I’m using a dialog for data entry, and I’m having issues initializing the filter view with values before displaying the dialog.
I’ve tried several different approaches based on searching the Acumatica source, my latest attempt is in the following code block.
The dialog always initially shows without any of the pre set data displayed. If I enter in the additional data and save, a new record is correctly created with the prefilled values.
When I debug, I can see the values are updated before the AskExt call, they just do not display on form.
If I run the action a second time after the first record is updated, the dialog shows the previously input data including the prefilled values.
I seems like this should work, I’m handling for the multiple calls for the AskExt by checking the for Answer == None and only initializing the fiilter in that case. I’ve tried it with and without passing the RefreshRequired parameter as true. Same results.
Action - this is in POOrderEntry extension
public PXAction<AHSPOLineNonConfFilter> AddPOLineNonConf;
[PXUIField(DisplayName = "Add Non Conformance", MapEnableRights = PXCacheRights.Select, MapViewRights = PXCacheRights.Select)]
[PXButton(CommitChanges = true, Category = "Other", DisplayOnMainToolbar = false)]
protected virtual IEnumerable addPOLineNonConf(PXAdapter adapter)
{
PXTrace.WriteInformation("Reached the AddPOLineNonConf action");
AHSPOLineNonConfFilter nc = new AHSPOLineNonConfFilter();
POLine curline = Base.Transactions.Current;
// break out if no detail line is selected
if (curline == null) return adapter.Get();
if (CreateVendorNonConfFilterView.View.Answer == WebDialogResult.None)
{
CreateVendorNonConfFilterView.Reset();
nc.OrderType = curline.OrderType;
nc.OrderNbr = curline.OrderNbr;
nc.LineNbr = curline.LineNbr;
nc.InventoryID = curline.InventoryID;
nc.InventoryDescr = curline.TranDesc;
nc.ReceiptNbr = null;
nc.LotSerialNo = null;
nc.Quantity = null;
nc.NonConfReason = null;
nc.Resolution = null;
nc.NCNotes = null;
Base.Caches<AHSPOLineNonConfFilter>().Clear();
Base.Caches<AHSPOLineNonConfFilter>().Insert(nc);
Base.Caches<AHSPOLineNonConfFilter>().IsDirty = false;
// CreateVendorNonConfFilterView.SelectSingle();
CreateVendorNonConfFilterView.Current = nc;
CreateVendorNonConfFilterView.AskExt();
}
if (CreateVendorNonConfFilterView.View.Answer == WebDialogResult.OK)
{
// user selected ok to add new polinenonfrecord
nc = CreateVendorNonConfFilterView.Current;
if (nc != null)
{
// add new record
// valiation needed?
AHSPOLineNonConf NewNC = new AHSPOLineNonConf();
NewNC = AHSPOLineNonConfView.Insert(NewNC);
NewNC.ReceiptNbr = nc.ReceiptNbr;
NewNC.InventoryID = nc.InventoryID;
NewNC.InventoryDescr = nc.InventoryDescr;
NewNC.NonConfReason = nc.NonConfReason;
NewNC.Resolution = nc.Resolution;
NewNC.Quantity = nc.Quantity;
NewNC.NCNotes = nc.NCNotes;
AHSPOLineNonConfView.Update(NewNC);
Base.Save.Press();
}
}
//}
return adapter.Get();
}
Filter DAC
using System;
using PX.Data;
using PX.Data.BQL.Fluent;
using PX.Data.ReferentialIntegrity.Attributes;
using PX.Objects.IN;
namespace PX.Objects.PO
{
[Serializable]
[PXHidden]
public class AHSPOLineNonConfFilter : IBqlTable
{
#region OrderType
[PXString(2, IsFixed = true, InputMask = "")]
[PXUIField(DisplayName = "Order Type")]
public virtual string OrderType { get; set; }
public abstract class orderType : PX.Data.BQL.BqlString.Field<orderType> { }
#endregion
#region OrderNbr
[PXString(15, IsUnicode = true, InputMask = "")]
[PXUIField(DisplayName = "Order Nbr")]
public virtual string OrderNbr { get; set; }
public abstract class orderNbr : PX.Data.BQL.BqlString.Field<orderNbr> { }
#endregion
#region LineNbr
[PXInt()]
[PXUIField(DisplayName = "Line Nbr")]
public virtual int? LineNbr { get; set; }
public abstract class lineNbr : PX.Data.BQL.BqlInt.Field<lineNbr> { }
#endregion
#region ReceiptType
[PXUIField(DisplayName = "Type")]
[PXString(2, IsFixed = true)]
public virtual string ReceiptType { get; set; }
public abstract class receiptType : PX.Data.BQL.BqlString.Field<receiptType> { }
#endregion
#region ReceiptNbr
[PXString(15, IsUnicode = true, InputMask = "")]
[PXUIField(DisplayName = "Receipt Nbr.")]
