I am currently writing logic to automatically create move transaction records from the materials screen. Currently the records are being created successfully via code but when I try to release the move transaction automatically I get the following error: “Parent Lot/ Serial Number is required.”
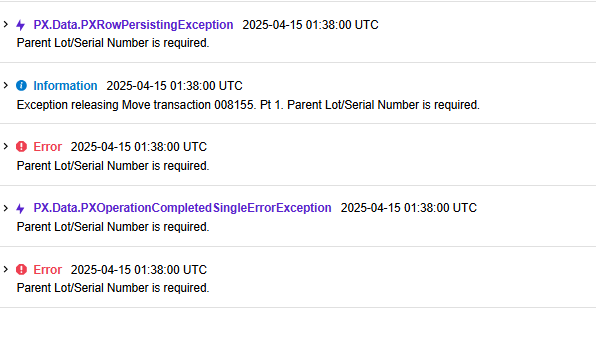
But on creating the move transaction automatically the parent lot/ serial number is being correctly assigned as below:
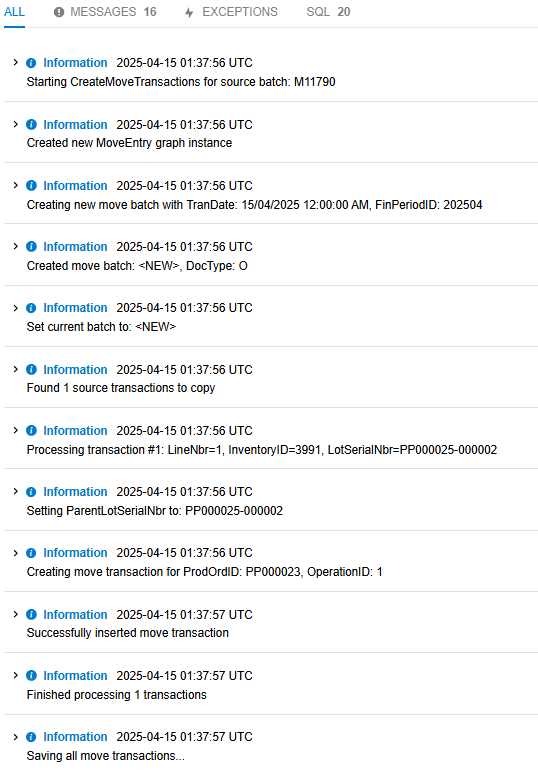

using System;
using System.Linq;
using System.Collections;
using System.Collections.Generic;
using PX.Data;
using PX.Data.BQL;
using PX.Data.BQL.Fluent;
using PX.Objects.Common;
using PX.Objects.Common.Exceptions;
using PX.Objects.IN;
using PX.Objects.CM;
using PX.Objects.CS;
using PX.Objects.AM.Attributes;
using PX.Objects.PM;
using PX.Objects.GL;
using PX.Objects;
using PX.Objects.AM;
namespace PX.Objects.AM
{
public class MaterialEntry_Extension : PXGraphExtension<PX.Objects.AM.MaterialEntry>
{
#region Release Override
public delegate IEnumerable ReleaseDelegate(PXAdapter adapter);
[PXOverride]
public IEnumerable Release(PXAdapter adapter, ReleaseDelegate baseMethod)
{
// First, execute the base release method to ensure original functionality
var documents = baseMethod(adapter).Cast<AMBatch>().ToList();
// Process move transactions after base release
foreach (AMBatch batch in documents)
{
CreateMoveTransactions(batch);
}
return documents;
}
#endregion
private void CreateMoveTransactions(AMBatch batch)
{
try
{
PXTrace.WriteInformation($"Starting CreateMoveTransactions for source batch: {batch.BatNbr}");
var moveGraph = PXGraph.CreateInstance<MoveEntry>();
PXTrace.WriteInformation($"Created new MoveEntry graph instance");
// Create and insert the move batch
PXTrace.WriteInformation($"Creating new move batch with TranDate: {batch.TranDate}, FinPeriodID: {batch.FinPeriodID}");
var moveBatch = moveGraph.batch.Insert(new AMBatch
{
TranDate = batch.TranDate,
FinPeriodID = batch.FinPeriodID,
Hold = false
});
if (moveBatch == null)
{
PXTrace.WriteError("Failed to insert move batch - batch is null");
throw new PXException("Failed to create move batch.");
}
PXTrace.WriteInformation($"Created move batch: {moveBatch.BatNbr}, DocType: {moveBatch.DocType}");
// Set current batch
moveGraph.batch.Current = moveBatch;
PXTrace.WriteInformation($"Set current batch to: {moveBatch.BatNbr}");
// Copy transactions from original batch
int transactionCount = 0;
var materialTrans = PXSelect<AMMTran,
Where<AMMTran.batNbr, Equal<Required<AMMTran.batNbr>>,
And<AMMTran.docType, Equal<Required<AMMTran.docType>>>>>
.Select(moveGraph, batch.BatNbr, batch.DocType);
PXTrace.WriteInformation($"Found {materialTrans.Count} source transactions to copy");
foreach (AMMTran mtran in materialTrans)
{
transactionCount++;
PXTrace.WriteInformation($"Processing transaction #{transactionCount}: LineNbr={mtran.LineNbr}, InventoryID={mtran.InventoryID}, LotSerialNbr={mtran.LotSerialNbr}");
var parentLotSerial = string.IsNullOrEmpty(mtran.ParentLotSerialNbr)
? mtran.LotSerialNbr
: mtran.ParentLotSerialNbr;
PXTrace.WriteInformation($"Setting ParentLotSerialNbr to: {parentLotSerial}");
var moveTran = new AMMTran
{
OrderType = mtran.OrderType,
ProdOrdID = mtran.ProdOrdID,
OperationID = mtran.OperationID,
SiteID = mtran.SiteID,
LocationID = mtran.LocationID,
LotSerialNbr = mtran.LotSerialNbr,
ParentLotSerialNbr = parentLotSerial,
Qty = 1m
};
PXTrace.WriteInformation($"Creating move transaction for ProdOrdID: {moveTran.ProdOrdID}, OperationID: {moveTran.OperationID}");
moveGraph.transactions.Insert(moveTran);
PXTrace.WriteInformation($"Successfully inserted move transaction");
}
PXTrace.WriteInformation($"Finished processing {transactionCount} transactions");
// Save transactions
PXTrace.WriteInformation("Saving all move transactions...");
moveGraph.Actions.PressSave();
PXTrace.WriteInformation($"Successfully saved {transactionCount} transactions in batch {moveBatch.BatNbr}");
// SIMPLE RELEASE - Just press the release button
PXTrace.WriteInformation($"Attempting to release move batch {moveBatch.BatNbr}...");
moveGraph.release.Press();
PXTrace.WriteInformation($"Successfully released move batch {moveBatch.BatNbr}");
}
catch (Exception ex)
{
PXTrace.WriteError($"Error in CreateMoveTransactions: {ex.ToString()}");
throw;
}
}
#endregion
}
}
Please let me know what I might be missing, thank you!