I have customized purchase orders screen, add an extension to POLine DAC and add two fields.
public class POLineExt : PXCacheExtension<PX.Objects.PO.POLine>
{
#region UsrInventoryItemClassID
[PXInt]
[PXUIField(DisplayName="ItemClass")]
[PXDBScalar(typeof(
Search<InventoryItem.itemClassID,
Where<InventoryItem.inventoryID, Equal<POLine.inventoryID>>>))]
public virtual int? UsrInventoryItemClassID { get; set; }
public abstract class usrInventoryItemClassID : PX.Data.BQL.BqlInt.Field<usrInventoryItemClassID> { }
#endregion
#region UsrInventoryItemClassCD
[PXString(32)]
[PXUIField(DisplayName="Item Class Description")]
[PXDBScalar(typeof(
Search2<INItemClass.itemClassCD,
InnerJoin<InventoryItem, On<InventoryItem.itemClassID, Equal<INItemClass.itemClassID>>>,
Where<InventoryItem.inventoryID, Equal<POLine.inventoryID>>>))]
public virtual string UsrInventoryItemClassCD { get; set; }
public abstract class usrInventoryItemClassCD : PX.Data.BQL.BqlString.Field<usrInventoryItemClassCD> { }
#endregion
}
Dispaly these two in the grid,
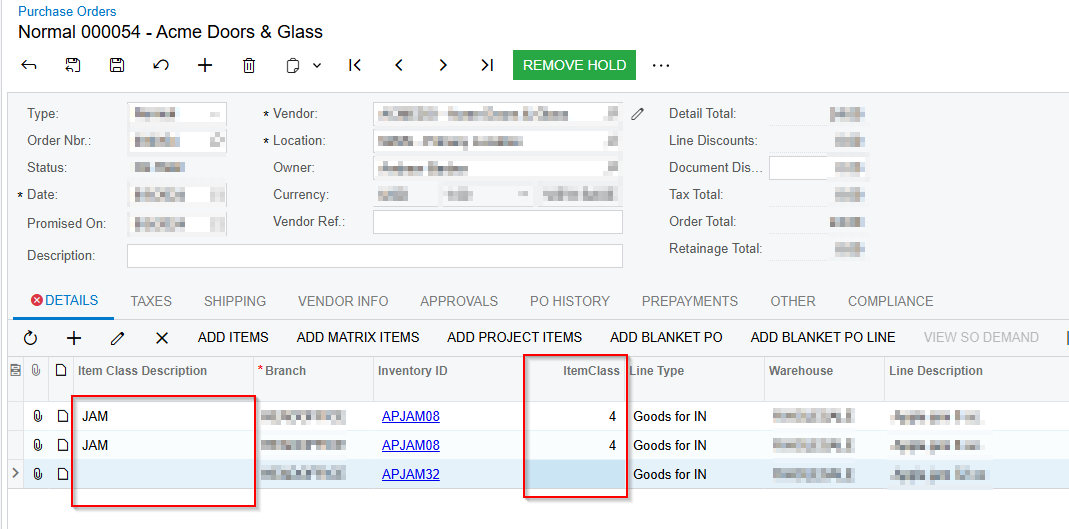
When I select the Inventory ID, I need to load the item class and item class description according to [PXDBScalar] query. So, I wrote an event as below. but it is not work properly. to update the UI I has to reload the whole website. How to fix that issue?
protected void POLine_InventoryID_FieldUpdated(PXCache cache, PXFieldUpdatedEventArgs e)
{
//Base.Transactions.Cache.Clear();
//Base.Transactions.Cache.ClearQueryCache();
//Base.Transactions.View.RequestRefresh();
//cache.SetValueExt<POLineExt.usrInventoryItemClassID>(e.Row, 4);
//cache.SetValueExt<POLineExt.usrInventoryItemClassCD>(e.Row, "JAM");
if (e.Row == null) return;
var row = (POLine)e.Row;
var tranView = row.GetExtension<POLineExt>();
cache.SetValueExt<POLineExt.usrInventoryItemClassID>(row, tranView.UsrInventoryItemClassID);
cache.Update(row);
}