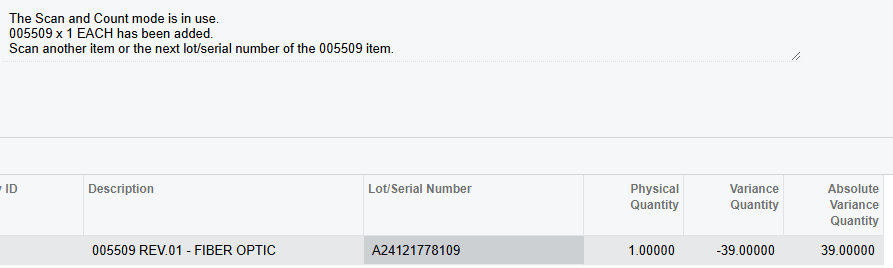
But later, on PI Review, the absolute variance quantity is still at 40:
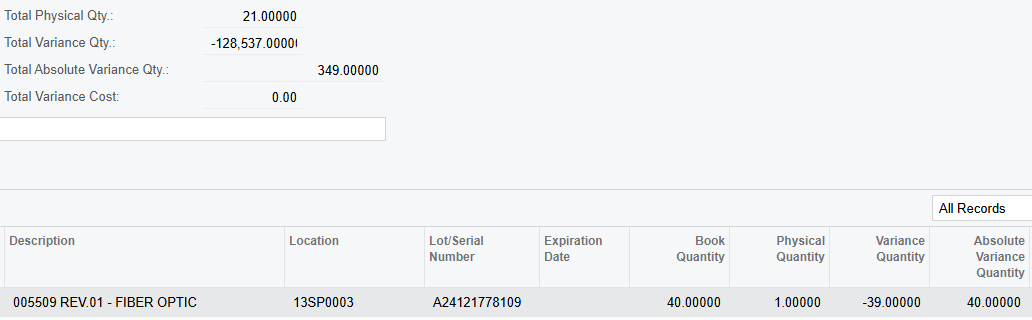
I figure this has something to do with how PXCache works, but even after looking at a lot of documentation and searching the business logic code for how the other fields are updated/saved, I haven’t gotten any of my ideas to work. I am also suspecting incorrect inheritance/extension on my part.
Here is my code for the various screens and sources I’ve tried:
Host
using System;
using System.Collections.Generic;
using PX.Common;
using PX.Data;
using PX.Data.BQL;
using PX.Data.BQL.Fluent;
using PX.BarcodeProcessing;
//using WMSBase = WarehouseManagementSystem<INScanCount, INScanCount.Host>;
using PX.Objects;
using PX.Objects.IN.WMS;
namespace PX.Objects.IN.WMS
{
public class INScanCount_Host_Extension : PXGraphExtension<PX.Objects.IN.INPICountEntry>
{
#region Event Handlers
protected void INPIDetail_PhysicalQty_FieldUpdated(PXCache cache, PXFieldUpdatedEventArgs e)
{
var row = (INPIDetail)e.Row;
if (row is null) return;
decimal varianceQuantityVariable = (decimal)(row.PhysicalQty - row.BookQty);//how variance quantity is initially calculated
decimal absoluteVarianceQuantity = Math.Abs(varianceQuantityVariable);
cache.SetValueExt<INPIDetailExt.usrAbsVarQty>(row, absoluteVarianceQuantity);
return;
}
#endregion
}
}
-----------------------------------------
INPICountEntry (Physical Inventory Count)
using System;
using System.Collections;
using System.Collections.Generic;
using PX.Data;
using PX.Objects.CS;
using PX.Objects.GL;
using PX.Objects.IN.PhysicalInventory;
using PX.Objects.RQ;
using PX.Objects;
using PX.Objects.IN;
namespace PX.Objects.IN
{
//this is for the Physical Inventory Count screen, where there are multiple individual input boxes at the top
//INPICountEntry is what Scan and Count extends in the source code (Scan and Get inherits Methods and Views from INPICountEntry)
public class INPICountEntry_Extension : PXGraphExtension<PX.Objects.IN.INPICountEntry>
{
#region Event Handlers
protected void INPIDetail_PhysicalQty_FieldUpdated(PXCache cache, PXFieldUpdatedEventArgs e)
{
INPIDetail detailRow = e.Row as INPIDetail;
if (detailRow is null) return;
INPIDetailExt inpiDetailExtVar = PXCache<INPIDetail>.GetExtension<INPIDetailExt>(detailRow);
decimal varianceQuantityVariable = (decimal)(detailRow.PhysicalQty - detailRow.BookQty);//how variance quantity is initially calculated
decimal absoluteVarianceQuantity = Math.Abs(varianceQuantityVariable);
cache.SetValueExt<INPIDetailExt.usrAbsVarQty>(detailRow, absoluteVarianceQuantity);
return;
}
#endregion
}//end of Class
}//end of namespace
-----------------------------------------
INPIReview (Physical Inventory Review) this one already works as intended
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using PX.Common;
using PX.Data;
using PX.Data.BQL;
using PX.Data.BQL.Fluent;
using PX.Objects.CM;
using PX.Objects.Common.Extensions;
using PX.Objects.CS;
using PX.Objects.IN.PhysicalInventory;
using PX.Data.WorkflowAPI;
using PX.Objects;
using PX.Objects.IN;
namespace PX.Objects.IN
{
//this is for the PI Review screen, where data can be entered manually line by line
public class INPIReview_Extension : PXGraphExtension<PX.Objects.IN.INPIReview>
{
protected void INPIDetail_PhysicalQty_FieldUpdated(PXCache cache, PXFieldUpdatedEventArgs e)
{
var row = (INPIDetail)e.Row;
if (row == null) return;
decimal varianceQuantityVariable = (decimal)(row.PhysicalQty - row.BookQty);//how variance quantity is initially calculated
decimal absoluteVarianceQuantity = Math.Abs(varianceQuantityVariable);
cache.SetValueExt<INPIDetailExt.usrAbsVarQty>(row, absoluteVarianceQuantity);
return;
}
}
}
-----------------------------------------
INScanCount
using System;
using System.Collections.Generic;
using PX.Common;
using PX.Data;
using PX.Data.BQL;
using PX.Data.BQL.Fluent;
using PX.BarcodeProcessing;
//using WMSBase = WarehouseManagementSystem<INScanCount, INScanCount.Host>;
using PX.Objects;
using PX.Objects.IN.WMS;
namespace PX.Objects.IN.WMS
{
//this is for the Scan and Count screen that the mobile scanners use, this is the most important one
public class INScanCount_Extension : INScanCount.ScanExtension
{
#region Event Handlers
protected void INPIDetail_PhysicalQty_FieldUpdated(PXCache cache, PXFieldUpdatedEventArgs e)
{
INPIDetail detailRow = e.Row as INPIDetail;
if (detailRow is null) return;
INPIDetailExt inpiDetailExtVar = PXCache<INPIDetail>.GetExtension<INPIDetailExt>(detailRow);
decimal varianceQuantityVariable = (decimal)(detailRow.PhysicalQty - detailRow.BookQty);//how variance quantity is initially calculated
decimal absoluteVarianceQuantity = Math.Abs(varianceQuantityVariable);
cache.SetValueExt<INPIDetailExt.usrAbsVarQty>(detailRow, absoluteVarianceQuantity);
return;
}
#endregion
}
}