Hello everyone,
I'm facing an issue with a custom Acumatica screen that involves managing customer records (SanitationCustomer
). I have a custom graph (SanitationCustomerMaint
) and DAC (SanitationCustomer
) that allow users to select a different ClientID
using a selector. The main problem occurs when switching between tabs and updating the ClientID
.
Here is the specific issue:
-
When I update the
ClientID
from the General tab, the customer information is correctly updated for fields such as name, address, city, etc., and all the related views are refreshed accordingly. -
However, when I switch to a different tab (e.g., Brewers or Invoices) and change the
ClientID
, the newClientID
appears to be set, but when I navigate back to the
General tab, the customer information shown is still from the previousClientID
.
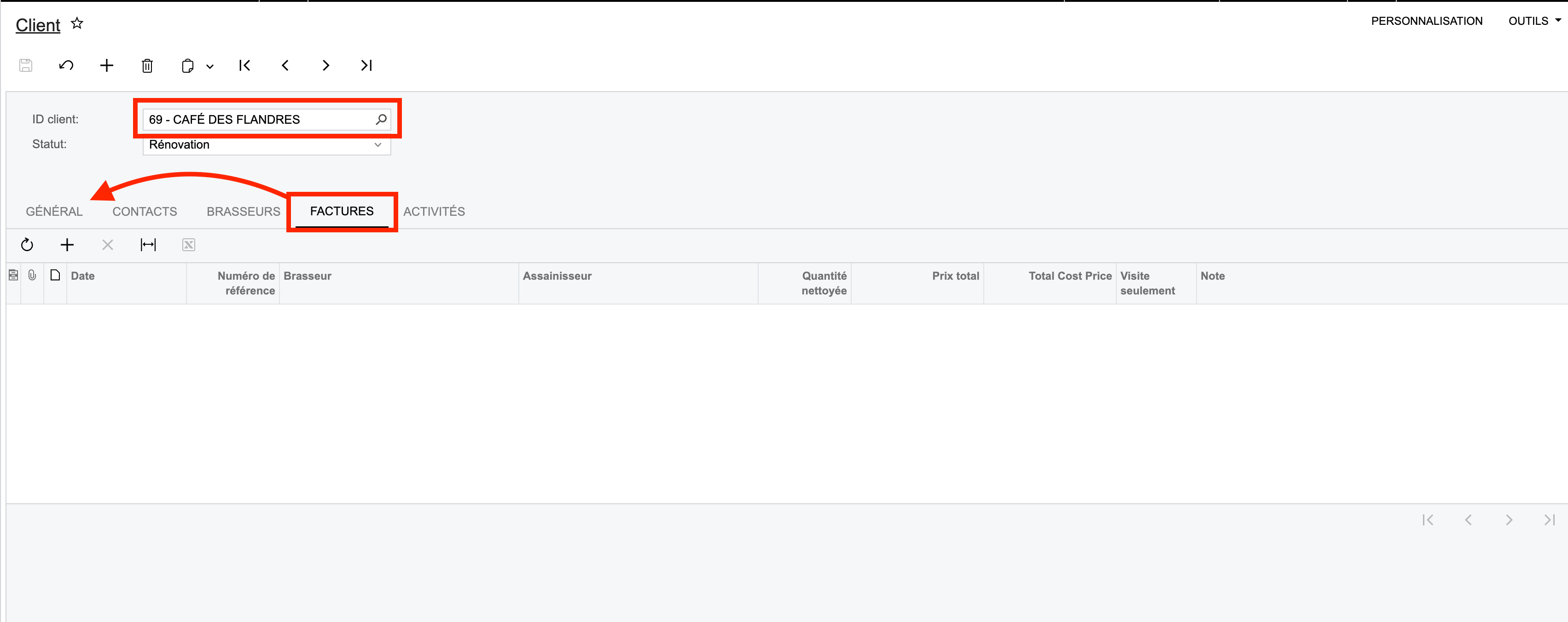
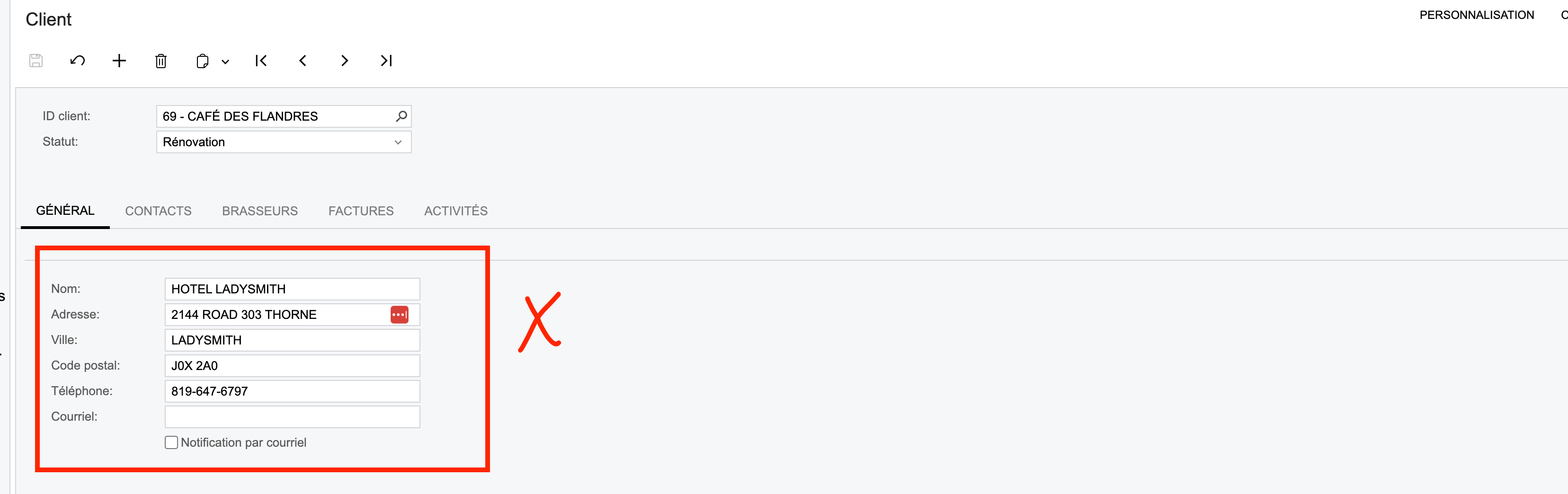
Additionally, if I save a new record to a related view (e.g., AssignedBreweries), it gets associated with the previous ClientID
instead of the newly selected one.
using System;
using PX.Data;
using PX.Data.BQL.Fluent;
using PX.Data.BQL;
using PX.Web.UI;
namespace Assainissement
{
public class SanitationCustomerMaint : PXGraph<SanitationCustomerMaint, SanitationCustomer>
{
#region Views
public PXSelect<SanitationCustomer> Customers;
public SelectFrom<ClientAvailability>
.Where<ClientAvailability.clientID.IsEqual<SanitationCustomer.clientID.FromCurrent>>.View ClientAvailabilities;
public SelectFrom<SanitationContact>
.Where<SanitationContact.clientID.IsEqual<SanitationCustomer.clientID.FromCurrent>>.View Contacts;
public SelectFrom<SanitationActivity>
.Where<SanitationActivity.clientID.IsEqual<SanitationCustomer.clientID.FromCurrent>>.View Activities;
public SelectFrom<ClientBrewery>
.InnerJoin<Brewery>.On<ClientBrewery.breweryID.IsEqual<Brewery.breweryID>>
.Where<ClientBrewery.clientID.IsEqual<SanitationCustomer.clientID.FromCurrent>>.View AssignedBreweries;
