I have a processing page to “mass delete” empty customer records. On the processing dialog, it shows the Remaining count as the total count of the records being processed until ALL items have been completed. Then, the Processed count shows all 3 items processed successfully after they are ALL complete.
I’m being a bit picky here, but I want the Processed count incremented and the Remaining count decremented after each record is processed.
When you click the PROCESS ALL button, this is where it starts out
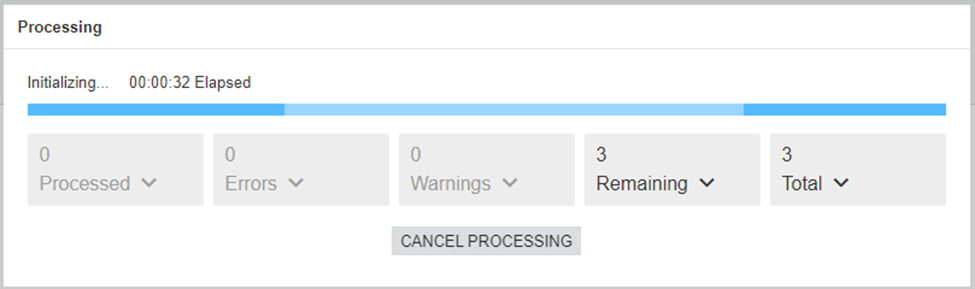
After processing is complete for all 3 records, it shows this:
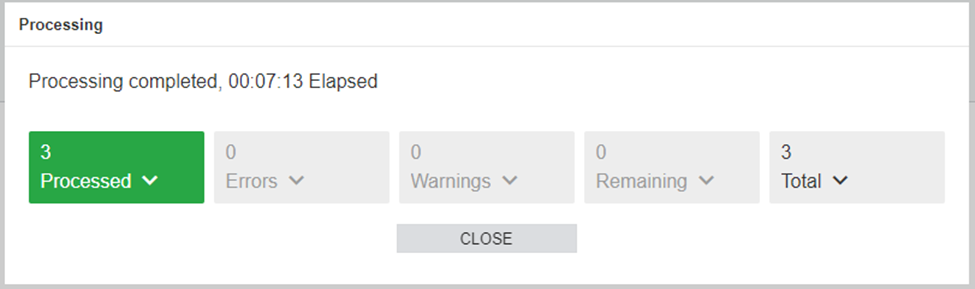
This is an example of my code:
public static void DoIt(List<ICSMCCustomersToProcess> custList, bool? deleteCustomer)
{
foreach (ICSMCCustomersToProcess cust in custList)
{
try
{
bool? customerNotDeleted;
string errorMessage;
var graph = PXGraph.CreateInstance<DeleteCustomers>();
graph.Clear();
graph.DeleteCustomer(cust, out customerNotDeleted, out errorMessage, deleteCustomer, true);
//default
PXProcessing<ICSMCCustomersToDelete>.SetProcessed();
//override 1
if (customerNotDeleted == true)
{
PXProcessing<ICSMCCustomersToDelete>.SetWarning(custList.IndexOf(cust), "Customer was unable to be deleted");
}
//override 2
if (errorMessage != "")
{
PXProcessing<ICSMCCustomersToDelete>.SetError(custList.IndexOf(cust), errorMessage);
}
}
catch (Exception e)
{
PXProcessing<ICSMCCustomersToDelete>.SetError(custList.IndexOf(cust), e);
}
}
}
I added PXProcessing<ICSMCCustomersToDelete>.SetProcessed(); right after the DeleteCustomer method is called.
Now, after the first record is processed, the counters are updated:
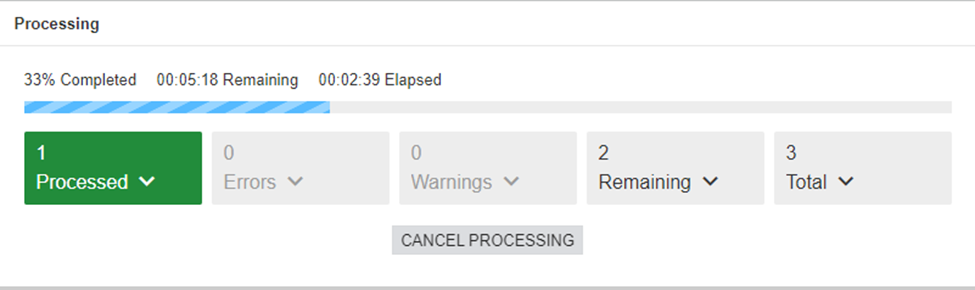
However, the remaining two records are both processed together and the Processed counter is not being updated the way it was for the first record.
After the remaining 2 records are processed, it goes straight to this:
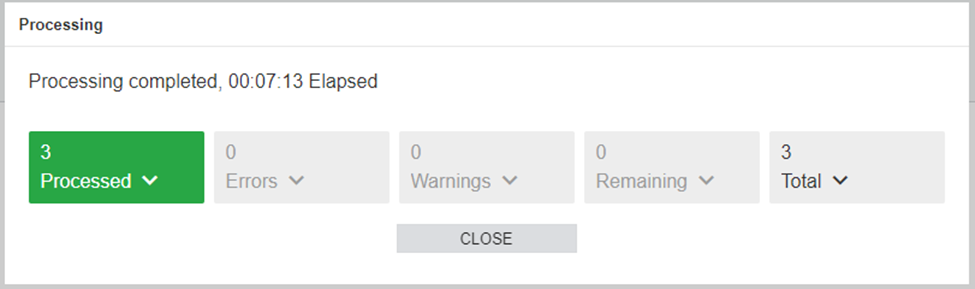
In testing, if I force one of the records to fail to be deleted, this code will set a warning and the Warnings count will be updated. In this line, there is a parameter to specify which record is being set to a warning.
PXProcessing<ICSMCCustomersToDelete>.SetWarning(custList.IndexOf(cust), "Customer was unable to be deleted");
However, PXProcessing<ICSMCCustomersToMerge>.SetProcessed(); does not offer a parameter so I thought maybe that was the issue.
Any ideas on how to force the completed records to move to the Processed counter?
Best answer by Leonardo Justiniano
View original