Hello,
We’ve recently upgraded from Acumatica 2023 R1 to 2024 R1 and are encountering an issue involving the Sales Order and Shipment modules. Specifically, we’ve noticed that the CustomerOrderNbr
field, which is typically copied from a Sales Order to a Shipment during shipment creation, is not consistently transferred.
This behaviour is managed by Acumatica’s core code and previously worked as expected on 2023 R1:
public virtual bool SetShipmentFieldsFromOrder(SOOrder order, SOShipment shipment, int? siteID, DateTime? shipDate, string operation, SOOrderTypeOperation orderOperation, bool newlyCreated)
{
if (newlyCreated)
{
// Removed all of the other fields, only keeping below line for brevity
[...]
shipment.CustomerOrderNbr = order.CustomerOrderNbr;
[...]
if(string.IsNullOrEmpty(shipment.ShipmentDesc))
shipment.ShipmentDesc = order.OrderDesc;
return true;
}
else
{
// In our case, it's all newly created shipments, so this portion of the code can be ignored.
[...]
}
}
Problem:
When a shipment is created from a Sales Order, the CustomerOrderNbr
is not always being copied to the shipment record. This issue occurs sporadically, without any error messages or clear patterns to reproduce the problem. We have multiple companies set up in our Acumatica instance, and the issue varies by company:
- For 2 out of 3 companies, the issue happens occasionally.
- For the 3rd company, the issue happens most of the time.
Just to point out, as can be seen in the code snippet above, the OrderDesc is copied over to the ShipmentDesc, and I can confirm, in the cases where the CustomerOrderNbr is null, the ShipmentDesc is indeed populated. So we know the code must’ve run through this if statement.
Environment:
- Version: Acumatica 2024 R1
- Modules affected: Sales Order & Shipment
Additional context:
We do have customisation around the core functionality, but we are not making changes to the CustomerOrderNbr
field itself, so we can’t see how our customisations would affect this specific behaviour, especially since the OrderDesc is indeed copied over to the ShipmentDesc.
#region SetShipmentFieldsFromOrder
public delegate Boolean SetShipmentFieldsFromOrderDelegate(SOOrder order, SOShipment shipment, Nullable<Int32> siteID, Nullable<DateTime> shipDate, String operation, SOOrderTypeOperation orderOperation, Boolean newlyCreated);
[PXOverride]
public Boolean SetShipmentFieldsFromOrder(SOOrder order, SOShipment shipment, Nullable<Int32> siteID, Nullable<DateTime> shipDate, String operation, SOOrderTypeOperation orderOperation, Boolean newlyCreated, SetShipmentFieldsFromOrderDelegate baseMethod)
{
if (newlyCreated)
{
if (order != null)
{
SOOrderExt orderExt = order.GetExtension<SOOrderExt>();
if (orderExt != null)
{
if (shipment != null)
{
SOShipmentExt shipmentExt = shipment.GetExtension<SOShipmentExt>();
if (shipmentExt != null)
{
shipmentExt.UsrDeliveryInstructions = orderExt.UsrDeliveryInstructions;
shipmentExt.UsrOrderInstructions = orderExt.UsrOrderInstructions;
shipmentExt.UsrPargoPickupPoint = orderExt.UsrPargoPickupPoint;
shipmentExt.UsrPriority = CalculatePriority(order, shipment);
if (!string.IsNullOrEmpty(orderExt.UsrDispatchByDateTime.ToString()))
{
bool isPrimaryWarehouse = IsPrimaryWarehouse(order, siteID);
if (isPrimaryWarehouse)
{
shipmentExt.UsrDispatchByDateTime = orderExt.UsrDispatchByDateTime;
shipmentExt.UsrDispatchNoEarlierThanDateTime = orderExt.UsrDispatchNoEarlierThanDateTime;
shipmentExt.UsrEarliestDateTime = orderExt.UsrEarliestDateTime;
shipmentExt.UsrTargetDateTime = orderExt.UsrTargetDateTime;
shipmentExt.UsrPromiseDateTime = orderExt.UsrPromiseDateTime;
}
else
{
shipmentExt.UsrDispatchByDateTime = orderExt.UsrRerouteDispatchByDateTime;
shipmentExt.UsrDispatchNoEarlierThanDateTime = orderExt.UsrRerouteDispatchNoEarlierThanDateTime;
shipmentExt.UsrEarliestDateTime = orderExt.UsrRerouteEarliestDateTime;
shipmentExt.UsrTargetDateTime = orderExt.UsrRerouteTargetDateTime;
shipmentExt.UsrPromiseDateTime = orderExt.UsrReroutePromiseDateTime;
}
}
}
}
}
}
}
return baseMethod(order, shipment, siteID, shipDate, operation, orderOperation, newlyCreated);
}
#endregion
The only scenario where I would expect this field not to be copied is when a shipment includes multiple orders, where Acumatica's core logic sets the field to null. However, in our case, each shipment has only one order assigned to it.
To add to the confusion, another strange occurrence is that we’ve observed cases where the CustomerOrderNbr
was initially copied over as expected, but when inspecting it later, the value has gone to null.
