The issue is that when changing a field, Acumatica pulls null values. Some fields retrieve values correctly, but others, such as BranchID, do not. At the same time, and perhaps related to my issue, the amount fields like January, February, etc., have commitChanges, and when I alter one of these fields, the others are set to 0.
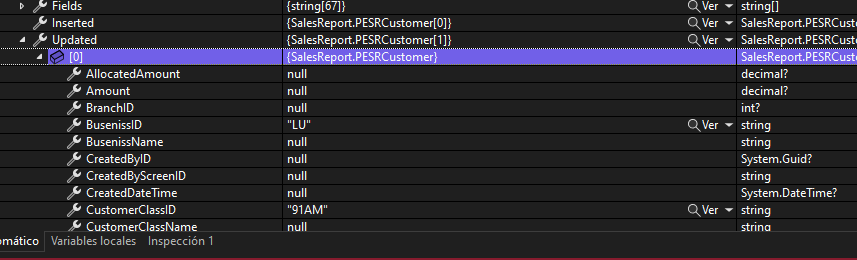
This is my code:
using System;
using System.Runtime.CompilerServices;
using PX.Common;
using PX.CS;
using PX.Data;
using PX.Data.BQL;
using PX.Data.BQL.Fluent;
using PX.Data.Maintenance.GI;
using PX.Data.ReferentialIntegrity.Attributes;
using PX.Objects.AR;
using PX.Objects.CS;
using PX.Objects.GL;
using PX.Objects.IN;
using static PX.SM.RowNewFile;
using CSAttribute = PX.CS.CSAttribute;
using CSAttributeDetail = PX.CS.CSAttributeDetail;
namespace SalesReport
{
public class BudgetCustomer : PXGraph<BudgetCustomer>
{
public PXCancel<BudgetFilter> Cancel;
public PXSave<BudgetFilter> Save;
public PXFilter<BudgetFilter> Filter;
public SelectFrom<PESRCustomer>
.Where<
PESRCustomer.branchID.IsEqual<BudgetFilter.branchID.FromCurrent>
.And<PESRCustomer.ledgerID.IsEqual<BudgetFilter.ledgerID.FromCurrent>>
.And<PESRCustomer.finYear.IsEqual<BudgetFilter.finYear.FromCurrent>>
>
.View Budget;
protected void PESRCustomer_CustomerID_FieldUpdated(PXCache cache, PXFieldUpdatedEventArgs e)
{
PESRCustomer miRegistro = (PESRCustomer)e.Row;
UpdateName(4, miRegistro);
}
protected virtual void _(Events.FieldUpdated<PESRCustomer, PESRCustomer.customerClassID> e)
{
PESRCustomer miRegistro = e.Row;
UpdateName(3, miRegistro);
}
protected virtual void _(Events.FieldUpdated<PESRCustomer, PESRCustomer.busenissID> e)
{
PESRCustomer miRegistro = e.Row;
UpdateName(1, miRegistro);
}
protected virtual void _(Events.FieldUpdated<PESRCustomer, PESRCustomer.productClassID> e)
{
PESRCustomer miRegistro = e.Row;
UpdateName(2, miRegistro);
}
protected virtual void _(Events.RowSelected<PESRCustomer> e)
{
PESRCustomer miRegistro = e.Row;
UpdateName(1, miRegistro);
UpdateName(2, miRegistro);
UpdateName(3, miRegistro);
UpdateName(4, miRegistro);
}
protected void UpdateName(int Indices, PESRCustomer miRegistro)
{
switch (Indices)
{
case 1:
if (miRegistro != null)
{
CSAttributeDetail busniss =
SelectFrom<CSAttributeDetail>
.Where<CSAttributeDetail.attributeID.IsEqual<@P.AsString>
.And<CSAttributeDetail.valueID.IsEqual<@P.AsString>>
>.View.Select(this, "SRBUSINESS", miRegistro.BusenissID);
if (busniss != null)
{
miRegistro.BusenissName = busniss.Description;
}
}
break;
case 2:
if (miRegistro != null)
{
INItemClass itemclasses = SelectFrom<INItemClass>.Where<INItemClass.itemClassID.IsEqual<@P.AsInt>>.View.Select(this, miRegistro.ProductClassID);
if (itemclasses != null)
{
miRegistro.ProductClassName = itemclasses.Descr;
}
}
break;
case 3:
if (miRegistro != null)
{
CustomerClass customerclassDescripcion = SelectFrom<CustomerClass>.Where<CustomerClass.customerClassID.IsEqual<@P.AsString>>.View.Select(this, miRegistro.CustomerClassID);
if (customerclassDescripcion != null)
{
miRegistro.CustomerClassName = customerclassDescripcion.Descr;
}
}
break;
case 4:
if (miRegistro != null)
{
Customer customer = PXSelect<Customer, Where<Customer.bAccountID, Equal<Required<Customer.bAccountID>>>>.Select(this, miRegistro.CustomerID);
if (customer != null)
{
miRegistro.CustomerName = customer.AcctName;
