I created an action button to create a service order from the customer screen. The customization works great. The only problem is the Address Lookup seemed to stop working. Any Idea what may be causing this?
using PX.Data;
using PX.Objects.CR;
using PX.Objects.FS;
using System.Collections;
using PX.SM;
namespace PX.Objects.AR
{
public class CustomerMaint_Extension : PXGraphExtension<PX.Objects.AR.CustomerMaint>
{
public class CustomerServiceOrderDac : IBqlTable{
[PXString(4, IsFixed = true, InputMask = ">AAAA")]
[PXUIField(DisplayName = "Service Order Type", Required = true)]
[PXDefault(PersistingCheck = PXPersistingCheck.NullOrBlank)]
[FSSelectorActiveSrvOrdType]
public virtual string ServiceOrderType{ get; set; }
public abstract class serviceOrderType : PX.Data.BQL.BqlString.Field<serviceOrderType> { }
}
public PXFilter<CustomerServiceOrderDac> pXFilter;
public PXAction<Customer> CreateServiceOrderAction;
[PXButton(CommitChanges = true, DisplayOnMainToolbar = true, Category = "Actions")]
[PXUIField(DisplayName = "Create Service Order", MapViewRights = PXCacheRights.Select, MapEnableRights = PXCacheRights.Update)]
protected virtual IEnumerable createServiceOrderAction(PXAdapter adapter) {
WebDialogResult result = pXFilter.AskExt();
if (result == WebDialogResult.OK){
Customer customer = Base.BAccount.Current;
if (customer == null) return adapter.Get();
var Filter = pXFilter.Current;
if (Filter == null) return adapter.Get();
var serviceOrderEntry = PXGraph.CreateInstance<ServiceOrderEntry>(); //Field Service Graph
if (serviceOrderEntry == null) return adapter.Get();
FSServiceOrder fsServiceOrder = new FSServiceOrder()
{
CustomerID = Base.BAccount.Current.BAccountID,
SrvOrdType = Filter.ServiceOrderType
};
if (fsServiceOrder.SrvOrdType == null)
{
const string ErrorMessage = "Service Order cant be blank";
throw new PXSetPropertyException(ErrorMessage, PXErrorLevel.Error);
}
PXLongOperation.StartOperation(Base, delegate ()
{
fsServiceOrder = serviceOrderEntry.ServiceOrderRecords.Insert(fsServiceOrder);
throw new PXRedirectRequiredException(serviceOrderEntry, null);
});
}
return adapter.Get();
}
public override void Initialize()
{
base.Initialize();
Base.action.AddMenuAction(CreateServiceOrderAction);
}
}
}
the address lookup
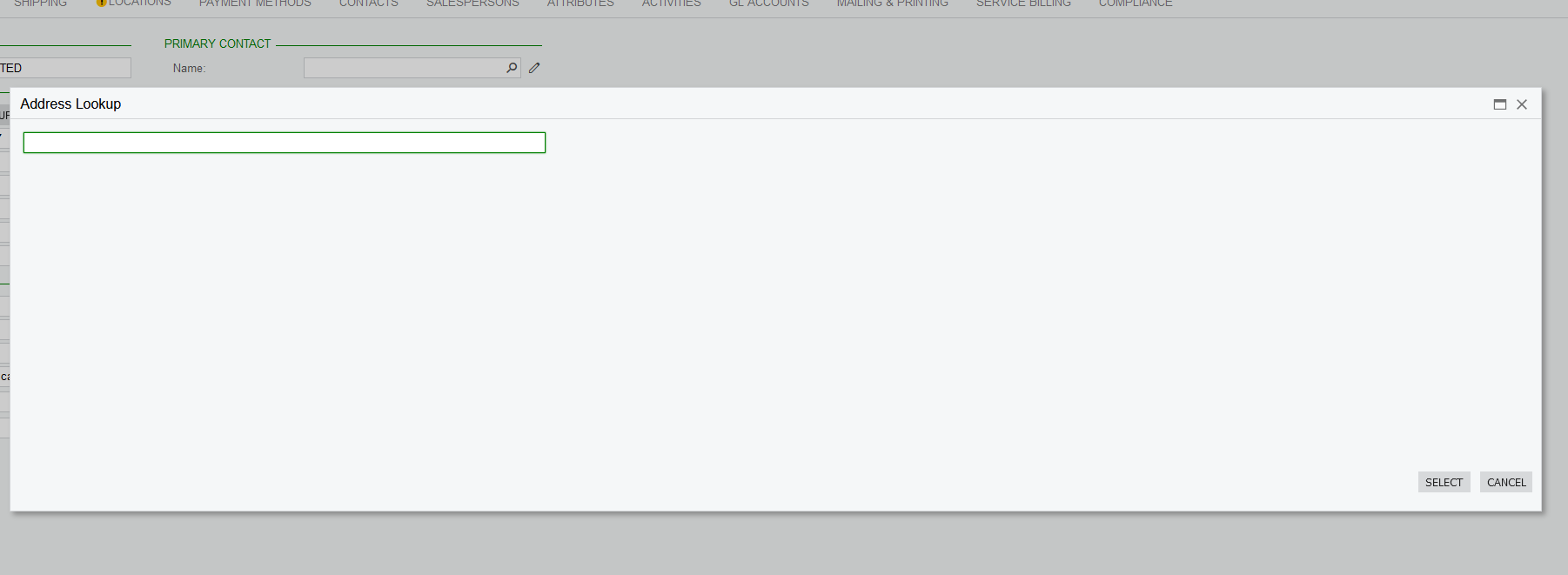