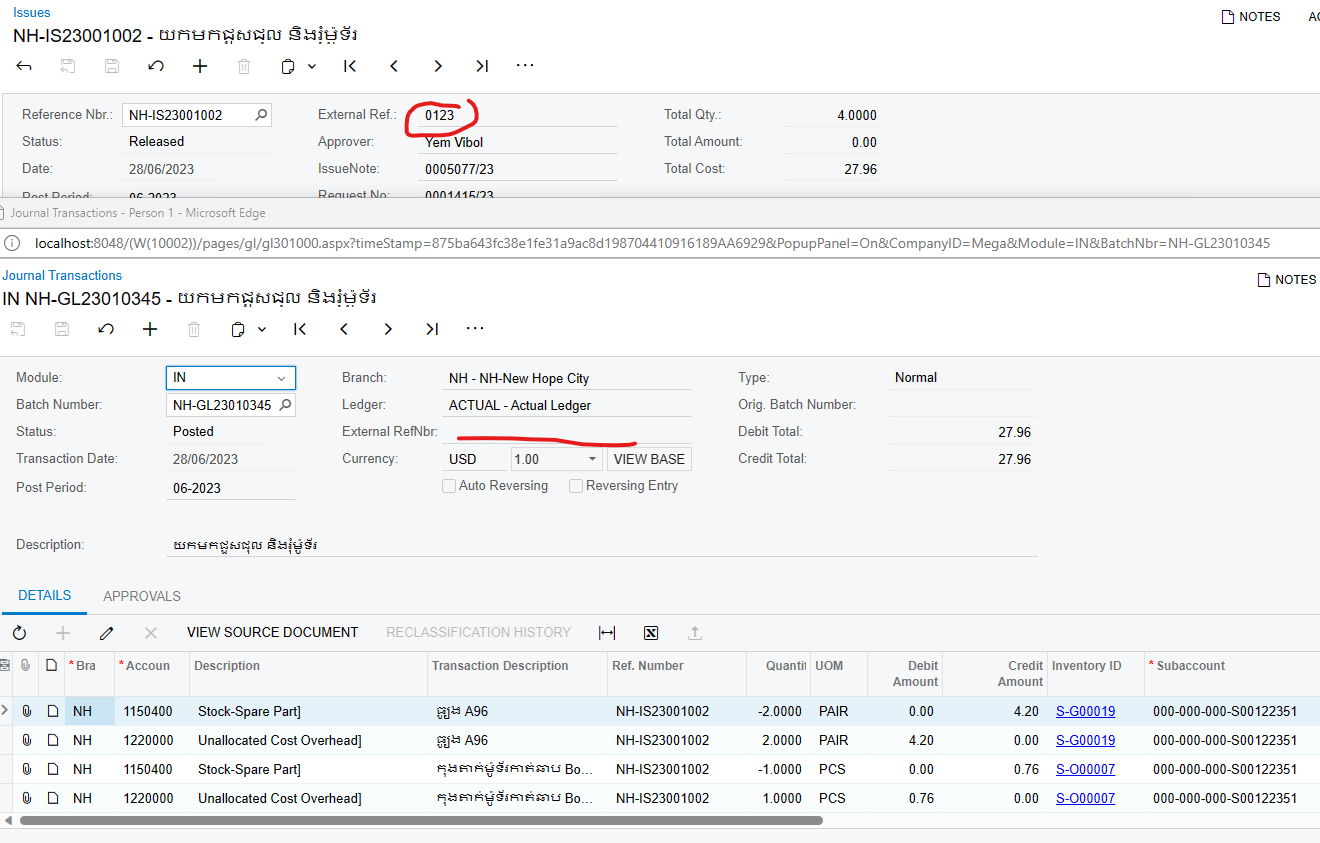
Best answer by vardan22
View originalI want to pass ext ref in issue screen to my custom field in journal screen when release action in issue screen.
You just need to override the ReleaseDocProc method specified in the INReleaseProcess graph.
public class INReleaseProcessExt : PXGraphExtension<INReleaseProcess>
{
public static bool IsActive() => true;
#region PXOverride
public delegate void ReleaseDocProcDelegate(JournalEntry je, INRegister doc, bool releaseFromHold = false);
[PXOverride]
public virtual void ReleaseDocProc(JournalEntry je, INRegister doc, bool releaseFromHold, ReleaseDocProcDelegate releaseDocProc)
{
releaseDocProc(je, doc, releaseFromHold);
Batch batch = je.BatchModule.Current;
if (batch != null && doc.DocType == INDocType.Issue)
{
batch.Description = doc.ExtRefNbr;
//for extension fields need to get an extension class and then the fields
je.BatchModule.Update(batch);
je.Save.Press();
}
}
#endregion
}
You just need to override the ReleaseDocProc method specified in the INReleaseProcess graph.