}
}
break;
}
}
}
#region PESRCustomer
[Serializable]
[PXCacheName("PESRCustomer")]
public class PESRCustomer : IBqlTable
{
#region Constantes
public static class SRBUSINESSConstants
{
public const string SRBusiness = "SRBUSINESS";
}
public class srbusiness : PX.Data.BQL.BqlString.Constant<srbusiness>
{
public srbusiness() : base(SRBUSINESSConstants.SRBusiness) { }
}
#endregion
#region BranchID
[Branch(typeof(BudgetFilter.branchID))]
public virtual int? BranchID { get; set; }
public abstract class branchID : PX.Data.BQL.BqlInt.Field<branchID> { }
#endregion
#region LedgerID
[PXDBInt(IsKey = true)]
[PXDefault(typeof(BudgetFilter.ledgerID))]
[PXUIField(Enabled = false, Visible = false)]//, Visible = false, Visibility = PXUIVisibility.Invisible
[PXSelector(typeof(Ledger.ledgerID), SubstituteKey = typeof(Ledger.ledgerCD))]
public virtual int? LedgerID { get; set; }
public abstract class ledgerID : PX.Data.BQL.BqlInt.Field<ledgerID> { }
#endregion
#region FinYear
[PXDBString(4, IsKey = true, IsFixed = true, InputMask = "")]
[PXDefault(typeof(BudgetFilter.finYear))]
[PXUIField(DisplayName = "Fin Year", Visible = false)]
public virtual string FinYear { get; set; }
public abstract class finYear : PX.Data.BQL.BqlString.Field<finYear> { }
#endregion
#region BusenissID
[PXDBString(9, IsKey = true)]
[PXUIField(DisplayName = "Business")]
[PXSelector(typeof(Search5<CSAttributeDetail.valueID,
InnerJoin<CSAttribute, On<CSAttribute.attributeID, Equal<CSAttributeDetail.attributeID>>>,
Where<CSAttribute.attributeID, Equal<srbusiness>>,
Aggregate<GroupBy<CSAttributeDetail.valueID>>,
OrderBy<Asc<CSAttributeDetail.valueID>>
>),
typeof(CSAttributeDetail.valueID), typeof(CSAttributeDetail.description),
DescriptionField = typeof(CSAttributeDetail.description)
)]
public virtual string BusenissID { get; set; }
public abstract class busenissID : PX.Data.BQL.BqlString.Field<busenissID> { }
#endregion
#region BusenissName
[PXString(250)]
[PXUIField(DisplayName = "Buseniss Name", IsReadOnly = true)]
public virtual string BusenissName { get; set; }
public abstract class busenissName : PX.Data.BQL.BqlString.Field<busenissName> { }
#endregion
#region ProductClassID
[PXDBInt(IsKey = true)]
[PXUIField(DisplayName = "Item Class ID", Visibility = PXUIVisibility.SelectorVisible)]
[PXSelector(typeof(Search<INItemClass.itemClassID>),
SubstituteKey = typeof(INItemClass.itemClassCD),
DescriptionField = typeof(INItemClass.descr), ValidateValue = false)]
public virtual int? ProductClassID { get; set; }
public abstract class productClassID : PX.Data.BQL.BqlInt.Field<productClassID> { }
#endregion
#region ProductClassName
[PXString(250)]
[PXUIField(DisplayName = "Item Class Name", IsReadOnly = true)]
public virtual string ProductClassName { get; set; }
public abstract class productClassName : PX.Data.BQL.BqlString.Field<productClassName> { }
#endregion
#region CustomerClassID
[PXDBString(18, IsKey = true, IsUnicode = true)]
[PXSelector(typeof(Search<CustomerClass.customerClassID>),
typeof(CustomerClass.customerClassID),
SubstituteKey = typeof(CustomerClass.customerClassID),
DescriptionField = typeof(CustomerClass.descr))]
[PXUIField(DisplayName = "Customer Class ID", Visibility = PXUIVisibility.SelectorVisible)]
public virtual string CustomerClassID { get; set; }
public abstract class customerClassID : PX.Data.BQL.BqlString.Field<customerClassID> { }
