Hi everyone,
I created a new customised processing screen based on the logic of the SO505000: a filter provides a list of actions; when the user selects one of them, the invoices affected by that action are displayed in a grid below. Finally, a dedicated method is invoked, depending on the action chosen, when the user clicks on the ‘Process’ button.
This works very well, but I've run into a problem: on a local instance, the processing dialog appears every time the ‘Process’ button is pressed, and this is the expected behaviour
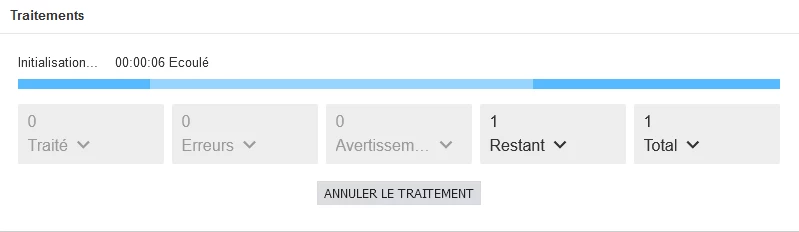
However, on a remote instance (saas), the process dialog appears just once, on the first execution after publishing. Thereafter, it doesn’t appear anymore even if the process is actually executed. I don't see wha's wrong...
Below, the DAC used to describe the filter :
public class ARInvoiceEIprocessFilter : IBqlTable
{
#region Action
[PXUIField(DisplayName = "Action")]
[PXDefault(ARInvoiceEIProcess.DefaultValue)]
[PXStringList(
new string[] { ARInvoiceEIProcess.DefaultValue, ARInvoiceEIProcess.SendInvoicesToOdCode, ARInvoiceEIProcess.CheckInvoicesErrors, ARInvoiceEIProcess.LifeCycleStatus, ARInvoiceEIProcess.ElectronicStatusCashInHand},
new string[] { Messages.DefaultValue, Messages.SendElectronicInvoices, Messages.CheckInvoicesErrors, Messages.GetLifeCycleStatus, Messages.SendElectronicStatusCashInHand}
)]
public virtual string Action { get; set; }
public abstract class action : BqlString.Field<action> { }
#endregion
#region StartDate
[PXDBDate]
[PXDefault]
[PXUIField(DisplayName = "Start Date", Visibility = PXUIVisibility.SelectorVisible, Required = false)]
public virtual DateTime? StartDate { get; set; }
public abstract class startDate : BqlDateTime.Field<startDate> { }
#endregion
#region EndDate
[PXDBDate]
[PXDefault(typeof(AccessInfo.businessDate))]
[PXUIField(DisplayName = "End Date", Visibility = PXUIVisibility.SelectorVisible)]
public virtual DateTime? EndDate { get; set; }
public abstract class endDate : BqlDateTime.Field<endDate> { }
#endregion
#region CustomerID
[PXUIField(DisplayName = "Customer")]
[Customer(DescriptionField = typeof(Customer.acctName))]
public virtual int? CustomerID { get; set; }
public abstract class customerID : BqlInt.Field<customerID> { }
#endregion
#region DocType
public abstract class docType : BqlString.Field<docType> { }
[PXDBString(3, IsFixed = true)]
[PXDefault()]
[EInvoiceDocType.List()]
[PXUIField(DisplayName = "Doc. type", Visibility = PXUIVisibility.SelectorVisible, Enabled = true)]
[PXFieldDescription]
public virtual string DocType { get; set; }
#endregion
#region RefNbr
public abstract class refNbr : BqlString.Field<refNbr> { }
[PXDBString(15, IsUnicode = true, InputMask = "")]
[PXDefault()]
[PXUIField(DisplayName = "Reference Nbr.", Visibility = PXUIVisibility.SelectorVisible, TabOrder = 1)]
[PXSelector(typeof(Search<ARInvoice.refNbr, Where<ARInvoice.docType, Equal<Current<docType>>, And<ARInvoice.released.IsEqual<True>>>>), Filterable = true)]
[PXFieldDescription]
public virtual string RefNbr { get; set; }
#endregion
#region IncludeDocumentsAlreadySend
public abstract class includeDocumentsAlreadySend : BqlBool.Field<includeDocumentsAlreadySend> { }
[PXBool]
[PXDefault(false, PersistingCheck = PXPersistingCheck.Nothing)]
[PXUIField(DisplayName = "Include documents already send")]
public virtual bool? IncludeDocumentsAlreadySend { get; set; }
#endregion
#region CegEStatus
[PXDBString(50, IsUnicode = true)]
[PXDefault(PersistingCheck = PXPersistingCheck.Nothing)]
[PXUIField(DisplayName = "Electronic Status")]
[EiStatuses.List]
public virtual string CegEStatus { get; set; }
public abstract class cegEStatus : BqlString.Field<cegEStatus> { }
#endregion
