This is an extension of a thread:
How can I change header value of a field based on currently selected row in the grid.
So far, I have found a working solution. However, there's a minor issue: the UsrWorkCenterID field (show details...) doesn't update automatically. I need to clear the current record to obtain the new value. If anyone knows how to resolve this minor issue, please share the solution.
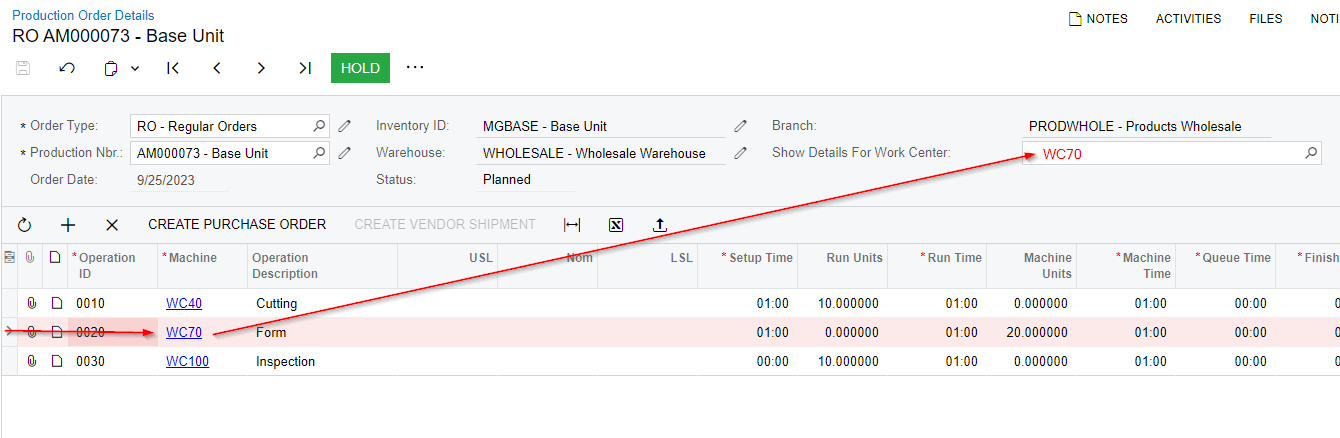
protected void AMProdItem_UsrWorkCenterID_FieldSelecting(PXCache cache, PXFieldSelectingEventArgs e)
{
// Getting the production item data.
var prodItemRow = (AMProdItem)e.Row;
if (prodItemRow == null) return; // If there's no data, return.
// Trying to find the current operation info.
var currentOperRow = Base.Caches<AMProdOper>().Current as AMProdOper;
// If the current operation matches the item's details, use its Work Center ID.
if (currentOperRow != null && currentOperRow.ProdOrdID == prodItemRow.ProdOrdID)
{
e.ReturnValue = currentOperRow.WcID;
}
else
{
// If not, look for related operations to find a Work Center ID.
var relatedOper = PXSelect<AMProdOper,
Where<AMProdOper.orderType, Equal<Required<AMProdOper.orderType>>,
And<AMProdOper.prodOrdID, Equal<Required<AMProdOper.prodOrdID>>>>
>.SelectWindowed(Base, 0, 1, prodItemRow.OrderType, prodItemRow.ProdOrdID);
// If a related operation is found, set the return value to its Work Center ID.
if (relatedOper != null && relatedOper.Count > 0)
{
e.ReturnValue = ((AMProdOper)relatedOper).WcID;
}
}
}
#region UsrWorkCenterID
[PXString(20)]
[PXSelector(typeof(Search<AMProdOper.wcID,
Where<AMProdOper.orderType, Equal<Current<AMProdItem.orderType>>,
And<AMProdOper.prodOrdID, Equal<Current<AMProdItem.prodOrdID>>>>>))]
[PXUIField(DisplayName = "Show Details For Work Center")]
public virtual string UsrWorkCenterID { get; set; }
public abstract class usrWorkCenterID : PX.Data.BQL.BqlString.Field<usrWorkCenterID> { }