I added a custom field to the Bills and Adjustments screen Details grid.
The users want to be able to lookup the project id by filtering on the Task ID. They KNOW the task ID, but they don’t know the Project and the Project lookup only lists the projects.
My custom lookup pulls from the PMTasks table and is joined to the PMProjects DAC. My lookup allows you to filter on the Task ID.
When you select the first line shown in the print screen, it should be returning Task ID 1652.
In the code, it is returning 1584 no matter which item is selected in the grid.
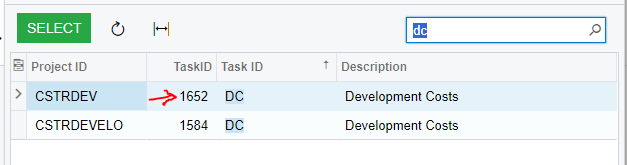
This is the DAC Extension showing my Selector. Is there something wrong with it? the Grid shows the TaskID so I can see it is unique. I don’t plan to show the integer, but I am showing it for debugging purposes, .
public sealed class APTranExt : PXCacheExtension<PX.Objects.AP.APTran>
{
#region UsrProjectTaskID
[PXUIField(DisplayName = "Lookup Project Task")]
[PXSelector(
typeof(Search2<PMTask.taskID,
InnerJoin<PMProject, On<PMProject.contractID, Equal<PMTask.projectID>>>,
Where<PMProject.isActive, Equal<True>>>),
typeof(PMProject.contractCD),
typeof(PMTask.taskID),
typeof(PMTask.taskCD),
typeof(PMTask.description),
SubstituteKey = typeof(PMTask.taskCD),
DescriptionField = typeof(PMTask.description))]
public int? UsrProjectTaskID { get; set; }
public abstract class usrProjectTaskID : PX.Data.BQL.BqlInt.Field<usrProjectTaskID> { }
#endregion
}
In debug, the value in the custom field is 1584, even though I selected the first record:
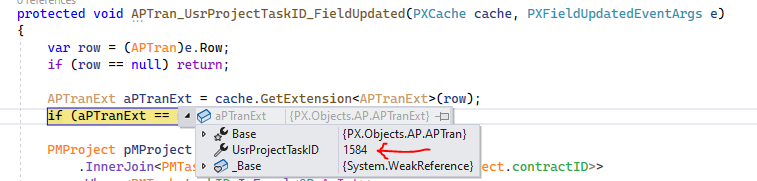
If I remove the join to the PMProject DAC, the same thing still happens. It returns 1584 no matter which record I choose.
Best answer by Joe Schmucker
View original