Hi I have done a customization where I set the required qty to 1.00 in the material wizard 2 screen which works, I now need to make sure the release qty field also reflects this change where release qty needs to be the 1.00*the BOM, it still seems to be the original qty * the BOM with the logic I have written below.
using System;
using PX.Data;
using System.Collections.Generic;
using System.Collections;
using PX.Objects.Common.Extensions;
using PX.Objects;
using PX.Objects.AM;
namespace PX.Objects.AM
{
public class MatlWizard2_Extension : PXGraphExtension<PX.Objects.AM.MatlWizard2>
{
#region Event Handlers
// Use RowSelected to update QtyReq when the row is selected or edited
protected void AMWrkMatl_RowSelected(PXCache sender, PXRowSelectedEventArgs e)
{
var row = (AMWrkMatl)e.Row;
if (row == null) return;
// Force QtyReq to 1.00 whenever a row is selected or edited
row.QtyReq = 1.00m;
sender.SetValue<AMWrkMatl.qtyReq>(row, 1.00m);
// Optional: add debugging info here for verification
PXTrace.WriteInformation("AMWrkMatl_RowSelected: Setting QtyReq to 1.00");
// Perform the MatlQty calculation
if (row.QtyReq != null && row.BaseQtyReq != null)
{
// Check if it's a byproduct, if so, reverse the sign
var qty = Math.Abs(row.QtyReq.GetValueOrDefault()) * (row.IsByproduct.GetValueOrDefault() ? -1 : 1);
var baseQty = Math.Abs(row.BaseQtyReq.GetValueOrDefault()) * (row.IsByproduct.GetValueOrDefault() ? -1 : 1);
// Set MatlQty based on QtyReq and BaseQtyReq (similar to original code logic)
row.MatlQty = qty * baseQty;
sender.SetValue<AMWrkMatl.matlQty>(row, row.MatlQty);
PXTrace.WriteInformation($"Calculated MatlQty: {row.MatlQty}");
}
}
#endregion
}
}
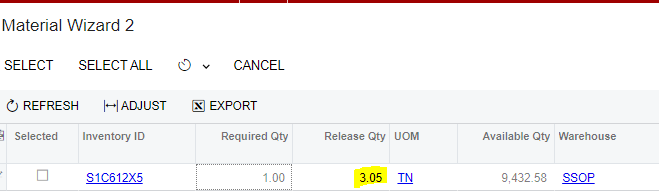
Any help would be appreciated, thank you!