I have been trying to send a token via API Key Authorization method but I've been getting an unauthorized error:
{StatusCode: 401, ReasonPhrase: 'Unauthorized', Version: 1.1, Content: System.Net.Http.NoWriteNoSeekStreamContent, Headers:
{
Date: Wed, 23 Oct 2024 16:35:03 GMT
Transfer-Encoding: chunked
Server: Microsoft-IIS/10.0
Content-Type: application/json; charset=utf-8
}}
This is my code:
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using PX.Common;
using PX.Data;
using PX.Data.BQL;
using PX.Data.BQL.Fluent;
using PX.Objects.CM;
using PX.Objects.CS;
using PX.Objects.GL;
using PX.Objects.Common.Extensions;
using PX.Objects;
using PX.Objects.IN;
using RiverlandDesarrolloV231;
using System.Net.Http;
using System.Threading.Tasks;
using Newtonsoft.Json;
using PX.Concurrency;
namespace PX.Objects.IN
{
public class INReceiptEntry_Extension : PXGraphExtension<PX.Objects.IN.INReceiptEntry>
{
#region Event Handlers
public PXAction<INRegister> PrenderAntena;
[PXButton]
[PXUIField(DisplayName = "Prender Antena", MapEnableRights = PXCacheRights.Select, MapViewRights = PXCacheRights.Select, Enabled = true)]
protected virtual IEnumerable prenderAntena(PXAdapter adapter)
{
this.Base.LongOperationManager.StartAsyncOperation((ct) => Prueba());
return adapter.Get();
}
public static async Task Prueba()
{
var credentials = new Credentials
{
usuario = new FieldValue { value = "MyUser" },
contraseña = new FieldValue { value = "MyPassword" }
};
string jsonPayload = JsonConvert.SerializeObject(credentials, Formatting.Indented);
string url = "MyUrl";
var handler = new WinHttpHandler();
using (HttpClient client = new HttpClient(handler))
{
try
{
var requestMessage = new HttpRequestMessage(HttpMethod.Get, url);
requestMessage.Content = new StringContent(jsonPayload, Encoding.UTF8, "application/json");
HttpResponseMessage response = await client.SendAsync(requestMessage);
PXTrace.WriteInformation("Estado de la respuesta: " + response.StatusCode.ToString());
if (response.IsSuccessStatusCode)
{
string responseContent = await response.Content.ReadAsStringAsync();
PXTrace.WriteInformation("Respuesta del servidor: " + responseContent.ToString());
var apiResponse = JsonConvert.DeserializeObject<ApiResponse>(responseContent);
PXTrace.WriteInformation("API key: " + apiResponse.apiKey?.value.ToString());
var receiptNbr = new ReceiptNbr
{
numeroSolicitud = new FieldValue { value = "0987654" }
};
string jsonPayload2 = JsonConvert.SerializeObject(receiptNbr, Formatting.Indented);
string url2 = "MyUrl";
var handler2 = new WinHttpHandler();
using (HttpClient client2 = new HttpClient(handler2))
{
try
{
var requestMessage2 = new HttpRequestMessage(HttpMethod.Post, url2);
requestMessage2.Content = new StringContent(jsonPayload2, Encoding.UTF8, "application/json");
client2.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Api-Key", apiResponse.apiKey?.value);
HttpResponseMessage response2 = await client.SendAsync(requestMessage2);
PXTrace.WriteInformation("Estado de la respuesta: " + response2.StatusCode.ToString());
if (response2.IsSuccessStatusCode)
{
string responseContent2 = await response2.Content.ReadAsStringAsync();
PXTrace.WriteInformation("Respuesta del servidor: " + responseContent2.ToString());
var apiResponse2 = JsonConvert.DeserializeObject<ApiResponse>(responseContent2);
PXTrace.WriteInformation("API key: " + apiResponse2.apiKey?.value.ToString());
}
}
catch (HttpRequestException httpEx2)
{
PXTrace.WriteInformation("Excepción en la solicitud HTTP : " + httpEx2.Message);
}
catch (Exception ex2)
{
PXTrace.WriteInformation("Excepción en la solicitud : " + ex2.Message);
}
}
}
else
{
string errorResponse = await response.Content.ReadAsStringAsync();
PXTrace.WriteInformation("Error en la solicitud: " + response.ReasonPhrase.ToString(), "Contenido: " + errorResponse);
}
}
catch (HttpRequestException httpEx)
{
PXTrace.WriteInformation("Excepción en la solicitud HTTP : " + httpEx.Message);
}
catch (Exception ex)
{
PXTrace.WriteInformation("Excepción en la solicitud : " + ex.Message);
}
}
}
public class ApiResponse
{
public ApiKey apiKey { get; set; }
}
public class ApiKey
{
public string value { get; set; }
}
public class Credentials
{
public FieldValue usuario { get; set; }
public FieldValue contraseña { get; set; }
}
public class FieldValue
{
public string value { get; set; }
}
public class ReceiptNbr
{
public FieldValue numeroSolicitud { get; set; }
}
#endregion
}
}
I added this line of code but it may not be working properly:
client2.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Api-Key", apiResponse.apiKey?.value);
All this works well in Postman
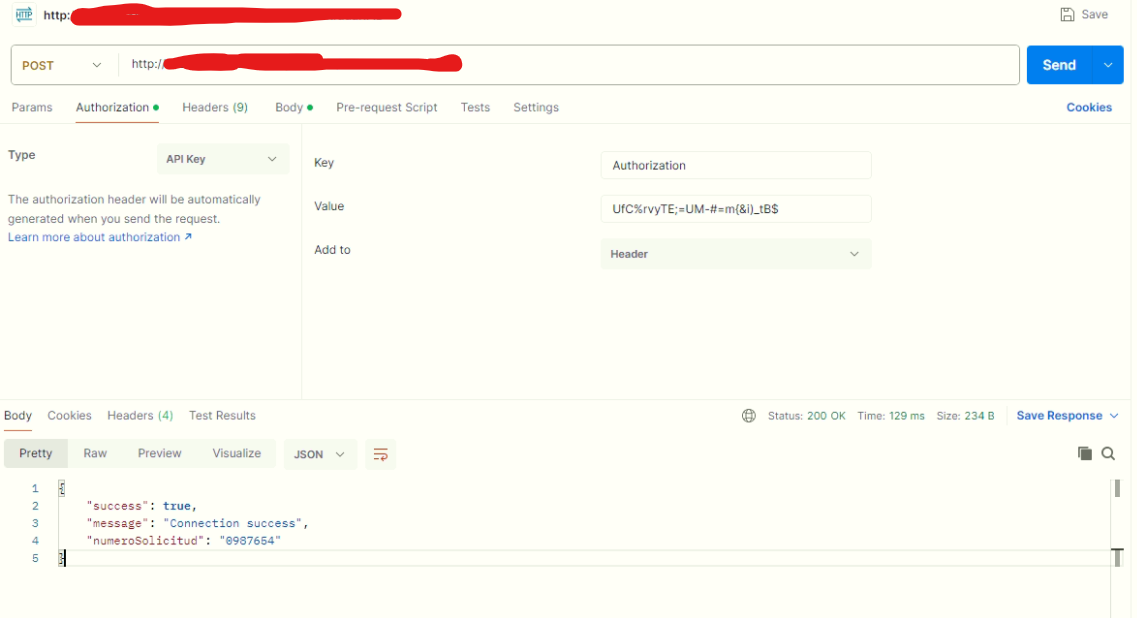
Can anyone help me to resolve this issue?