Hi,
I want to execute a custom logic when the user click the Release button, but only if the Release process completes successfully. How I can get the error message from the release process? This is my code.
[PXOverride]
public IEnumerable Release(PXAdapter adapter, ReleaseDelegate baseMethod)
{
baseMethod(adapter);
_inservice.UpdateItemLocation(Base, itemloc.Cache, AddOrSubstract.Add, Base.transactions.Cache);
Base.Save.PressButton();
return adapter.Get();
}
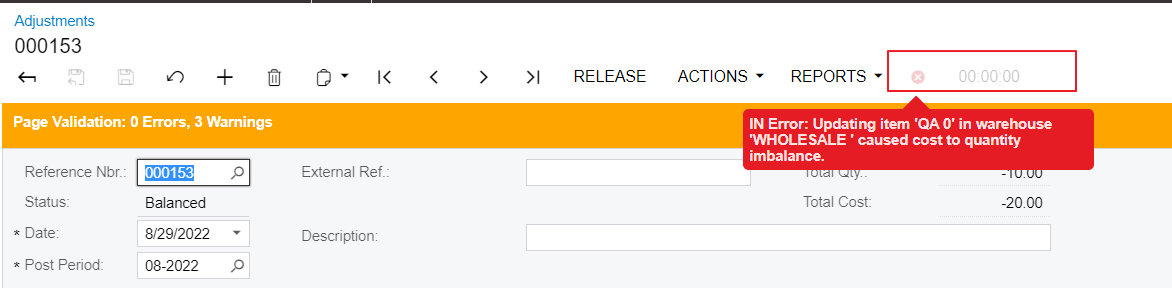
Thanks,
EV
Best answer by edsonvelez64
View original