I have two custom fields Absolute Variance Quantity for rows and Total Absolute Variance Quantity in the header. I can get these fields to update via PI Review and Scan and Count just fine, but when I do an action such as Set Not Entered To Zero, the custom header field won’t update.
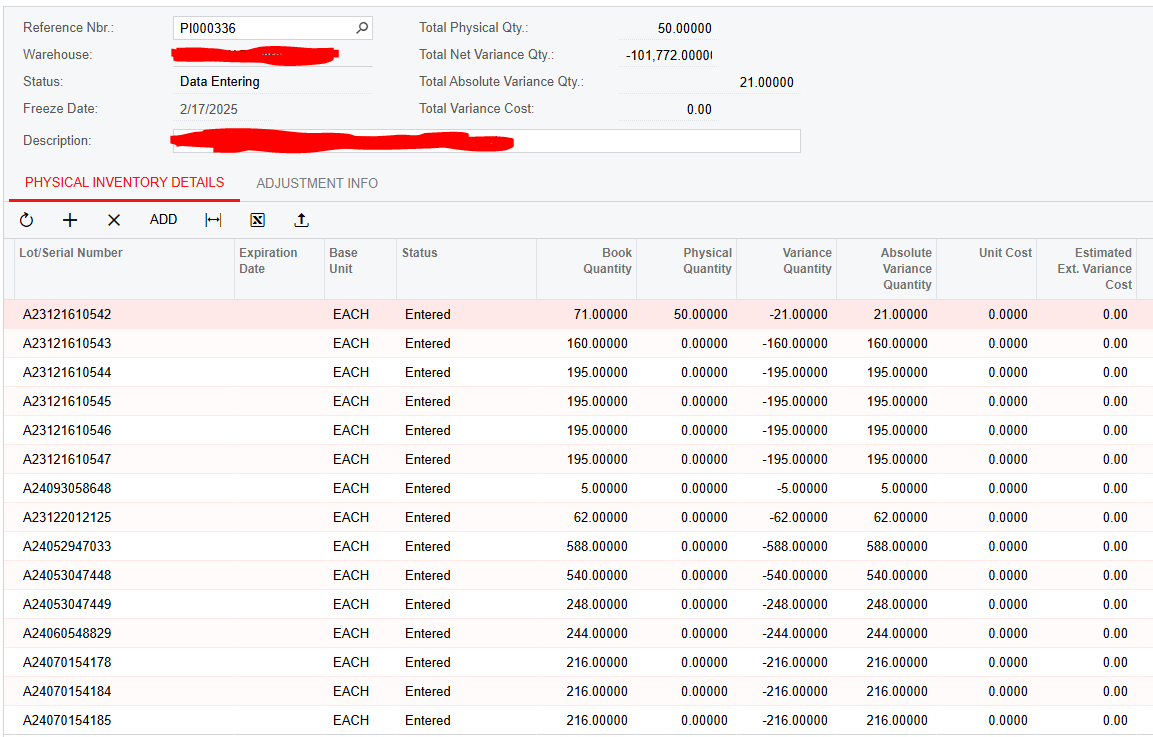
Here is my code for the field definitions and this screen:
INPIHeaderExt.usrTotalAbsVarQty
[PXDBQuantity]
[PXDefault(TypeCode.Decimal, "0.00")]
[PXUIField(DisplayName = "Total Absolute Variance Qty.", Visibility = PXUIVisibility.SelectorVisible, Enabled = false)]
INPIDetailExt.usrAbsVarQty
[PXDBQuantity()]
[PXUIField(DisplayName="Absolute Variance Quantity", Enabled = false)]
[PXFormula(null, typeof(SumCalc<INPIHeaderExt.usrTotalAbsVarQty>) )]
Code Editor: INPIReview (Physical Inventory Review)
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using PX.Common;
using PX.Data;
using PX.Data.BQL;
using PX.Data.BQL.Fluent;
using PX.Objects.CM;
using PX.Objects.Common.Extensions;
using PX.Objects.CS;
using PX.Objects.IN.PhysicalInventory;
using PX.Data.WorkflowAPI;
using PX.Objects;
using PX.Objects.IN;
namespace PX.Objects.IN
{
//this is for the PI Review screen, where data can be entered manually line by line
public class INPIReview_Extension : PXGraphExtension<PX.Objects.IN.INPIReview>
{
protected void _(Events.FieldUpdated<INPIDetail, INPIDetail.physicalQty> e)
{
var row = (INPIDetail)e.Row;
if (row == null) return;
//INPIDetail details = (INPIDetail)Base.Tools.Current;
//var inpiDetail = PXCache<INPIDetail>.GetExtension<INPIDetailExt>(details);
//INPIDetailExt inpiDetailExtVar = Base.Document.Current.GetExtension<INPIDetailExt>();
decimal varianceQuantityVariable = (decimal)0.00;
if(row.PhysicalQty != null && row.BookQty != null){
varianceQuantityVariable = (decimal)(row.VarQty);//how variance quantity is initially calculated
}
decimal absoluteVarianceQuantity = Math.Abs(varianceQuantityVariable);
e.Cache.SetValueExt<INPIDetailExt.usrAbsVarQty>(row, absoluteVarianceQuantity);
//save this changed cache to the form
Base.Actions.PressSave();
//Refresh the header
Base.PIHeader.View.RequestRefresh();
return;
}
}
}
I’ve tried changing the field in the event handler to INPIDetail.varQty but it resulted in the custom detail field not updating at all. Changing the event handler to RowUpdated also didn’t work, and it only changed the header field after the next row got changed, so it was always one row behind.