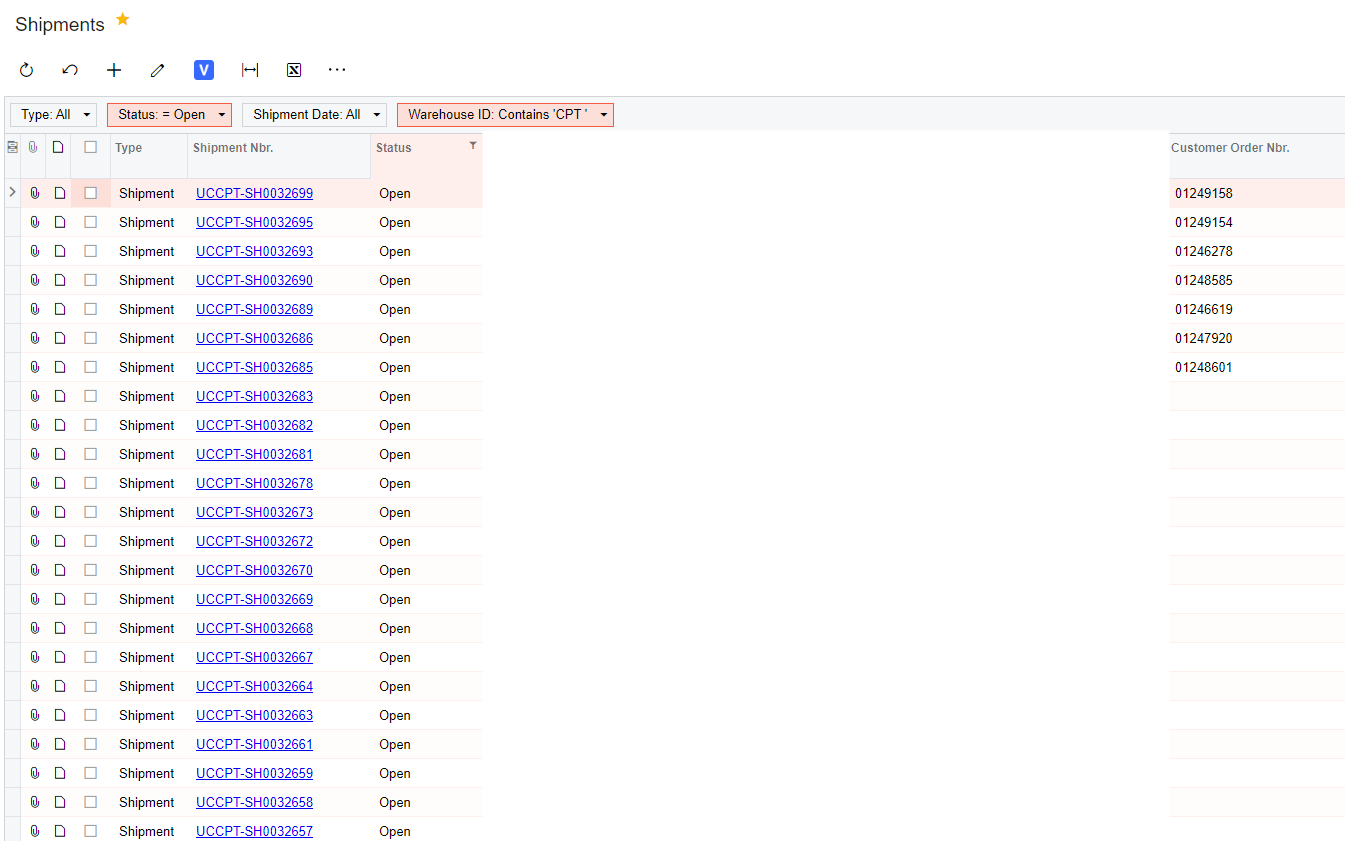
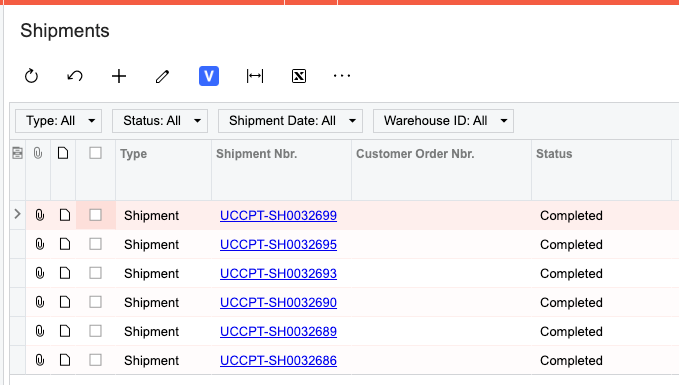
What we’ve observed:
- The issue did not occur before upgrading to Acumatica 2024 R1 (although we did jump a major version, we skipped 2023R2).
- No error messages or logs seem to provide any clues as to why this field is not always copied.
Has anyone else encountered a similar issue after upgrading to 2024 R1? Are there any known changes in the core code that could have impacted this functionality? Any suggestions or insights would be greatly appreciated.
Thank you in advance!