#endregion
#region CustomerClassName
[PXString(250)]
[PXUIField(DisplayName = "Customer Class Name", IsReadOnly = true)]
public virtual string CustomerClassName { get; set; }
public abstract class customerClassName : PX.Data.BQL.BqlString.Field<customerClassName> { }
#endregion
#region CustomerID
[PXDBInt(IsKey = true)]
[PXSelector(typeof(Customer.bAccountID), typeof(Customer.acctCD), typeof(Customer.acctName),
SubstituteKey = typeof(Customer.acctCD),
DescriptionField = typeof(Customer.acctName))]
[PXUIField(DisplayName = "Customer ID")]
public virtual int? CustomerID { get; set; }
public abstract class customerID : PX.Data.BQL.BqlInt.Field<customerID> { }
#endregion
#region CustomerName
[PXString(250)]
[PXUIField(DisplayName = "Customer Name", IsReadOnly = true)]
public virtual string CustomerName { get; set; }
public abstract class customerName : PX.Data.BQL.BqlString.Field<customerName> { }
#endregion
#region Amount
[PXDBDecimal()]
[PXUIField(DisplayName = "Amount")]
[PXDefault(TypeCode.Decimal, "0.0")]
public virtual Decimal? Amount { get; set; }
public abstract class amount : PX.Data.BQL.BqlDecimal.Field<amount> { }
#endregion
#region AllocatedAmount
[PXDBDecimal()]
[PXUIField(DisplayName = "Allocated Amount", Enabled = false)]
[PXDefault(TypeCode.Decimal, "0.0")]
[PXFormula(typeof(Add<finPeriod01, Add<finPeriod02, Add<finPeriod03, Add<finPeriod04, Add<finPeriod05, Add<finPeriod06, Add<finPeriod07, Add<finPeriod08, Add<finPeriod09, Add<finPeriod10, Add<finPeriod11, Add<finPeriod12, finPeriod13>>>>>>>>>>>>))]
public virtual Decimal? AllocatedAmount { get; set; }
public abstract class allocatedAmount : PX.Data.BQL.BqlDecimal.Field<allocatedAmount> { }
#endregion
#region FinPeriod01
[PXDBDecimal()]
[PXUIField(DisplayName = "January")]
[PXDefault(TypeCode.Decimal, "0.0")]
public virtual Decimal? FinPeriod01 { get; set; }
public abstract class finPeriod01 : PX.Data.BQL.BqlDecimal.Field<finPeriod01> { }
#endregion
#region FinPeriod02
[PXDBDecimal()]
[PXUIField(DisplayName = "February")]
[PXDefault(TypeCode.Decimal, "0.0")]
public virtual Decimal? FinPeriod02 { get; set; }
public abstract class finPeriod02 : PX.Data.BQL.BqlDecimal.Field<finPeriod02> { }
#endregion
#region FinPeriod03
[PXDBDecimal()]
[PXUIField(DisplayName = "March")]
[PXDefault(TypeCode.Decimal, "0.0")]
public virtual Decimal? FinPeriod03 { get; set; }
public abstract class finPeriod03 : PX.Data.BQL.BqlDecimal.Field<finPeriod03> { }
#endregion
#region FinPeriod04
[PXDBDecimal()]
[PXUIField(DisplayName = "April")]
[PXDefault(TypeCode.Decimal, "0.0")]
public virtual Decimal? FinPeriod04 { get; set; }
public abstract class finPeriod04 : PX.Data.BQL.BqlDecimal.Field<finPeriod04> { }
#endregion
#region FinPeriod05
[PXDBDecimal()]
[PXUIField(DisplayName = "May")]
[PXDefault(TypeCode.Decimal, "0.0")]
public virtual Decimal? FinPeriod05 { get; set; }
public abstract class finPeriod05 : PX.Data.BQL.BqlDecimal.Field<finPeriod05> { }
#endregion
#region FinPeriod06
[PXDBDecimal()]
[PXUIField(DisplayName = "June")]
[PXDefault(TypeCode.Decimal, "0.0")]
public virtual Decimal? FinPeriod06 { get; set; }
public abstract class finPeriod06 : PX.Data.BQL.BqlDecimal.Field<finPeriod06> { }
#endregion
#region FinPeriod07
[PXDBDecimal()]
[PXUIField(DisplayName = "July")]
[PXDefault(TypeCode.Decimal, "0.0")]
