Hi,
I have added a selector field (Location) in the Lot/Serial Nbr field in the Sales Order line details form as per the screenshots.
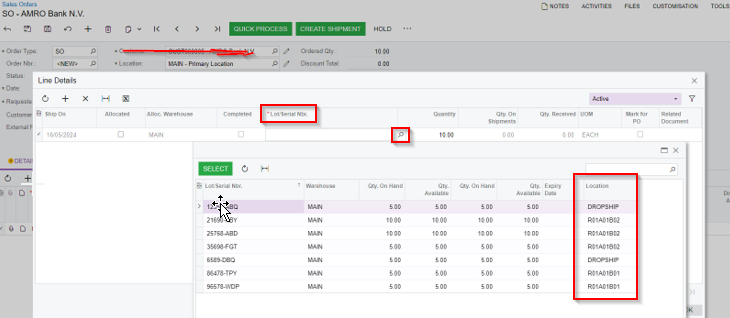
This item has the same lot number in different locations under the same warehouse.
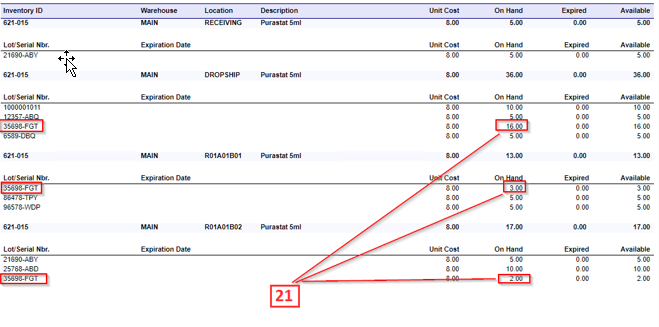
Follow the navigation below.
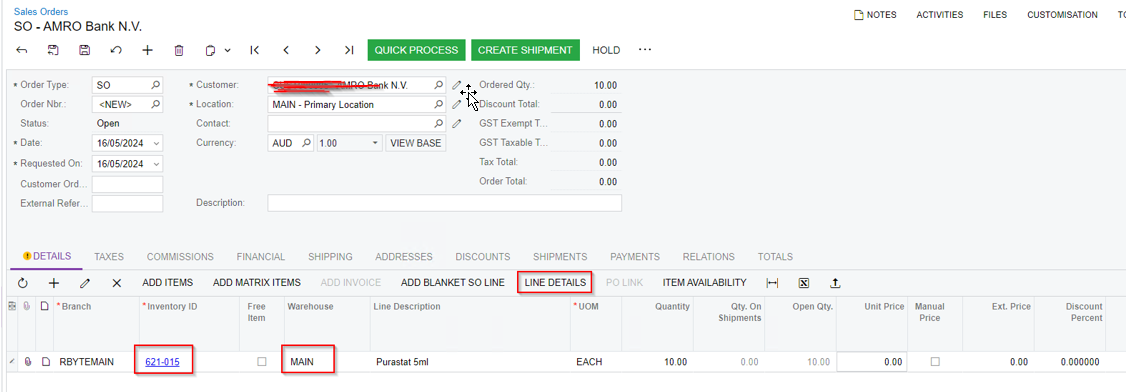
The system cumulatively shows information based on the lot number. I need to see on-hand information with different locations as per the screenshot.
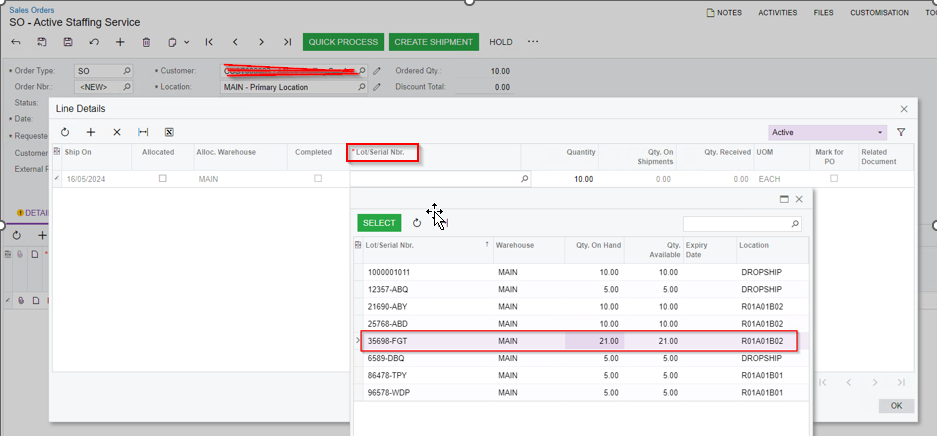
The expected result should be as follows: the system should not accumulate the values and should display all the same lot number information for different locations.The above screen 21 onhand qty should be split.

------------------------------------------------------------------------------------------------------------------------
I found the following configuration steps. I am not sure exactly how to apply them.
Can someone help me resolve this?
Detailed Implementation
1. Modify the Data Retrieval Query
Ensure the query fetches lot numbers with their respective locations and quantities.
sql
Copy code
SELECT
LotNumber,
Location,
Quantity
FROM
Inventory
WHERE
WarehouseID = @WarehouseID
ORDER BY
LotNumber, Location
2. Customize the Selector Logic
Adjust the selector field logic to display the lot numbers by location. This can typically be done in the backend code where the selector is populated.
Example in C#:
csharp
Copy code
public List<LotDetails> GetLotDetailsByWarehouse(string warehouseId)
{
// Fetch data from the database
var lotDetails = new List<LotDetails>();
// Populate lotDetails with data from the database query
return lotDetails;
}
public void PopulateLotNumberSelector(string warehouseId)
{
var lotDetails = GetLotDetailsByWarehouse(warehouseId);
lotNumberSelector.DataSource = lotDetails;
lotNumberSelector.DataTextField = "LotNumber"; // Display field
lotNumberSelector.DataValueField = "LotNumber"; // Value field
lotNumberSelector.DataBind();
}
3. Update the User Interface
Ensure the UI correctly displays the lot numbers with their locations and quantities.
Example in ASP.NET:
Define the grid in your ASP.NET page:
html
Copy code
<asp:GridView ID="lotDetailsGrid" runat="server" AutoGenerateColumns="False">
<Columns>
<asp:BoundField DataField="LotNumber" HeaderText="Lot Number" />
<asp:BoundField DataField="Location" HeaderText="Location" />
<asp:BoundField DataField="Quantity" HeaderText="Quantity" />
</Columns>
</asp:GridView>
Bind the data in the code-behind:
csharp
Copy code
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
PopulateLotNumberSelector("WarehouseID");
}
}
private void PopulateLotNumberSelector(string warehouseId)
{
var lotDetails = GetLotDetailsByWarehouse(warehouseId);
lotDetailsGrid.DataSource = lotDetails;
lotDetailsGrid.DataBind();
}
By following these steps, you can adjust the selector behavior to display lot numbers separately based on their locations within the same warehouse, ensuring accurate and detailed visibility of the lot quantities.