I’ve been asked to provide an estimate for a new customization. I’ve done several advance things in Acumatica but this one I’m wondering if a) is this possible? and/or b) should it even be attempted?
- They want to use Matrix Items with 8 attributes in a template.
- They want to be able to add cost/labor to each attribute value and have the price calculated during entry and saved directly to AR prices when item is created
Add custom fields to Attribute values:
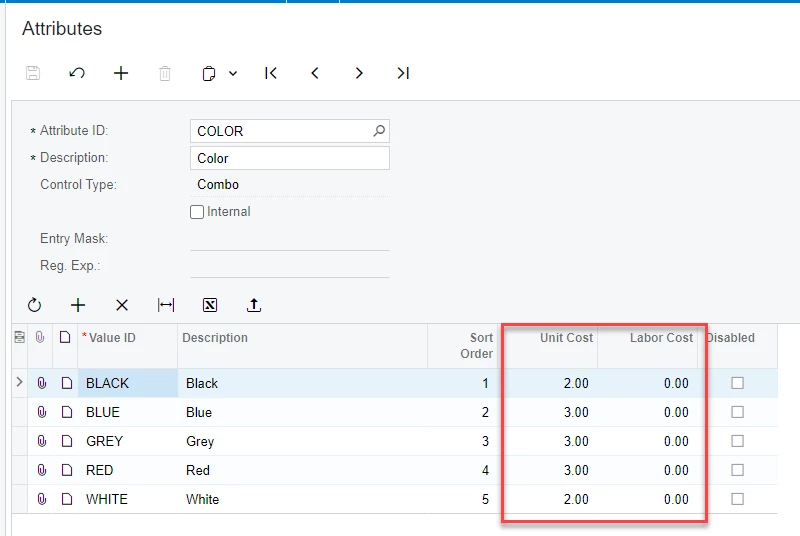
Use these values to calculate a price during matrix entry:
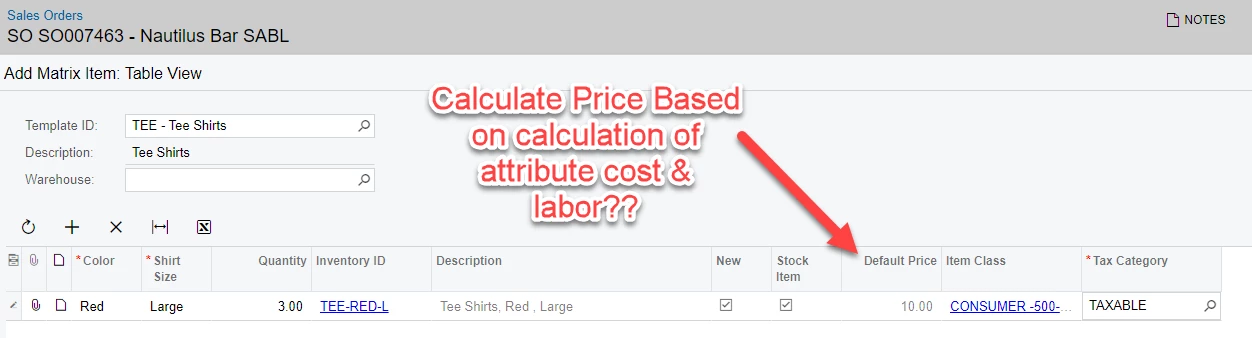
This does not look like traditional graph extension with overrides. I’ve tracked down some code PX.Objects\IN\Matrix\GraphExtensions\HeaderAndAttributesExt.cs
which looks like where the fieldupdating event is being hooked up to each attribute value but I can’t see a way to sub-class or extend this abstract class.
I guess I’m at the point where I just need to hear if this is a fools errand and/or if there is some other possible way to accomplish this. Customer does not want/need e-commerce integration nor do they want to purchase the product configurator.