Hello
I want to override value of field Asset Class and Department of data class FAAccrualTran (screen FA504500)
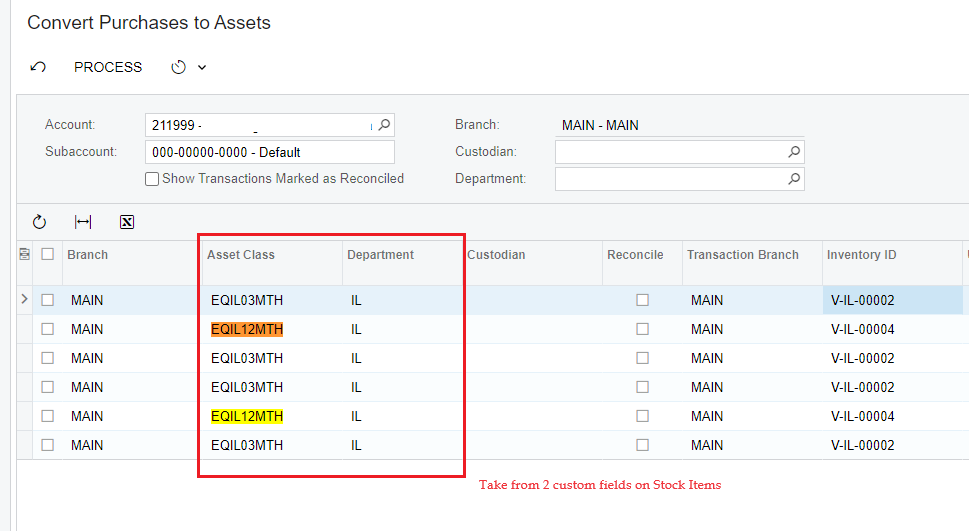
I implemented event handler RowInserting to set value for two fields: Asset Class and Department as following:
protected void FAAccrualTran_RowInserting(PXCache cache, PXRowInsertingEventArgs e, PXRowInserting InvokeBaseHandler)
{
if (InvokeBaseHandler != null)
InvokeBaseHandler(cache, e);
var row = (FAAccrualTran)e.Row;
if (row != null && row.GLTranInventoryID != null)
{
InventoryItem inventory = PXSelect<InventoryItem, Where<InventoryItem.inventoryID, Equal<Required<InventoryItem.inventoryID>>>>.Select(Base, row.GLTranInventoryID);
if (inventory != null)
{
var inventoryItemExt = PXCache<InventoryItem>.GetExtension<InventoryItemExt>(inventory);
if (inventoryItemExt != null && inventoryItemExt.UsrDepartment != null)
{
cache.SetValueExt<FAAccrualTran.department>(row, inventoryItemExt.UsrDepartment.Trim());
}
if (inventoryItemExt != null && inventoryItemExt.UsrAssetClass != null)
{
cache.SetValueExt<FAAccrualTran.classID>(row, inventoryItemExt.UsrAssetClass.Trim());
}
}
}
}
Then I create a Schedule Automation as use these field as condition. However, the value of these field aren’t override
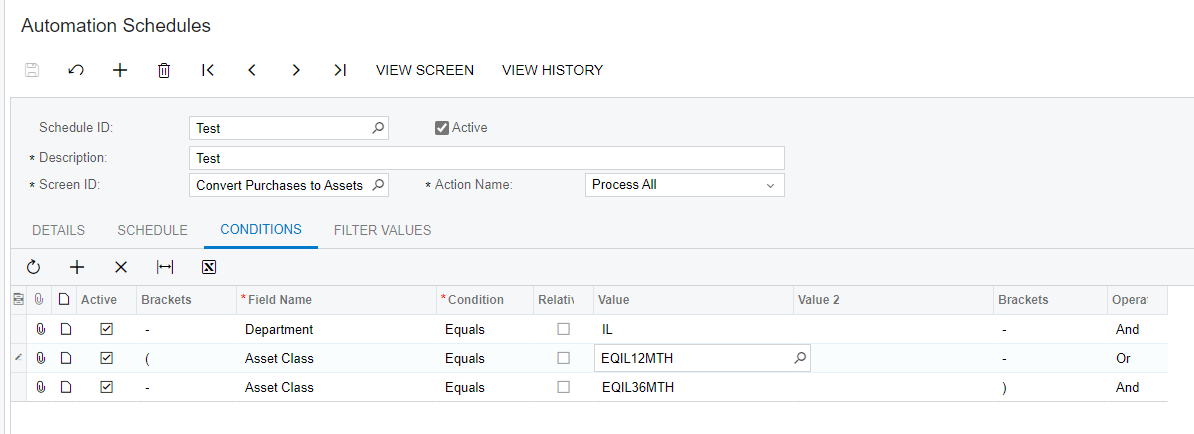
Do you know how to achieve the goal: set value of field Asset Class and Department which is taken from Stock Item and could use it as trigger condition for Schedule Automation
Tx,
Khoi
Best answer by darylbowman
View original