On the Process Order page.
I have created an action “Create Blanket Lines”
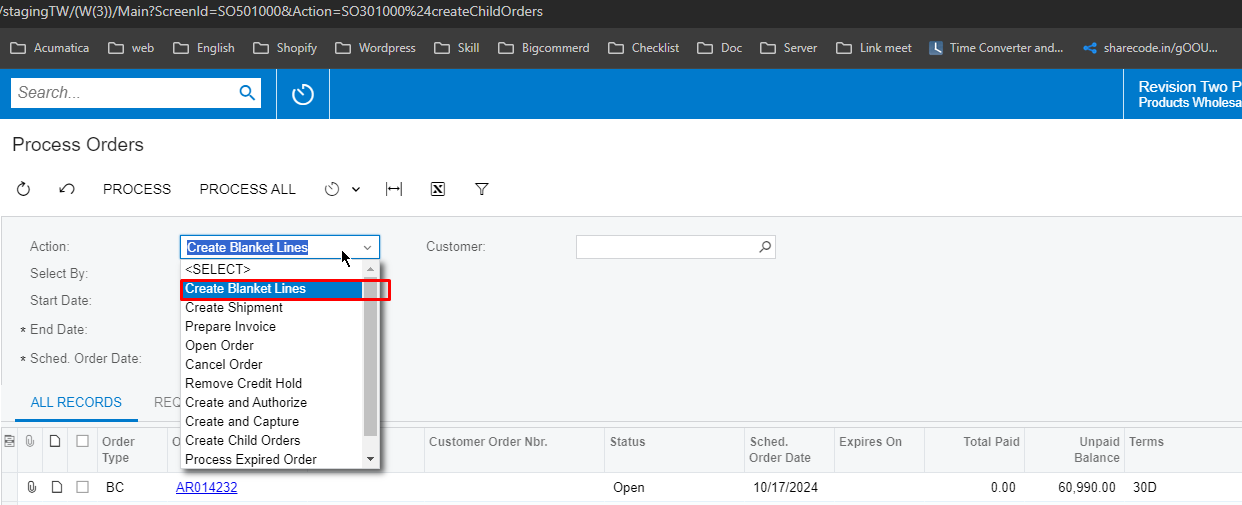
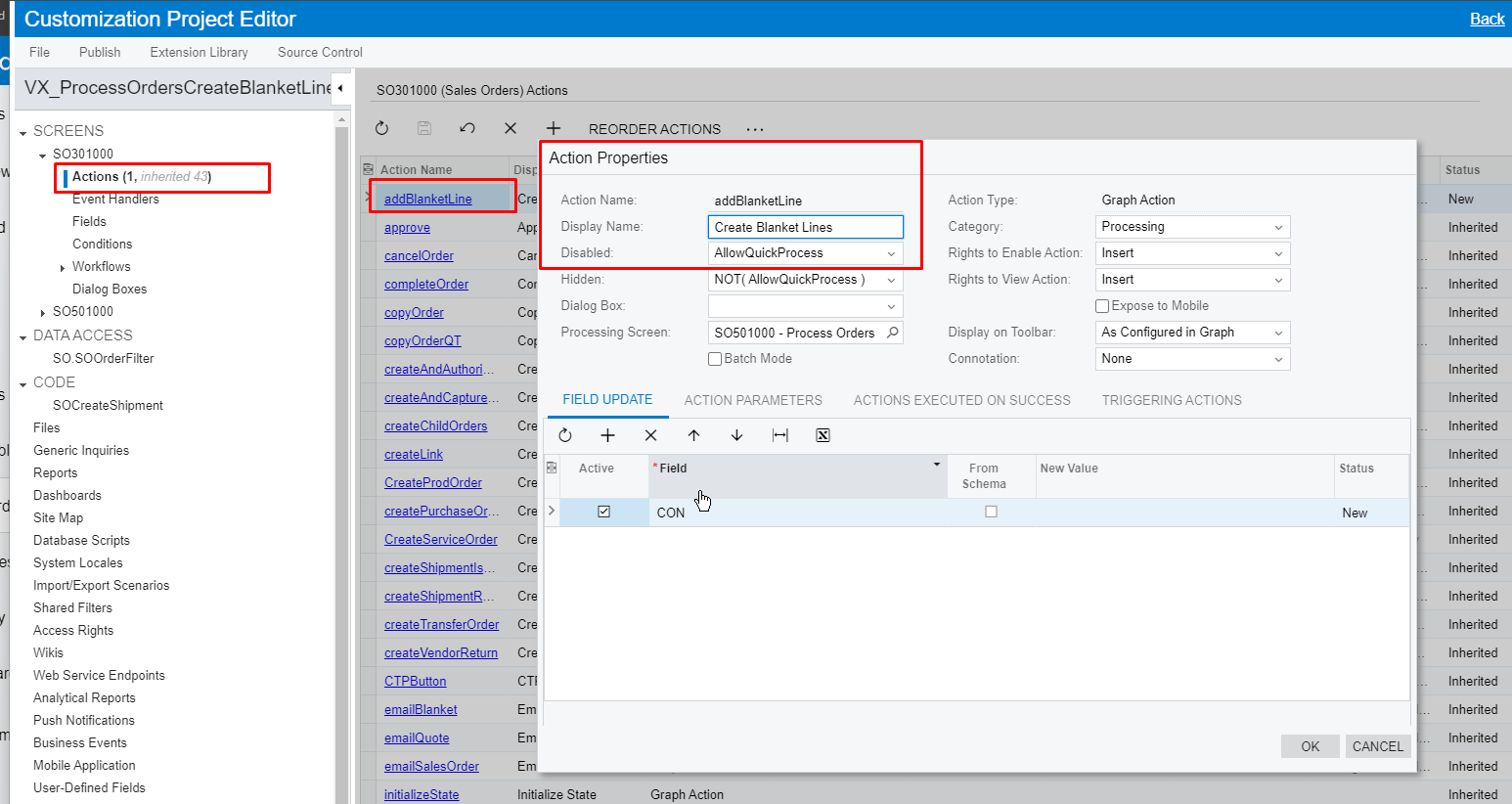
Here is my code
using System;
using System.Collections;
using System.Collections.Generic;
using PX.Data;
using PX.Objects.CN.Subcontracts.AP.CacheExtensions;
using PX.Objects.SO;
using static PX.Web.Customization.RowFilesTree;
public class SOCreateShipment_Extension : PXGraphExtension<PX.Objects.SO.SOCreateShipment>
{
protected void ProcessCustomization()
{
PXTrace.WriteInformation("open 1");
SOOrderFilter filter = Base.Filter.Current as SOOrderFilter;
if (filter != null && filter.Action == "SO301000$addBlanketLine")
{
foreach (SOOrder order in Base.Caches[typeof(SOOrder)].Updated)
{
if (order.Selected == true)
{
// My customization code
}
}
}
}
}
The problem I'm having is:
I want when I select the action “Create Blanket Lines’ and press the Process button, it will run the ProcessCustomization code( without affecting other actions ). Because I want to be able to use Processing Dialog Box to control whether my code runs correctly.
I haven't done that yet.
I have tried using the following ways:
public PXAction<SOOrderFilter> Process;
[PXButton]
[PXUIField(DisplayName = "Process")]
protected IEnumerable process(PXAdapter adapter) {
ProcessCustomization();
}
or
use protected void SOOrderFilter_RowSelected(PXCache cache, PXRowSelectedEventArgs e)
However, it is not correct, it all affects when I click process for another action.
Please help me regarding this issue (I select the action “Create Blanket Lines’ and press the Process button, it will run the ProcessCustomization code( without affecting other actions )).