I have created a custom screen which contains two grids in separate tabs. one grid is a read only grid. while other grid allows to CRUD operations. In this grid I use fully custom DAC. when I trying to insert data, even when insert correct values (not null) for each column sometimes it gives “cannot insert values to columns since null”.
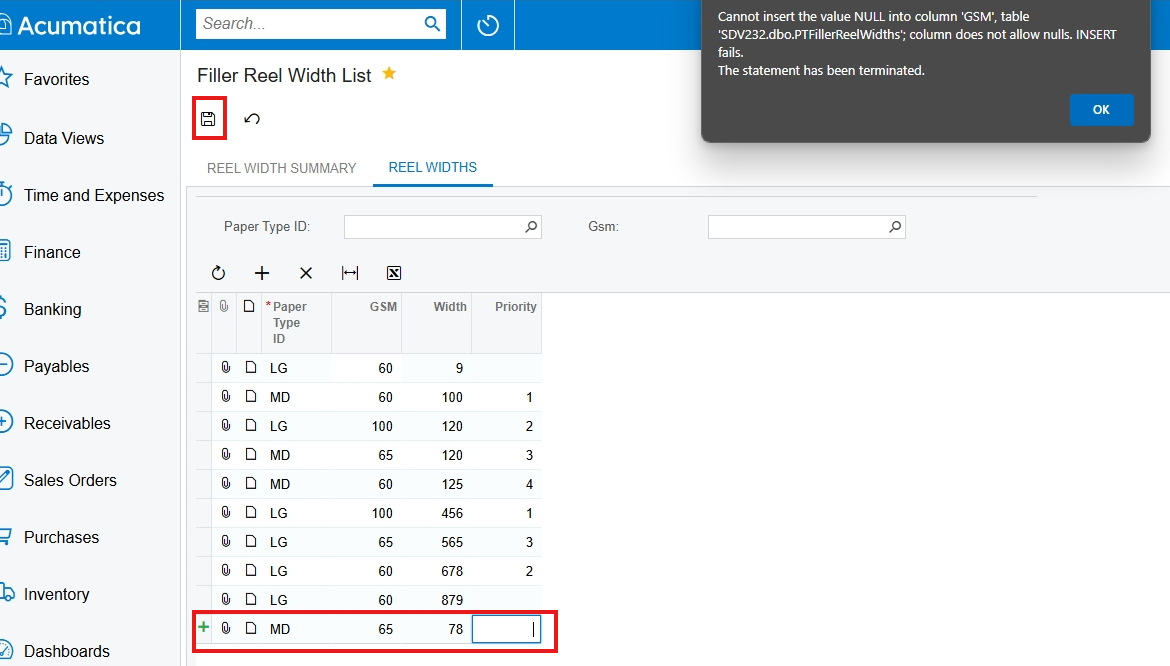
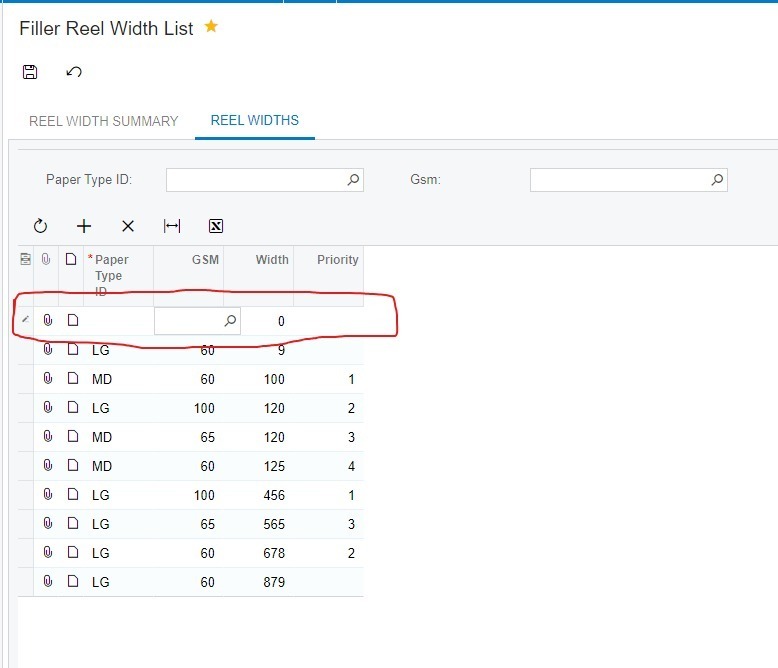
Even I set values after clicking save button it inserts an empty row as above.
