I am trying to add a grid to store values to my custom DAC on Projects screen. But I am not able to add a new row. When I try to add new row the row is added momentarily and then just vanishes. I checked the trace and it is showing this exception. ComplianceDocuments view is available in the Acumatica code.
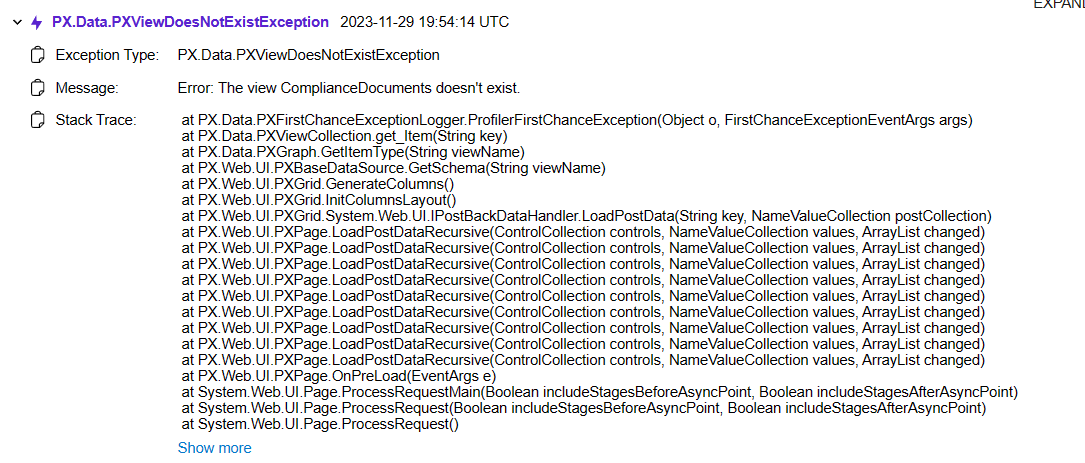
My Custom DAC code
using System;
using PX.Data;
namespace MyCust
{
[Serializable]
[PXCacheName("MyDAC")]
public class MyDAC : IBqlTable
{
#region ProjectID
[PXDBInt(IsKey = true)]
[PXUIField(DisplayName = "Project ID")]
public virtual int? ProjectID { get; set; }
public abstract class projectID : PX.Data.BQL.BqlInt.Field<projectID> { }
#endregion
#region Id
[PXDBIdentity(IsKey = true)]
public virtual int? Id { get; set; }
public abstract class id : PX.Data.BQL.BqlInt.Field<id> { }
#endregion
#region CreatedDateTime
[PXDBCreatedDateTime()]
public virtual DateTime? CreatedDateTime { get; set; }
public abstract class createdDateTime : PX.Data.BQL.BqlDateTime.Field<createdDateTime> { }
#endregion
#region CreatedByID
[PXDBCreatedByID()]
public virtual Guid? CreatedByID { get; set; }
public abstract class createdByID : PX.Data.BQL.BqlGuid.Field<createdByID> { }
#endregion
#region CreatedByScreenID
[PXDBCreatedByScreenID()]
public virtual string CreatedByScreenID { get; set; }
public abstract class createdByScreenID : PX.Data.BQL.BqlString.Field<createdByScreenID> { }
#endregion
#region LastModifiedDateTime
[PXDBLastModifiedDateTime()]
public virtual DateTime? LastModifiedDateTime { get; set; }
public abstract class lastModifiedDateTime : PX.Data.BQL.BqlDateTime.Field<lastModifiedDateTime> { }
#endregion
#region LastModifiedByID
[PXDBLastModifiedByID()]
public virtual Guid? LastModifiedByID { get; set; }
public abstract class lastModifiedByID : PX.Data.BQL.BqlGuid.Field<lastModifiedByID> { }
#endregion
#region LastModifiedByScreenID
[PXDBLastModifiedByScreenID()]
public virtual string LastModifiedByScreenID { get; set; }
public abstract class lastModifiedByScreenID : PX.Data.BQL.BqlString.Field<lastModifiedByScreenID> { }
#endregion
#region Tstamp
[PXDBTimestamp()]
[PXUIField(DisplayName = "Tstamp")]
public virtual byte[] Tstamp { get; set; }
public abstract class tstamp : PX.Data.BQL.BqlByteArray.Field<tstamp> { }
#endregion
#region Noteid
[PXNote()]
public virtual Guid? Noteid { get; set; }
public abstract class noteid : PX.Data.BQL.BqlGuid.Field<noteid> { }
#endregion
#region Description
[PXDBString(500, IsUnicode = true, InputMask = "")]
[PXUIField(DisplayName = "Description")]
public virtual string Description { get; set; }
public abstract class description : PX.Data.BQL.BqlString.Field<description> { }
#endregion
#region Percentage
[PXDBDecimal()]
[PXUIField(DisplayName = "Percentage")]
public virtual Decimal? Percentage { get; set; }
public abstract class percentage : PX.Data.BQL.BqlDecimal.Field<percentage> { }
#endregion
#region CalculatePrice
[PXDBDecimal()]
[PXUIField(DisplayName = "Calculate Price")]
public virtual Decimal? CalculatePrice { get; set; }
public abstract class calculatePrice : PX.Data.BQL.BqlDecimal.Field<calculatePrice> { }
#endregion
}
}
And this is the declared view in GraphExt
public PXSelect<MyDAC, Where<MyDAC.projectID, Equal<Current<PMProject.contractID>>>> MyView;
ASPX Code for the grid added to Summary tab in screen (PM301000)
<px:PXGrid runat="server" ID="CstPXGrid3" SkinID="Details">
<Levels>
<px:PXGridLevel DataMember="MyView">
<Columns>
<px:PXGridColumn DataField="Percentage" Width="100" />
<px:PXGridColumn DataField="Description" Width="280" />
<px:PXGridColumn DataField="CalculatePrice" Width="100" /></Columns></px:PXGridLevel></Levels></px:PXGrid>
Best answer by darylbowman
View original