[PXSelector(typeof(Search<POReceiptLine.receiptNbr, Where<POReceiptLine.pONbr, Equal<Current<AHSPOLineNonConfFilter.orderNbr>>, And<POReceiptLine.pOLineNbr, Equal<Current<AHSPOLineNonConfFilter.lineNbr>>>>>))]
[PXCustomizeSelectorColumns(typeof(POReceiptLine.receiptType), typeof(POReceiptLine.receiptNbr), typeof(POReceiptLine.lineNbr), typeof(POReceiptLine.pONbr), typeof(POReceiptLine.pOLineNbr))]
public virtual string ReceiptNbr { get; set; }
public abstract class receiptNbr : PX.Data.BQL.BqlString.Field<receiptNbr> { }
#endregion
#region Quantity
[PXInt()]
[PXUIField(DisplayName = "Quantity")]
public virtual int? Quantity { get; set; }
public abstract class quantity : PX.Data.BQL.BqlInt.Field<quantity> { }
#endregion
#region LotSerialNo
[PXString(255, IsUnicode = true, InputMask = "")]
[PXUIField(DisplayName = "Lot Serial No")]
public virtual string LotSerialNo { get; set; }
public abstract class lotSerialNo : PX.Data.BQL.BqlString.Field<lotSerialNo> { }
#endregion
#region InventoryID
[PXInt()]
[PXUIField(DisplayName = "Inventory ID")]
[PXSelector(typeof(Search<InventoryItem.inventoryID, Where<Match<Current<AccessInfo.userName>>>>), typeof(InventoryItem.inventoryCD), typeof(InventoryItem.descr))]
public virtual int? InventoryID { get; set; }
public abstract class inventoryID : PX.Data.BQL.BqlInt.Field<inventoryID> { }
#endregion
#region InventoryDescr
[PXString(256, IsUnicode = true, InputMask = "")]
[PXUIField(DisplayName = "Inventory Descr")]
public virtual string InventoryDescr { get; set; }
public abstract class inventoryDescr : PX.Data.BQL.BqlString.Field<inventoryDescr> { }
#endregion
#region NonConfReason
[PXString(IsUnicode = true, InputMask = "")]
[PXUIField(DisplayName = "Non Conf Reason")]
[PXStringList(new string[] { "ARRIDAM", "WRPARREC", "INCQTY", "PORCOS", "ARROUTLE", "INCSOFT", "INCLABEL", "WROLOC", "DOA" },
new string[] { "Arrived damaged", " Wrong Part Received", "Incorrect Quantity", "Poor Cosmetics", "Arrived Outside Lead Time", "Incorrect Software", "Incorrect Labeling", "Wrong Lcation", "DOA" })]
public virtual string NonConfReason { get; set; }
public abstract class nonConfReason : PX.Data.BQL.BqlString.Field<nonConfReason> { }
#endregion
#region Resolution
[PXString(IsUnicode = true, InputMask = "")]
[PXUIField(DisplayName = "Resolution")]
public virtual string Resolution { get; set; }
public abstract class resolution : PX.Data.BQL.BqlString.Field<resolution> { }
#endregion
#region NCNotes
[PXString(IsUnicode = true, InputMask = "")]
[PXUIField(DisplayName = "Notes")]
public virtual string NCNotes { get; set; }
public abstract class nCNotes : PX.Data.BQL.BqlString.Field<nCNotes> { }
#endregion
}
}
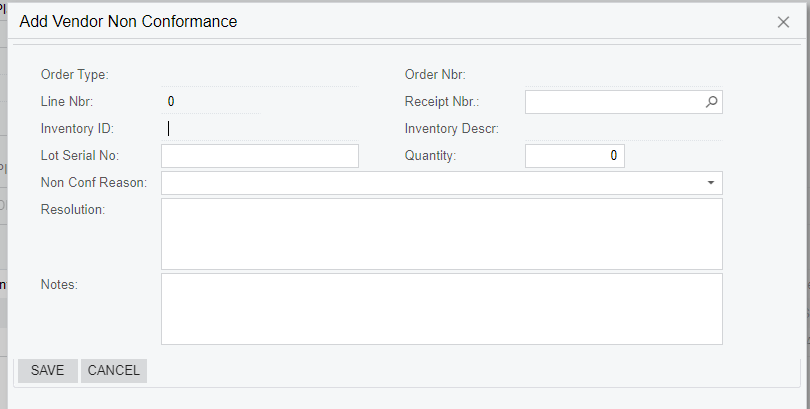
Best answer by scottstanaland12
View original