DAC:
using System;
using PX.Data;
namespace EMPPlanningToolR4N5
{
[Serializable]
[PXCacheName("PTFillerReelWidths")]
public class PTFillerReelWidths : IBqlTable
{
#region Width
[PXDBInt(IsKey = true)]
[PXUIField(DisplayName = "Width")]
[PXDefault(0)]
public virtual int? Width { get; set; }
public abstract class width : PX.Data.BQL.BqlInt.Field<width> { }
#endregion
#region PaperTypeID
[PXDBString(2, IsKey = true, IsFixed = true, InputMask = "")]
[PXUIField(DisplayName = "Paper Type ID")]
[PXDefault("##")]
[PXSelector(typeof(PTPaperTypes.paperTypeID),
typeof(PTPaperTypes.paperTypeID),
typeof(PTPaperTypes.paperTypeCD),
SubstituteKey = typeof(PTPaperTypes.paperTypeID),
DescriptionField = typeof(PTPaperTypes.description)
)]
public virtual string PaperTypeID { get; set; }
public abstract class paperTypeID : PX.Data.BQL.BqlString.Field<paperTypeID> { }
#endregion
#region Gsm
[PXDBInt(IsKey = true)]
[PXUIField(DisplayName = "GSM")]
[PXSelector(typeof(PTFillerReelGSMs.gsm),
typeof(PTFillerReelGSMs.gsm)
)]
public virtual int? Gsm { get; set; }
public abstract class gsm : PX.Data.BQL.BqlInt.Field<gsm> { }
#endregion
#region Priority
[PXDBInt()]
[PXUIField(DisplayName = "Priority")]
public virtual int? Priority { get; set; }
public abstract class priority : PX.Data.BQL.BqlInt.Field<priority> { }
#endregion
}
}
Graph :
using System;
using System.Collections;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using PX.Data;
using PX.Data.BQL.Fluent;
namespace EMPPlanningToolR4N5
{
public class PTFillerReelWidthListMaint : PXGraph<PTFillerReelWidthListMaint>
{
public SelectFrom<PTFillerReelWidths>.View FillerReelWidthView;
public SelectFrom<PTFillerReelWidthSummary>.View FillerReelWidthSummaryView;
public PXFilter<PTFillerReelWidthsFilter> ReelWidthFilter;
public PXFilter<PTFillerReelWidthsFilter> ReelWidthSummaryFilter;
public PXSave<PTFillerReelWidths> Save;
public PXCancel<PTFillerReelWidths> Cancel;
public PTFillerReelWidthListMaint()
{
FillerReelWidthSummaryView.AllowDelete = false;
FillerReelWidthSummaryView.AllowInsert = false;
}
public IEnumerable fillerReelWidthSummaryView()
{
var query = SelectFrom<PTFillerReelWidths>.View.Select(this).RowCast<PTFillerReelWidths>();
PTFillerReelWidthsFilter filter = ReelWidthSummaryFilter.Current;
PXTrace.WriteInformation($"Filter : {filter.Gsm}, {filter.PaperTypeID}");
if (!string.IsNullOrEmpty(filter?.PaperTypeID))
{
query = query.Where(x => x.PaperTypeID == filter.PaperTypeID);
}
if (filter?.Gsm != null)
{
query = query.Where(x => x.Gsm == filter.Gsm);
}
query = query.ToList();
var groupedData = query
.GroupBy(x => new { x.PaperTypeID, x.Gsm })
.Select(g => new PTFillerReelWidthSummary
{
PaperTypeID = g.Key.PaperTypeID,
Gsm = g.Key.Gsm,
WidthCount = g.Count()
}).ToList();
return groupedData;
}
public IEnumerable fillerReelWidthView()
{
var query = SelectFrom<PTFillerReelWidths>.View.Select(this).RowCast<PTFillerReelWidths>();
PTFillerReelWidthsFilter filter = ReelWidthFilter.Current;
PXTrace.WriteInformation($"Filter : {filter.Gsm}, {filter.PaperTypeID}");
if (!string.IsNullOrEmpty(filter?.PaperTypeID))
{
query = query.Where(x => x.PaperTypeID == filter.PaperTypeID);
}
if (filter?.Gsm != null)
{
query = query.Where(x => x.Gsm == filter.Gsm);
}
return query.ToList();
}
}
[PXHidden]
public class PTFillerReelWidthsFilter : IBqlTable
{
#region PaperTypeID
[PXString]
[PXUIField(DisplayName = "Paper Type ID")]
[PXSelector(typeof(PTPaperTypes.paperTypeID),
typeof(PTPaperTypes.paperTypeID),
typeof(PTPaperTypes.paperTypeCD),
SubstituteKey = typeof(PTPaperTypes.paperTypeID),
DescriptionField = typeof(PTPaperTypes.description)
)]
public virtual string PaperTypeID { get; set; }
public abstract class paperTypeID : PX.Data.BQL.BqlString.Field<paperTypeID> { }
#endregion
#region Gsm
[PXInt]
[PXUIField(DisplayName = "Gsm")]
[PXSelector(typeof(PTFillerReelGSMs.gsm),
typeof(PTFillerReelGSMs.gsm)
)]
public virtual int? Gsm { get; set; }
public abstract class gsm : PX.Data.BQL.BqlInt.Field<gsm> { }
#endregion
}
}