Hi,
I’m having trouble with one of my projects.
I created a screen linked to a table I made. When I select my client through the selector, the client’s data is displayed in the designated fields.
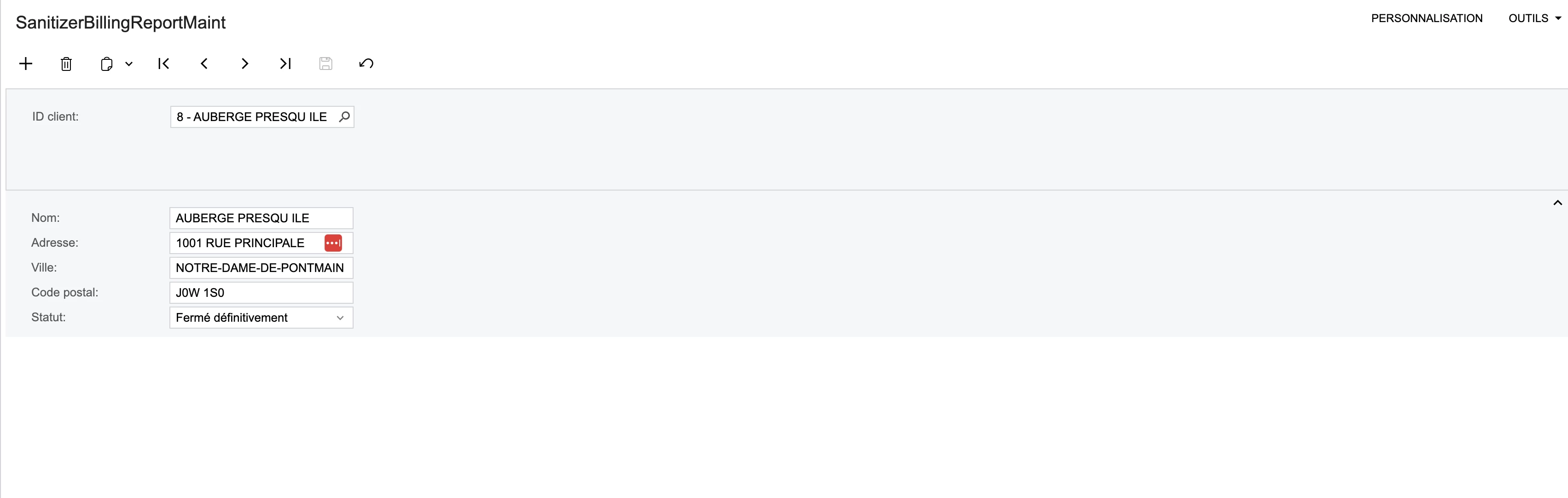
However, when I try to add new data by pressing the "+", I encounter a problem: the default client ID is set to -2147483647. When I enter information into the form and save, two rows are created in the table—one with a new ID but empty fields, and another with the values I entered into the form.
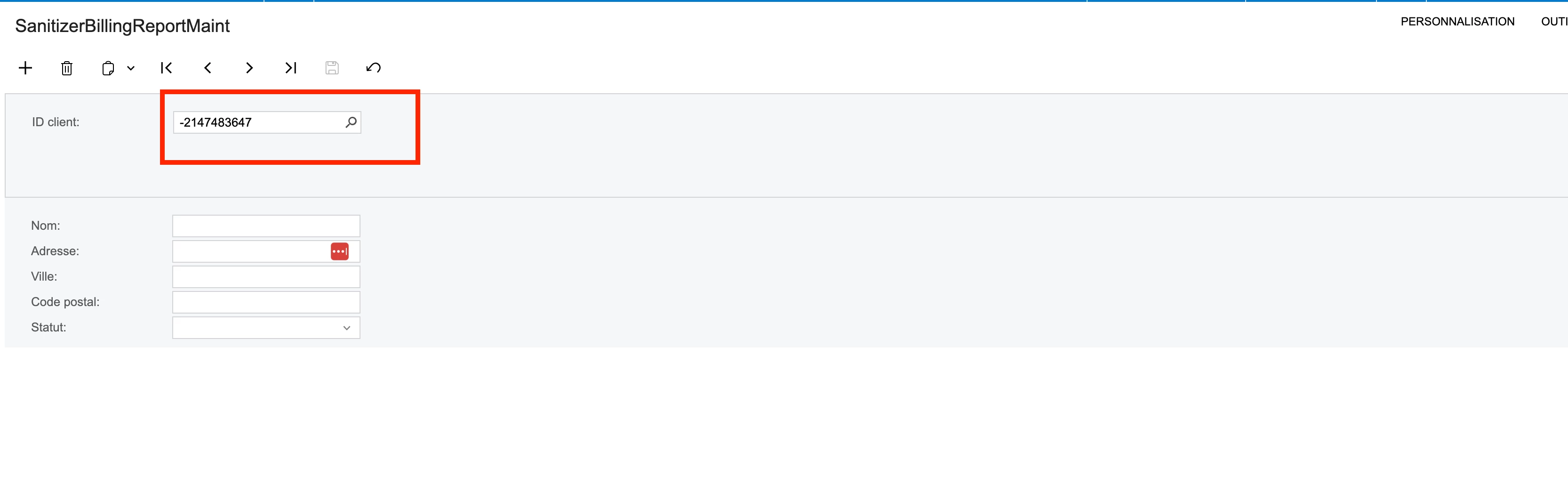