public class INReleaseProcessExt : PXGraphExtension<INReleaseProcess>
{
public static bool IsActive() => true;
#region PXOverride
public delegate void ReleaseDocProcDelegate(JournalEntry je, INRegister doc, bool releaseFromHold = false);
[PXOverride]
public virtual void ReleaseDocProc(JournalEntry je, INRegister doc, bool releaseFromHold, ReleaseDocProcDelegate releaseDocProc)
{
releaseDocProc(je, doc, releaseFromHold);
Batch batch = je.BatchModule.Current;
if (batch != null && doc.DocType == INDocType.Issue)
{
batch.Description = doc.ExtRefNbr;
//for extension fields need to get an extension class and then the fields
je.BatchModule.Update(batch);
je.Save.Press();
}
}
#endregion
}
I try with your code “description field” has updated but extension fields not update. here is my code
public class INReleaseProcess_Extension : PXGraphExtension<INReleaseProcess>
{
// Acuminator disable once PX1096 PXOverrideSignatureMismatch [Justification]
public delegate void ReleaseDocProcDelegate(JournalEntry je, INRegister doc, bool releaseFromHold);
[PXOverride]
public void ReleaseDocProc(
JournalEntry je,
INRegister doc, bool releaseFromHold,
INReleaseProcess_Extension.ReleaseDocProcDelegate baseMethod)
{
//je.RowInserted.AddHandler<Batch>(((cache, e) =>
//{
// Batch glBatch = e.Row as Batch;
// BatchExt batch = PXCache<Batch>.GetExtension<BatchExt>(glBatch);
// batch.UsrExternalRefNbr = doc.ExtRefNbr;
// //je.BatchModule.Current.GetExtension<BatchExt>().UsrExternalRefNbr = doc.ExtRefNbr;
// //je.BatchModule.Current.Description = "test1";
//}
//));
baseMethod(je, doc, releaseFromHold);
Batch batch = je.BatchModule.Current;
if (batch != null && doc.DocType == INDocType.Issue)
{
//batch.Description = doc.ExtRefNbr;
BatchExt batchext = PXCache<Batch>.GetExtension<BatchExt>(batch);
batchext.UsrExternalRefNbr = doc.ExtRefNbr;
batch.Description = doc.ExtRefNbr.ToString();
//for extension fields need to get an extension class and then the fields
je.BatchModule.Update(batch);
je.Save.Press();
}
}
}
You just need to override the ReleaseDocProc method specified in the INReleaseProcess graph.
public class INReleaseProcessExt : PXGraphExtension<INReleaseProcess>
{
public static bool IsActive() => true;
#region PXOverride
public delegate void ReleaseDocProcDelegate(JournalEntry je, INRegister doc, bool releaseFromHold = false);
[PXOverride]
public virtual void ReleaseDocProc(JournalEntry je, INRegister doc, bool releaseFromHold, ReleaseDocProcDelegate releaseDocProc)
{
releaseDocProc(je, doc, releaseFromHold);
Batch batch = je.BatchModule.Current;
if (batch != null && doc.DocType == INDocType.Issue)
{
batch.Description = doc.ExtRefNbr;
//for extension fields need to get an extension class and then the fields
je.BatchModule.Update(batch);
je.Save.Press();
}
}
#endregion
}
I try with your code “description field” has updated but extension fields not update. here is my code
public class INReleaseProcess_Extension : PXGraphExtension<INReleaseProcess>
{
// Acuminator disable once PX1096 PXOverrideSignatureMismatch [Justification]
public delegate void ReleaseDocProcDelegate(JournalEntry je, INRegister doc, bool releaseFromHold);
[PXOverride]
public void ReleaseDocProc(
JournalEntry je,
INRegister doc, bool releaseFromHold,
INReleaseProcess_Extension.ReleaseDocProcDelegate baseMethod)
{
//je.RowInserted.AddHandler<Batch>(((cache, e) =>
//{
// Batch glBatch = e.Row as Batch;
// BatchExt batch = PXCache<Batch>.GetExtension<BatchExt>(glBatch);
// batch.UsrExternalRefNbr = doc.ExtRefNbr;
// //je.BatchModule.Current.GetExtension<BatchExt>().UsrExternalRefNbr = doc.ExtRefNbr;
// //je.BatchModule.Current.Description = "test1";
//}
//));
baseMethod(je, doc, releaseFromHold);
Batch batch = je.BatchModule.Current;
if (batch != null && doc.DocType == INDocType.Issue)
{
//batch.Description = doc.ExtRefNbr;
BatchExt batchext = PXCache<Batch>.GetExtension<BatchExt>(batch);
batchext.UsrExternalRefNbr = doc.ExtRefNbr;
batch.Description = doc.ExtRefNbr.ToString();
//for extension fields need to get an extension class and then the fields
je.BatchModule.Update(batch);
je.Save.Press();
}
}
}
I solved it by je.BatchModule.SetValueExt<BatchExt.usrExternalRefNbr>(batch,doc.ExtRefNbr);
thank you very much
Enter your E-mail address. We'll send you an e-mail with instructions to reset your password.