#region CegElectronicSendingDate
[PXDBDate]
[PXUIField(DisplayName = "Electronic sending date")]
public virtual DateTime? CegElectronicSendingDate { get; set; }
public abstract class cegElectronicSendingDate : BqlDateTime.Field<cegElectronicSendingDate> { }
#endregion
#region CegLastElectronicUpdate
public abstract class cegLastElectronicUpdate : BqlDateTime.Field<cegLastElectronicUpdate> { }
[PXDBDate]
[PXUIField(DisplayName = "Electronic status modified on")]
public virtual DateTime? CegLastElectronicUpdate { get; set; }
#endregion
}
Herte is the processing view :
[PXFilterable]
public PXFilteredProcessingJoin<ARInvoice, ARInvoiceEIprocessFilter,
InnerJoin<Customer, On<ARInvoice.customerID, Equal<Customer.bAccountID>>>,
Where<True, Equal<True>>,
OrderBy<Desc<ARInvoice.refNbr>>> RecordsToProcess;
The content of this view is overriden since it depends on the action selected in the filter :
public virtual IEnumerable<PXResult<ARInvoice, Customer>> recordsToProcess()
{
if (_actionChanged)
{
RecordsToProcess.Cache.Clear();
_actionChanged = false;
}
int startRow = PXView.StartRow;
int totalRows = 0;
PXSelectBase<ARInvoice> select = DataProvider.GetGridRequest(Filter.Current, this, Filter.Current.Action);
DataProvider.ApplyAdditionalFilters(select, Filter.Current);
foreach (PXResult<ARInvoice, Customer> res in
select.View.Select(PXView.Currents, null, PXView.Searches, PXView.SortColumns, PXView.Descendings, PXView.Filters, ref startRow, PXView.MaximumRows, ref totalRows)
.Cast<PXResult<ARInvoice, Customer>>())
{
ARInvoice item = res;
Customer customer = res;
RestoreSelectedFlag(item);
yield return new PXResult<ARInvoice, Customer>(item, customer);
}
PXView.StartRow = 0;
}
private void RestoreSelectedFlag(ARInvoice item)
{
ARInvoice cached = (ARInvoice)RecordsToProcess.Cache.Locate(item);
if (cached != null)
{
item.Selected = cached.Selected;
}
}
The process action is set like this :
public virtual void _(Events.RowSelected<ARInvoiceEIprocessFilter> e)
{
string actionCode = Filter.Current.Action;
bool isNotDefaultActionCode = !DefaultValue.Equals(actionCode);
if (isNotDefaultActionCode)
{
_codeToActionMap.TryGetValue(actionCode, out ProcessListDelegate action);
RecordsToProcess.SetProcessDelegate(action);
RecordsToProcess.View.RequestRefresh();
}
EnableFilterFields(e.Cache, enable: isNotDefaultActionCode);
EnableProcessButtons(enable: isNotDefaultActionCode);
}
And the action that is invoked is described below. It does actually nothing cause I wanted to be sure that the instructions I was initally running in it were not the source of my issue.
public static void Test(List<ARInvoice> selectedInvoices)
{
ARInvoiceEIProcess processGraph = CreateInstance<ARInvoiceEIProcess>();
PXLongOperation.StartOperation(processGraph, delegate ()
{
UploadContext uploadContext = new UploadContext(processGraph, processGraph.OdDataProvider)
{
RowIndex = 0
};
foreach (ARInvoice arInvoice in selectedInvoices)
{
uploadContext.ARInvoice = arInvoice;
uploadContext.AnomalyHandler.ResetCurrentErrorsCount();
try
{
// Do nothing
}
catch (Exception e)
{
uploadContext.AnomalyHandler.AddError(e);
}
finally
{
ProcessSendResult(uploadContext, arInvoice);
}
uploadContext.RowIndex++;
}
});
}
private static void ProcessSendResult(UploadContext UploadContext, ARInvoice arInvoice, FileInfo generatedFacturXFileInfo)
{
if (UploadContext.AnomalyHandler.HasCurrentErrors())
{
UploadContext.AnomalyHandler.GetAnomalies().ForEach(a => PXProcessing<ARInvoice>.SetError(UploadContext.RowIndex, a.Message));
}
else
{
AddFacturXAsNoteDoc(UploadContext, arInvoice, generatedFacturXFileInfo);
PXProcessing<ARInvoice>.SetInfo(UploadContext.RowIndex, ActionsMessages.RecordProcessed);
PXProcessing<ARInvoice>.SetProcessed();
}
}