public SelectFrom<BreweryBeerLine>
.InnerJoin<Beer>.On<BreweryBeerLine.beerID.IsEqual<Beer.beerID>>
.Where<BreweryBeerLine.clientID.IsEqual<SanitationCustomer.clientID.FromCurrent>
.And<Beer.breweryID.IsEqual<SanitationCustomer.breweryID.FromCurrent>>>.View AssignedBeers;
public SelectFrom<SanitizerBillingReport>
.Where<SanitizerBillingReport.clientID.IsEqual<SanitationCustomer.clientID.FromCurrent>>
.OrderBy<SanitizerBillingReport.date.Desc>.View Invoices;
#endregion
#region Events
// Assurez-vous que le ClientID est renseigné à l'insertion dans les tables dépendantes
protected void _(Events.RowInserted<ClientAvailability> e)
{
if (e.Row != null && Customers.Current != null)
{
e.Row.ClientID = Customers.Current.ClientID;
}
}
protected void _(Events.RowInserted<SanitationActivity> e)
{
if (e.Row != null && Customers.Current != null)
{
e.Row.ClientID = Customers.Current.ClientID;
}
}
protected void _(Events.RowInserted<SanitationContact> e)
{
if (e.Row != null && Customers.Current != null)
{
e.Row.ClientID = Customers.Current.ClientID;
}
}
protected void _(Events.RowInserted<SanitizerBillingReport> e)
{
if (e.Row != null && Customers.Current != null)
{
e.Row.ClientID = Customers.Current.ClientID;
// Récupérer le prix basé sur la quantité nettoyée
PriceGridTable priceGrid = SelectFrom<PriceGridTable>
.Where<PriceGridTable.breweryID.IsEqual<@P.AsInt>
.And<PriceGridTable.minQuantity.IsLessEqual<@P.AsInt>>
.And<PriceGridTable.maxQuantity.IsGreaterEqual<@P.AsInt>
.Or<PriceGridTable.maxQuantity.IsNull>>>
.OrderBy<Asc<PriceGridTable.minQuantity>>
.View.Select(this, e.Row.BreweryID, e.Row.TotalCleaned, e.Row.TotalCleaned).TopFirst;
if (priceGrid != null)
{
e.Row.TotalPrice = priceGrid.PricePerUnit * e.Row.TotalCleaned;
}
else
{
e.Row.TotalPrice = 0;
}
}
}
protected void _(Events.RowInserted<ClientBrewery> e)
{
if (e.Row != null && Customers.Current != null)
{
