I have created a button on the Process Order page
What I want is that when I click the Demo Processing button, the Processing dialog should be displayed. To be able to control whether the insert Order was successful or not. Function GenerateNewLineItems will work on insert item in OrderLine.
function GenerateNewLineItems.
using System;
using System.Collections;
using System.Collections.Generic;
using PX.CloudServices.DAC;
using PX.Data;
using PX.Objects.CN.Subcontracts.AP.CacheExtensions;
using PX.Objects.SO;
public class SOCreateShipment_Extension : PXGraphExtension<PX.Objects.SO.SOCreateShipment>
{
public PXAction<SOOrderFilter> DemoProcessing;
[PXButton(CommitChanges = true)]
[PXUIField(DisplayName = "Demo Processing")]
protected void demoProcessing()
{
SOOrderFilter filter = Base.Filter.Current;
if (filter == null)
{
throw new PXException("Filter cannot be null");
}
PXLongOperation.StartOperation(Base, () =>
{
try
{
foreach (SOOrder order in PXSelect<SOOrder>.Select(Base))
{
var filePath = PXCache<SOOrderFilter>.GetExtension<SOOrderFilterExt>(filter).UsrNextSchShipDate;
if (filePath != null)
{
int shipOnMonthOffset = 3;
SOOrder parentOrder = PXSelect<SOOrder, Where<SOOrder.orderNbr, Equal<Required<SOOrder.orderNbr>>>>.Select(Base, order.OrderNbr);
if (parentOrder != null)
{
GenerateNewLineItems(parentOrder, filePath, shipOnMonthOffset);
PXProcessing<SOOrderFilter>.SetProcessed();
}
else
{
PXProcessing<SOOrderFilter>.SetError(new PXException("The order has not been selected from the list"));
}
}
else
{
PXProcessing<SOOrderFilter>.SetError(new PXException("There is no Next Sch Ship Date yet"));
}
}
}
catch (Exception ex)
{
PXTrace.WriteError(ex);
throw new PXOperationCompletedException("Error in Demo Processing: " + ex.Message);
}
});
}
private void GenerateNewLineItems(SOOrder parentOrder, DateTime? nextSchShipDate, int shipOnMonthOffset)
{
using (PXTransactionScope ts = new PXTransactionScope())
{
SOOrderEntry orderEntryGraph = PXGraph.CreateInstance<SOOrderEntry>();
orderEntryGraph.Document.Current = orderEntryGraph.Document.Search<SOOrder.orderNbr>(parentOrder.OrderNbr, parentOrder.OrderType);
var duplicatedBranchesAndInventories = new HashSet<(int?, int?)>();
try
{
foreach (SOLine line in PXSelect<SOLine,
Where<SOLine.orderType, Equal<Required<SOLine.orderType>>,
And<SOLine.orderNbr, Equal<Required<SOLine.orderNbr>>>>>.Select(orderEntryGraph, parentOrder.OrderType, parentOrder.OrderNbr))
{
if (line.Completed == true)
{
try
{
var key = (line.BranchID, line.InventoryID);
if (!duplicatedBranchesAndInventories.Contains(key))
{
SOLine newLine = new SOLine
{
// My CODE
};
orderEntryGraph.Transactions.Cache.Insert(newLine);
duplicatedBranchesAndInventories.Add(key);
}
}
catch (Exception ex)
{
PXProcessing<SOLine>.SetError(new PXException($"Error inserting new line for order {parentOrder.OrderNbr}: {ex.Message}"));
}
}
}
orderEntryGraph.Save.Press();
ts.Complete();
}
catch (Exception ex)
{
PXTrace.WriteError(ex);
throw new PXOperationCompletedException("Error: Issue Generate New Line Items " + ex.Message);
}
}
}
}
I would like it to display like this when pressing Demo Processing
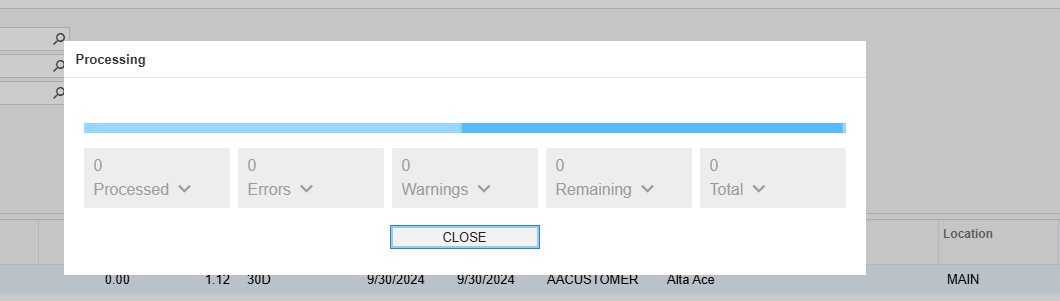
But click the Demo Processing button, it doesn't show the Processing dialog, it shows like this.
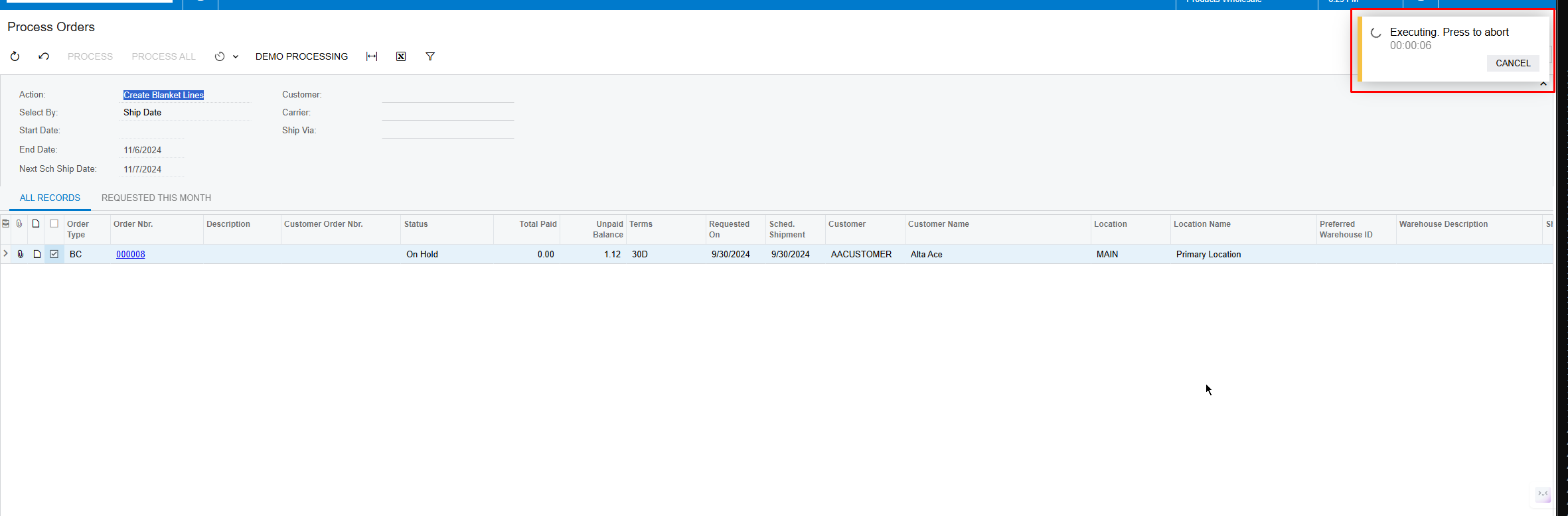
I don't know if there is a missing or incorrect piece of code that does it? it not show Processing dialog. So please help me with this problem?