I am trying to create the move transaction (AM302000) automatically when the release button is clicked in the materials screen (AM300000)
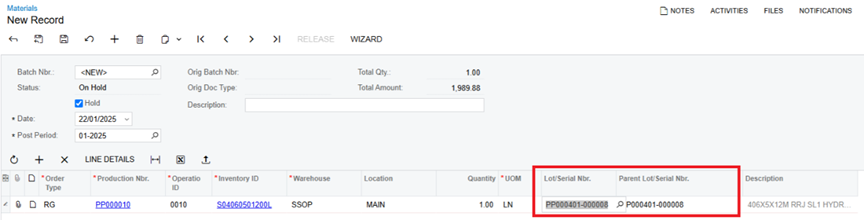
On release of this materials transaction the development will create and release the Move
Transaction with the below populated from the Materials Screen
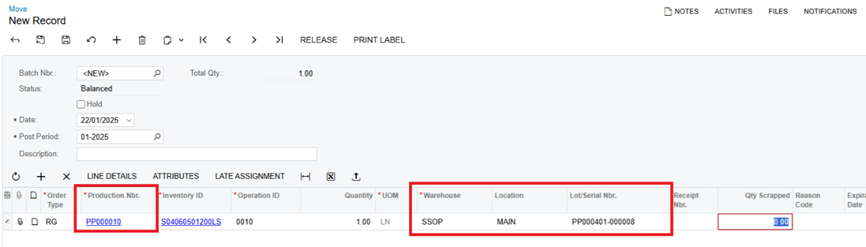
This is the override of the release button I have attempted below but it is not creating the new move transaction but there are no validation errors.
#region Release Override
public delegate IEnumerable ReleaseDelegate(PXAdapter adapter);
[PXOverride]
public IEnumerable Release(PXAdapter adapter, ReleaseDelegate baseMethod)
{
// First, execute the base release method to ensure original functionality
var documents = baseMethod(adapter).Cast<AMBatch>().ToList();
// Process move transactions after base release
foreach (AMBatch batch in documents)
{
if (batch.Status == "R") // Check if the batch is Released
{
CreateMoveTransactions(batch);
}
}
return documents;
}
#endregion
#region Private Methods
private void CreateMoveTransactions(AMBatch batch)
{
// Create a new graph for Move Entry
var moveGraph = PXGraph.CreateInstance<MoveEntry>();
// Get all material transactions for this batch
var materialTrans = SelectFrom<AMMTran>
.Where<AMMTran.batNbr.IsEqual<@P.AsString>>
.View.Select(Base, batch.BatNbr)
.RowCast<AMMTran>();
foreach (AMMTran mtran in materialTrans)
{
// Create the Move transaction
var moveTran = new AMMTran
{
ProdOrdID = mtran.ProdOrdID, // Production Nbr
SiteID = mtran.SiteID, // Warehouse
LocationID = mtran.LocationID, // Location
LotSerialNbr = mtran.ParentLotSerialNbr, // Lot/Serial Nbr
InventoryID = mtran.InventoryID,
UOM = mtran.UOM,
Qty = mtran.Qty,
TranType = "M" // Move transaction type
};
// Insert the move transaction into MoveEntry graph
moveGraph.transactions.Insert(moveTran);
}
// Save and process the move transactions
moveGraph.Actions.PressSave();
}
#endregion
Please let me know if there is anything missing, thank you!