Hi there,
I am trying to override the address line 1 on Shipment screen by defaulting it to the User Defined Field selection (pointing to Customer table). However, when I got this error when I try to test it:
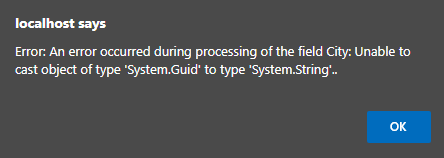
Below is my DAC as well as code for your reference:
DAC:
using System;
using PX.Data;
using PX.Data.BQL;
using PX.Objects.SO;
namespace PX.Objects.SO
{
[Serializable]
[PXCacheName("SOShipmentKvExt")]
public class SOShipmentKvExt : IBqlTable
{
public abstract class recordID : BqlGuid.Field<recordID> { }
[PXDBGuid(IsKey = true)]
public Guid? RecordID { get; set; }
public abstract class fieldName : BqlString.Field<fieldName> { }
[PXDBString(50,IsKey = true)]
[PXUIField(DisplayName ="Name")]
public string FieldName { get; set; }
public abstract class valueNumeric : BqlDecimal.Field<valueNumeric> { }
[PXDBDecimal(8)]
[PXUIField(DisplayName = "Value Numeric")]
public decimal? ValueNumeric { get; set; }
public abstract class valueDate : BqlDateTime.Field<valueDate> { }
[PXDBDate]
[PXUIField(DisplayName = "Value Date")]
public DateTime? ValueDate { get; set; }
public abstract class valueString : BqlString.Field<valueString> { }
[PXDBString(256)]
[PXUIField(DisplayName = "Value String")]
public string ValueString { get; set; }
public abstract class valueText : BqlString.Field<valueText> { }
[PXDBString]
[PXUIField(DisplayName = "Value Text")]
public string ValueText { get; set; }
}
}
Code:
using PX.CS.Contracts.Interfaces;
using PX.Data.ReferentialIntegrity.Attributes;
using PX.Data;
using PX.Objects.CR;
using PX.Objects.CS;
using PX.Objects.SO;
using PX.Objects;
using System.Collections.Generic;
using System;
namespace PX.Objects.SO
{
// Acuminator disable once PX1011 InheritanceFromPXCacheExtension [Justification]
// Acuminator disable once PX1016 ExtensionDoesNotDeclareIsActiveMethod extension should be constantly active
// Acuminator disable once PX1026 UnderscoresInDacDeclaration [Justification]
[PXNonInstantiatedExtension]
public class SO_SOAddress_ExistingColumn : PXCacheExtension<PX.Objects.SO.SOAddress>
{
#region AddressLine1
[PXMergeAttributes(Method = MergeMethod.Append)]
[PXDBScalar(typeof(Search2<SOAddress.addressLine1,
InnerJoin<SOShipment, On<SOAddress.customerID, Equal<SOShipment.customerID>>,
InnerJoin<SOShipmentKvExt, On <SOShipment.noteID, Equal<SOShipmentKvExt.recordID>>>>,
Where<SOShipment.customerID, Equal<Current<SOShipmentKvExt.valueString>>>>))]
public string AddressLine1 { get; set; }
#endregion
}
}
I suspect it is because the UDF is being treated as String while the Customer ID is treated as GUID, but I may be wrong. Any idea on how to tackle this issue?