Hi, I’m creating the following records via the appropriate graphs: purchase orders, sales orders, and purchase requisitions. But things are not working as I hoped/expected. I thought the idea of using graphs to create records was that you would benefit from features added to the graph, for example if you select a customer, the graph would automatically set the currency for you. This doesn’t seem to be happening consistently and I’m wondering if I’ve misunderstood something fundamental to Acumatica development.
My test system has a base currency for GBP
First, I created a test customer called CUST001 and a test vendor VEND001. These both use USD currency.
Next, I created records in the Acumatica UI.
For a sales order I enter the customer and press save. The location and currency have been defaulted. The purchase is the same, except I use VEND001.
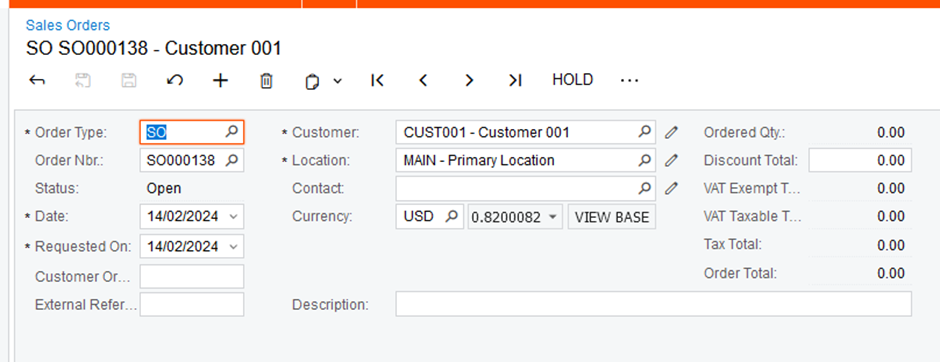
The purchase requisition follows the same idea. I enter the customer as CUST001 and the currency is automatically set to USD.
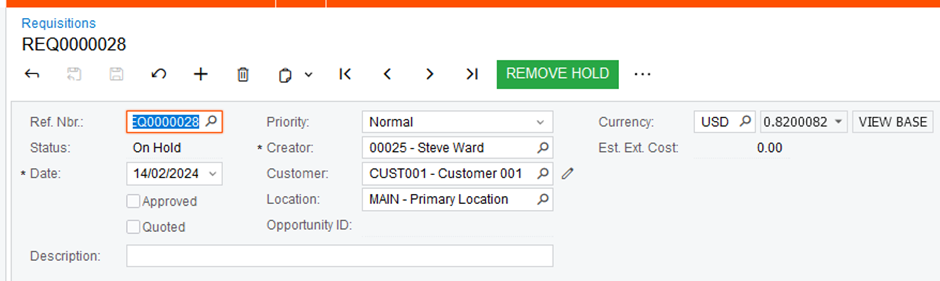
Finally, I’ve used the code below to create records from code. I believe the code is matching the actions from the UI. I set the customer/vendor, and save the record.
private void CreateTestRecords()
{
//CREATE PURCHASE ORDER
BAccount bAccount1 = Base.Select<BAccount>().FirstOrDefault(x => x.AcctCD == "VEND001");
POOrderEntry pOOrderEntry = PXGraph.CreateInstance<POOrderEntry>();
POOrder pOOrder = (POOrder)pOOrderEntry.Document.Cache.CreateInstance();
pOOrder.VendorID = bAccount1.BAccountID;
pOOrder = pOOrderEntry.Document.Insert(pOOrder);
try
{
pOOrderEntry.Actions.PressSave();
}
catch (Exception ex)
{
string s = ex.Message;
}
//CREATE SALES ORDER
BAccount bAccount2 = Base.Select<BAccount>().FirstOrDefault(x => x.AcctCD == "CUST001");
SOOrderEntry sOOrderEntry = PXGraph.CreateInstance<SOOrderEntry>();
SOOrder sOOrder = (SOOrder)sOOrderEntry.Document.Cache.CreateInstance();
sOOrder.CustomerID = bAccount2.BAccountID;
sOOrder = sOOrderEntry.Document.Insert(sOOrder);
try
{
sOOrderEntry.Actions.PressSave();
}
catch (Exception ex)
{
string s = ex.Message;
}
//CREATE PO REQUISITION
BAccount bAccount3 = Base.Select<BAccount>().FirstOrDefault(x => x.AcctCD == "CUST001");
RQRequisitionEntry rQRequisitionEntry = PXGraph.CreateInstance<RQRequisitionEntry>();
RQRequisition rQRequisition = (RQRequisition)rQRequisitionEntry.Document.Cache.CreateInstance();
rQRequisition.CustomerID = bAccount3.BAccountID;
rQRequisition = rQRequisitionEntry.Document.Insert(rQRequisition);
try
{
rQRequisitionEntry.Actions.PressSave();
}
catch (Exception ex)
{
string s = ex.Message;
}
}
The results from the code don’t match the results from the UI, and I don’t understand why.
On the purchase order the CuryID has been set to GBP (not USD like when using the UI), when Save is pressed an exception is raised saying "The currency 'USD' of the pay-to Supplier 'VEND001' differs from currency 'GBP' of the document."
The sales order is created just the UI version, the currency is set to USD as expected.
The requisition is created but the currency is GBP, not USD. See below.
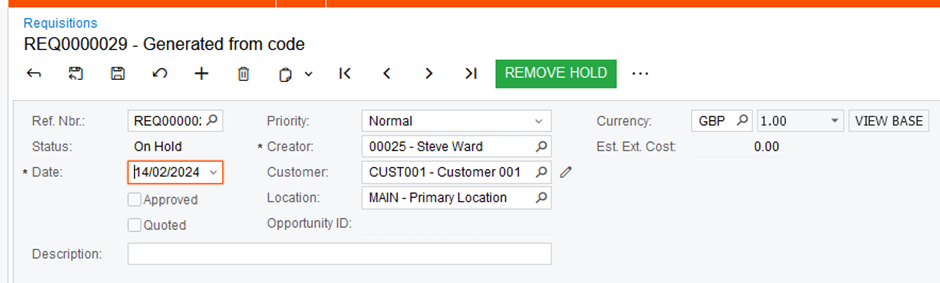
Can anyone explain why, on the purchase order and the requisition the currency isn’t being set to the customer/vendor currency?
Of course I could update the currency in the code but I thought the reason we create records via graphs was to take advantage of the features and validation built into the graphs. Have I misunderstood something?
Any comments/theories/explanations would be welcome.