In Production Details screen’s extension, I have added an Action called ConnectToMRN. In it’s workflow extension I have defined a dialog box also. I customized the Action such that it pop up the dialog box on click the button using the customization editor as bellow.
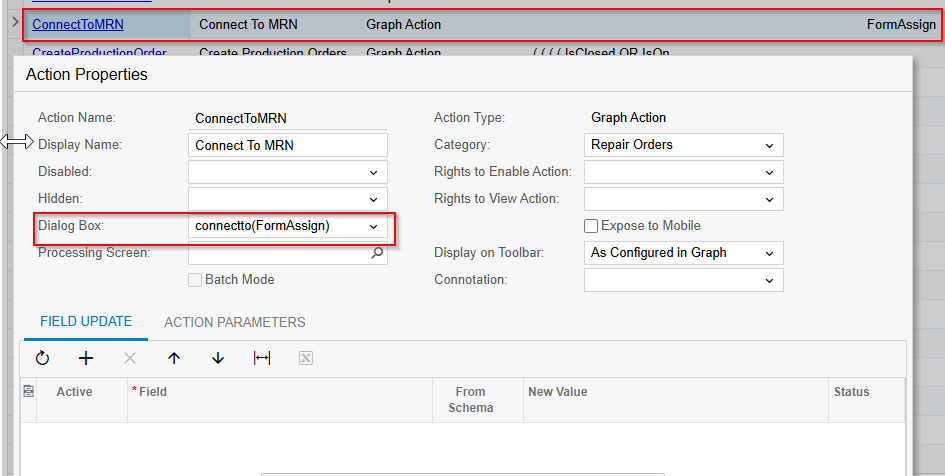
Following is complete workflow customization code of the production details screen.
public class ProductionOrderWorkflow : PXGraphExtension<ProdDetail_Workflow, ProdDetail>
{
public sealed override void Configure(PXScreenConfiguration config)
{
Configure(config.GetScreenConfigurationContext<ProdDetail, AMProdItem>());
}
protected static void Configure(WorkflowContext<ProdDetail, AMProdItem> context)
{
var formAssign = context.Forms.Create("FormAssign", form =>
form.Prompt("connectto").WithFields(fields =>
{
fields.Add("ConnectTo", field => field
.WithSchemaOf<MRMaterialRequest.refNbr>()
.IsRequired()
.Prompt("connectto"));
}));
var repairCategory = context.Categories.CreateNew(
ActionCategories.RepairCategoryID,
category => category.DisplayName(ActionCategories.DisplayNames.RepairOrders));
var viewOrder = context.ActionDefinitions
.CreateExisting<ProdDetail_Extension_1>(g => g.ConnectToMRN, a => a.WithCategory(repairCategory));
context.UpdateScreenConfigurationFor(screen => screen
.UpdateDefaultFlow(flow =>
{
return flow
.WithFlowStates(flowStates =>
{
flowStates.Update<ProductionOrderStatus.closed>(flowState =>
{
return flowState.WithActions(actions =>
actions.Add(viewOrder)
);
});
flowStates.Update<ProductionOrderStatus.completed>(flowState =>
{
return flowState.WithActions(actions =>
actions.Add(viewOrder)
);
});
flowStates.Update<ProductionOrderStatus.cancel>(flowState =>
{
return flowState.WithActions(actions =>
actions.Add(viewOrder)
);
});
});
})
.WithCategories(categories =>
{
categories.Add(repairCategory);
})
.WithActions(actions =>
{
actions.Add(viewOrder);
})
.WithForms(forms => forms.Add(formAssign))
);
}
following is my action code. It just contains the template without exact logic.
public PXSelect<MRMaterialRequest> MRMaterialRequestPopupView;
public PXAction<AMProdItem> ConnectToMRN;
[PXButton(CommitChanges = true)]
[PXUIField(DisplayName = "Connect To MRN", MapEnableRights = PXCacheRights.Select, MapViewRights = PXCacheRights.Select)]
protected virtual IEnumerable connectToMRN(PXAdapter adapter)
{
// Show the popup to select MRMaterialRequest
return adapter.Get();
}
- How can I access the value selected from the dialog box from my action?
- how to configure the action with Dialog box via the customization code? Without using the customization editor.
- is there any other way to implement above requirement without dealing with workflows?