e.Row.ClientID = Customers.Current.ClientID;
}
}
protected void _(Events.RowInserted<BreweryBeerLine> e)
{
if (e.Row != null && Customers.Current != null)
{
e.Row.ClientID = Customers.Current.ClientID;
}
}
// Événement déclenché lorsqu'un champ est mis à jour dans SanitationCustomer
protected virtual void _(Events.FieldUpdated<SanitationCustomer, SanitationCustomer.breweryID> e)
{
if (e.Row != null)
{
AssignedBeers.View.RequestRefresh(); // Rafraîchir la vue des bières assignées
}
}
protected virtual void _(Events.FieldUpdated<SanitationCustomer, SanitationCustomer.clientID> e)
{
if (e.Row != null)
{
Customers.View.RequestRefresh(); // Rafraîchir la vue principale pour synchroniser avec le client sélectionné
}
}
// Événement déclenché lorsque le champ TotalCleaned est mis à jour dans SanitizerBillingReport
protected void _(Events.FieldUpdated<SanitizerBillingReport, SanitizerBillingReport.totalCleaned> e)
{
SanitizerBillingReport row = e.Row;
if (row == null) return;
// Récupérer le prix basé sur la quantité nettoyée
PriceGridTable priceGrid = SelectFrom<PriceGridTable>
.Where<PriceGridTable.breweryID.IsEqual<@P.AsInt>
.And<PriceGridTable.minQuantity.IsLessEqual<@P.AsInt>>
.And<PriceGridTable.maxQuantity.IsGreaterEqual<@P.AsInt>
.Or<PriceGridTable.maxQuantity.IsNull>>>
.OrderBy<Asc<PriceGridTable.minQuantity>>
.View.Select(this, row.BreweryID, row.TotalCleaned, row.TotalCleaned).TopFirst;
if (priceGrid != null)
{
row.TotalPrice = priceGrid.PricePerUnit * row.TotalCleaned;
}
else
{
row.TotalPrice = 0;
}
}
#endregion
#region Actions
public PXAction<SanitationCustomer> TraceBreweryID;
[PXButton(CommitChanges = true)]
[PXUIField(DisplayName = "Afficher les bières")]
protected void traceBreweryID()
{
var currentBrewery = AssignedBreweries.Current;
if (currentBrewery != null)
{
Customers.Current.BreweryID = currentBrewery.BreweryID;
Customers.Cache.Update(Customers.Current);
AssignedBeers.View.RequestRefresh();
}
}
#endregion
}
}