Hello. When I create a sales order with code it is different to the sales order I create via the UI.
The order type and customer are the same so I don’t understand when the final order is different.
The sales order below has been created with the UI. I only set the Customer and Customer Order Ref fields. Notice that the Contact field has been set to ‘Book In’ automatically.
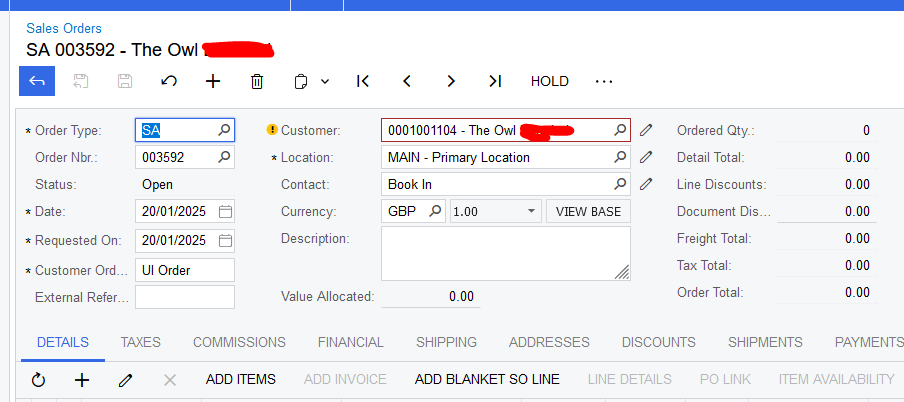
To create a sales order using code, I’ve used the following code.
SOOrderEntry sOOrderEntry = PXGraph.CreateInstance<SOOrderEntry>();
SOOrder sOOrder = (SOOrder)sOOrderEntry.Document.Cache.CreateInstance();
sOOrderEntry.Document.Cache.SetValueExt<SOOrder.orderType>(sOOrder, "SA");
sOOrderEntry.Document.Cache.SetValueExt<SOOrder.customerID>(sOOrder, 7511);
sOOrderEntry.Document.Cache.SetValueExt<SOOrder.customerOrderNbr>(sOOrder, "Test Order");
sOOrder = sOOrderEntry.Document.Insert(sOOrder);
sOOrderEntry.Actions.PressSave();
The sales order created is shown below. The Contact field is empty!
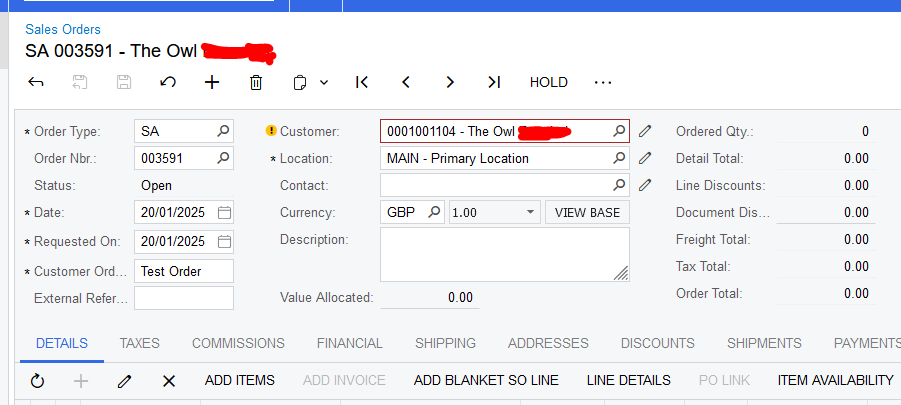
Can anyone explain why the Contact field isn’t updated?
Can anyone explain how to change the code to make the contact appear?