public virtual Decimal? FinPeriod07 { get; set; }
public abstract class finPeriod07 : PX.Data.BQL.BqlDecimal.Field<finPeriod07> { }
#endregion
#region FinPeriod08
[PXDBDecimal()]
[PXUIField(DisplayName = "August")]
[PXDefault(TypeCode.Decimal, "0.0")]
public virtual Decimal? FinPeriod08 { get; set; }
public abstract class finPeriod08 : PX.Data.BQL.BqlDecimal.Field<finPeriod08> { }
#endregion
#region FinPeriod09
[PXDBDecimal()]
[PXUIField(DisplayName = "September")]
[PXDefault(TypeCode.Decimal, "0.0")]
public virtual Decimal? FinPeriod09 { get; set; }
public abstract class finPeriod09 : PX.Data.BQL.BqlDecimal.Field<finPeriod09> { }
#endregion
#region FinPeriod10
[PXDBDecimal()]
[PXUIField(DisplayName = "October")]
[PXDefault(TypeCode.Decimal, "0.0")]
public virtual Decimal? FinPeriod10 { get; set; }
public abstract class finPeriod10 : PX.Data.BQL.BqlDecimal.Field<finPeriod10> { }
#endregion
#region FinPeriod11
[PXDBDecimal()]
[PXUIField(DisplayName = "November")]
[PXDefault(TypeCode.Decimal, "0.0")]
public virtual Decimal? FinPeriod11 { get; set; }
public abstract class finPeriod11 : PX.Data.BQL.BqlDecimal.Field<finPeriod11> { }
#endregion
#region FinPeriod12
[PXDBDecimal()]
[PXUIField(DisplayName = "December")]
[PXDefault(TypeCode.Decimal, "0.0")]
public virtual Decimal? FinPeriod12 { get; set; }
public abstract class finPeriod12 : PX.Data.BQL.BqlDecimal.Field<finPeriod12> { }
#endregion
#region FinPeriod13
[PXDBDecimal()]
[PXUIField(DisplayName = "Fin Period13")]
[PXDefault(TypeCode.Decimal, "0.0")]
public virtual Decimal? FinPeriod13 { get; set; }
public abstract class finPeriod13 : PX.Data.BQL.BqlDecimal.Field<finPeriod13> { }
#endregion
#region CreatedByID
[PXDBCreatedByID()]
public virtual Guid? CreatedByID { get; set; }
public abstract class createdByID : PX.Data.BQL.BqlGuid.Field<createdByID> { }
#endregion
#region CreatedByScreenID
[PXDBCreatedByScreenID()]
public virtual string CreatedByScreenID { get; set; }
public abstract class createdByScreenID : PX.Data.BQL.BqlString.Field<createdByScreenID> { }
#endregion
#region CreatedDateTime
[PXDBCreatedDateTime()]
public virtual DateTime? CreatedDateTime { get; set; }
public abstract class createdDateTime : PX.Data.BQL.BqlDateTime.Field<createdDateTime> { }
#endregion
#region LastModifiedByID
[PXDBLastModifiedByID()]
public virtual Guid? LastModifiedByID { get; set; }
public abstract class lastModifiedByID : PX.Data.BQL.BqlGuid.Field<lastModifiedByID> { }
#endregion
#region LastModifiedByScreenID
[PXDBLastModifiedByScreenID()]
public virtual string LastModifiedByScreenID { get; set; }
public abstract class lastModifiedByScreenID : PX.Data.BQL.BqlString.Field<lastModifiedByScreenID> { }
#endregion
#region LastModifiedDateTime
[PXDBLastModifiedDateTime()]
public virtual DateTime? LastModifiedDateTime { get; set; }
public abstract class lastModifiedDateTime : PX.Data.BQL.BqlDateTime.Field<lastModifiedDateTime> { }
#endregion
#region Tstamp
[PXDBTimestamp()]
[PXUIField(DisplayName = "Tstamp")]
public virtual byte[] Tstamp { get; set; }
public abstract class tstamp : PX.Data.BQL.BqlByteArray.Field<tstamp> { }
#endregion
#region Noteid
[PXNote()]
public virtual Guid? Noteid { get; set; }
public abstract class noteid : PX.Data.BQL.BqlGuid.Field<noteid> { }
#endregion
}
#endregion
}
Build 21.214.0034
Visual Studio 2022
You're welcome! Thank you for your attention.