I added a custom field to the Equipment screen FS205000.
I am looking up the AMProdID and storing it in the custom field.
The rowselected handler is getting the value and it shows on the screen. I set the cache to IsDirty = true so that the user will need to click save when the rowselected does not find a Production Nbr in the custom field.
If I don’t set cache.IsDirty = true, then it does not show that the record needs to be saved.
When you click the Save button, it does not save the value in the FSEquipment table.
This is the screen and it shows the value I am adding to the screen:
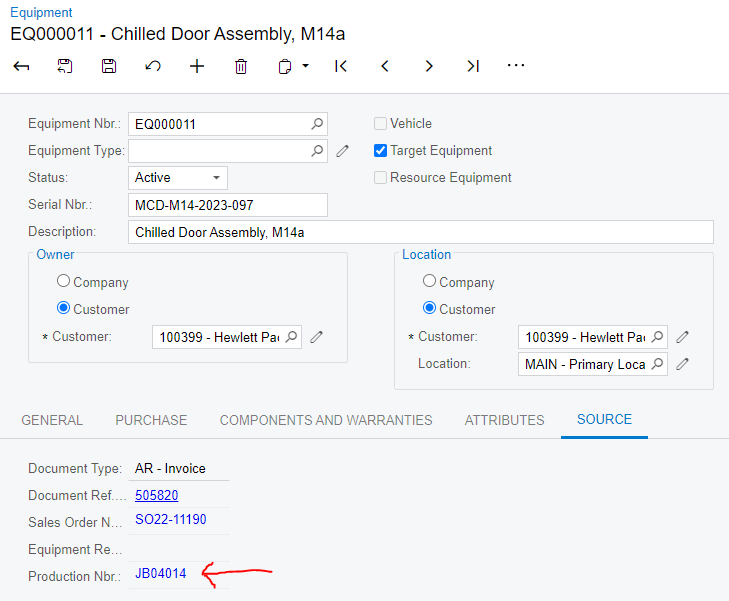
IF I change the Description and click Save, it DOES save the custom field.
Here is the graph extension:
public class SMEquipmentMaint_Extension : PXGraphExtension<SMEquipmentMaint>
{
public static bool IsActive() => true;
#region Event Handlers
protected void FSEquipment_RowSelected(PXCache cache, PXRowSelectedEventArgs e)
{
var row = (FSEquipment)e.Row;
FSEquipmentExt itemExt = PXCache<FSEquipment>.GetExtension<FSEquipmentExt>(row);
if (itemExt == null) return;
//if already has a value, exit method
if (itemExt.UsrAMProdOrdID != null) return;
AMProdItem aMProdItem = SelectFrom<AMProdItem>
.InnerJoin<SOLineMfgOnly>.On<SOLineMfgOnly.aMProdOrdID.IsEqual<AMProdItem.prodOrdID>>
.InnerJoin<ARTran>.On<ARTran.sOOrderNbr.IsEqual<SOLineMfgOnly.orderNbr>.And<ARTran.sOOrderLineNbr.IsEqual<SOLineMfgOnly.lineNbr>>>
.InnerJoin<FSEquipment>.On<FSEquipment.sourceRefNbr.IsEqual<ARTran.refNbr>.And<FSEquipment.arTranLineNbr.IsEqual<ARTran.lineNbr>>>
.Where<FSEquipment.SMequipmentID.IsEqual<FSEquipment.SMequipmentID.FromCurrent>.And<FSEquipment.sourceRefNbr.IsEqual<FSEquipment.sourceRefNbr.FromCurrent>>>
.AggregateTo<GroupBy<AMProdItem.prodOrdID>>.View.Select(Base);
if (aMProdItem != null)
{
itemExt.UsrAMProdOrdID = aMProdItem.ProdOrdID;
cache.IsDirty = true;
}
}
#endregion
}
This is the DAC extension. I used a selector so that you can drill into the Production Nbr field and open the Production Order Maintenance screen (which works great as is).
[PXDBString(15, IsUnicode = true, InputMask = "")]
[PXUIField(DisplayName = "Production Nbr.", Enabled = false)]
[PXSelector(typeof(SearchFor<AMProdItem.prodOrdID>.Where<AMProdItem.prodOrdID.IsEqual<FSEquipmentExt.usrAMProdOrdID.FromCurrent>>))]
public string UsrAMProdOrdID { get; set; }
public abstract class usrAMProdOrdID : PX.Data.BQL.BqlString.Field<usrAMProdOrdID> { }
Do I need to do something else to let the screen know the extension field has been updated?
I think this is the spot where I might need to change something:
if (aMProdItem != null)
{
itemExt.UsrAMProdOrdID = aMProdItem.ProdOrdID;
cache.IsDirty = true;
}
Best answer by Vignesh Ponnusamy
View original