I have a requirement to create a purchase receipt using Acumatica API.
This is my code for that.
RootObject obj = new RootObject();
obj.Type = "Receipt";
obj.VendorID = "GRI SL";
obj.VendorRef = "2131";
obj.Locatrion = "Main";
obj.ShipmentRef = "00028";
obj.Date = DateTime.ParseExact("10/10/2022", "dd/MM/yyyy",CultureInfo.InvariantCulture);
obj.PostPeriod = DateTime.ParseExact("10/10/2022", "dd/MM/yyyy",CultureInfo.InvariantCulture);
var jsonStrings = JsonConvert.SerializeObject(obj);
PXTrace.WriteInformation(jsonStrings);
var jsonString = JObject.Parse(jsonStrings);
PXTrace.WriteInformation(jsonString.ToString());
var URL = "https://globalrubberindustrieslimited.acumatica.com/entity/CustomEndpoint/22.200.001/PurchaseReceipt";
var httpRequest = (HttpWebRequest)WebRequest.Create(URL);
httpRequest.Method = "PUT";
httpRequest.ContentType = "application/json";
httpRequest.Headers.Add("Authorization", "Bearer " + my token);
httpRequest.SendChunked = true;
using (var streamWriter = new StreamWriter(httpRequest.GetRequestStream()))
{
streamWriter.Write(jsonString);
}
var httpResponse = (HttpWebResponse)httpRequest.GetResponse();
using (var streamReader = new StreamReader(httpResponse.GetResponseStream()))
{
var responseText = streamReader.ReadToEnd();
var responseJson = JsonConvert.DeserializeObject(responseText);
PXTrace.WriteInformation(responseText);
}
Json object in Trace
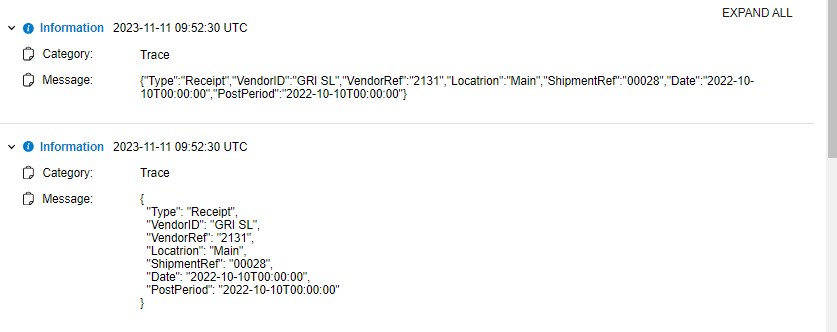
But , when calling the API It shows an error.And also ,When I use the same json via Postman It shows the same error.
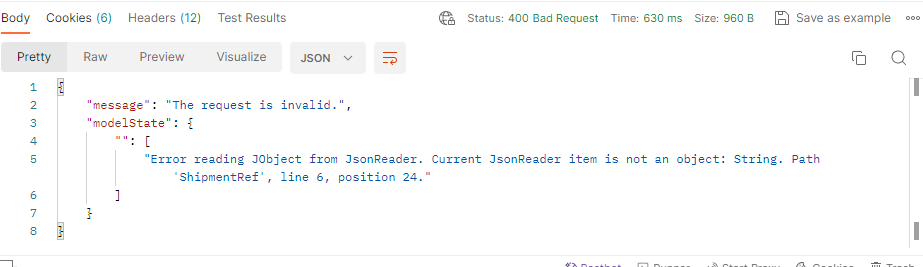
Can someone please help me for that.
Best answer by jinin
View original