I’m trying to default Use Customer Account to true or false based on the Shipping Terms via the Fields tab in the Customization Project. I’m using the code “=IIf([ShipTermsID] = 'PPA', False, True)” however, this doesn’t work. How can I get this to work.
Trying to Default a Value using Customization Project?
Best answer by harutyungevorgyan
Hi
If you already have a project you want to add this code to, open it. Otherwise, create a new one. Within your customization project, click the Code button (typically found in the left panel). Click Add Source Code → Add Graph Extension
In the lookup, search for “SOShipmentEntry” .
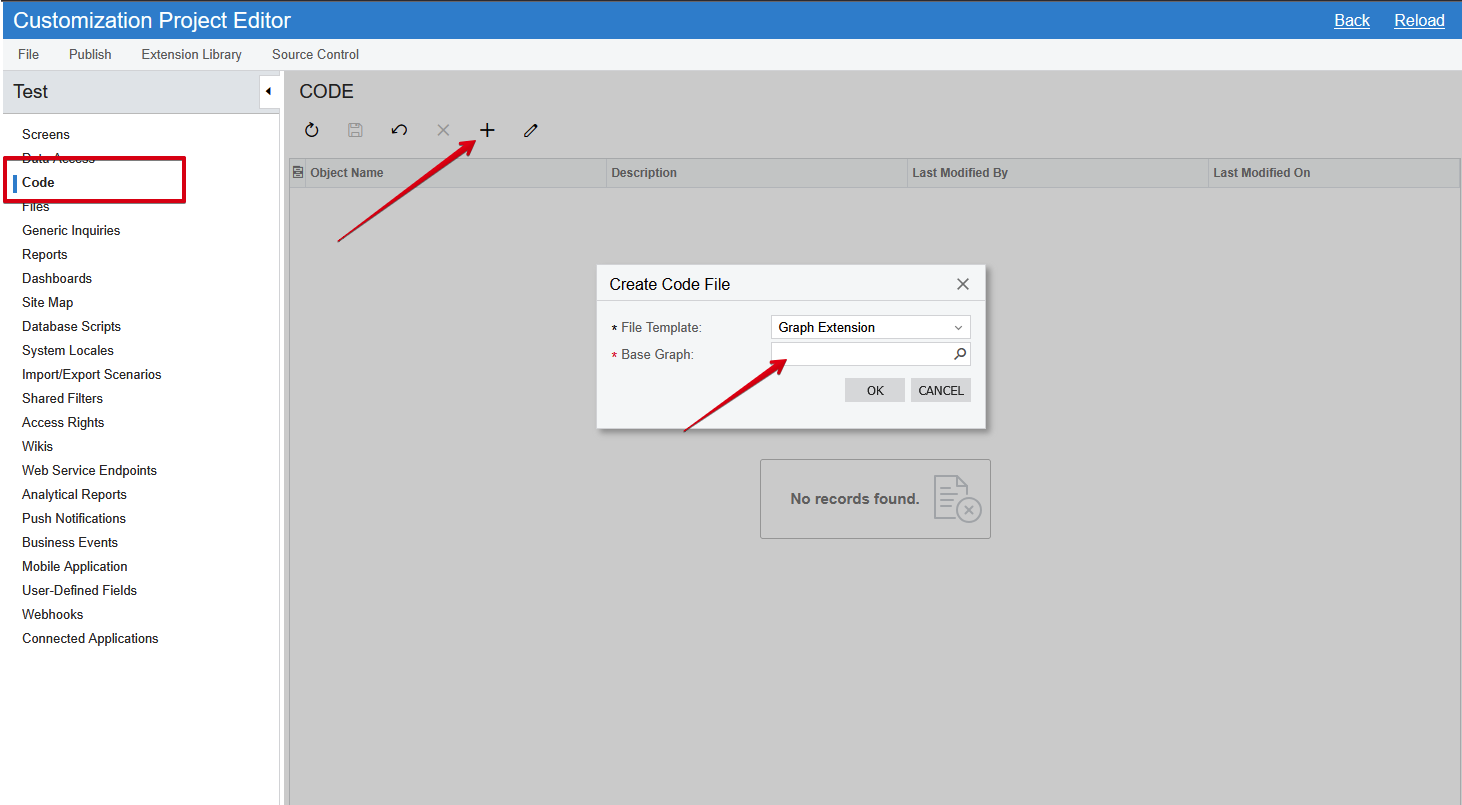
Once you’ve created the extension file, you’ll see a code editor. Paste in the event handler that
namespace PX.Objects.SO
{
public class SOShipmentEntry_Extension : PXGraphExtension<PX.Objects.SO.SOShipmentEntry>
{
#region Event Handlers
public virtual void _(Events.FieldUpdated<SOShipment.shipTermsID> e)
{
if (e.Row == null)
return;
e.Cache.SetValueExt<SOShipment.useCustomerAccount>(e.Row, e.NewValue != "PPA");
}
#endregion
}
}
After pasting your code, click Save, then publish your changes.
When it finishes, go back to the SO302000 screen (Shipments) and test by updating the Ship Terms field. Acumatica should then automatically set the Use Customer Account checkbox based on whether you chose “PPA” or not.
That worked perfectly
e.Cache.SetValueExt<SOShipment.useCustomerAccount>(e.Row, e.NewValue != "PPA")
And change a bit, but how would I invert this?
You already have e.NewValue != "PPA" and this part is totally simple, you say that e.NewValue (which is an updated value) is not "PPA". If you have complex conditions, you can ask, and I will give you the right code, but mostly, what you need to do is to use && as (and) and || as (or) and write your condition right after PPA so for example, e.NewValue != "PPA" || e.NewValue != "AAP").
Makes sense. If I wanted to do a logic loop for two shipping vias that check the Use Customers Account and two that unchecked the box, would I just do e.NewValue != "PPA" || e.NewValue != "AAP" || e.NewValue == "PA" || e.NewValue == "AP"? I tried doing this and it just defaulted to checking the box no matter what.
Thank you for your continued interest!
At this point, we’re diving into foundational principles of the C# language itself—particularly around boolean logic and condition evaluation. This can get quite deep for what should be a simple question-answer in Acumatica Community.
For your case, the cleanest and most readable way to implement the logic is by using a list and checking inclusion like this:
// Define the list of types for which the checkbox should be checked
string[] acceptedTypes = new string[] { "PA", "AP" };
// Check if the new value is in the accepted types list
// Make sure to include 'using System.Linq;' at the top of your file, as it's required for the Contains() method to work
bool useCustomerAccount = acceptedTypes.Contains(e.NewValue);
// Set the checkbox value based on the result
e.Cache.SetValueExt<SOShipment.useCustomerAccount>(e.Row, useCustomerAccount);
// If you want to check the checkbox when the value is NOT in the list, use:
// e.Cache.SetValueExt<SOShipment.useCustomerAccount>(e.Row, !useCustomerAccount);
You need to paste this code instead of e.Cache.SetValueExt<SOShipment.useCustomerAccount>(e.Row, e.NewValue != “PPA”); line in your code now.
If this approach is unclear or feels unfamiliar, I’d kindly suggest investing some time into C# basics. You don’t need to master the entire language right away, but knowing how conditionals, arrays, and events work will go a long way in making your Acumatica customizations more reliable and maintainable.
Happy coding! 👨💻
Reply
Enter your E-mail address. We'll send you an e-mail with instructions to reset your password.