I have a custom processing screen with two filter fields.
The filter for the screen id works. The filter for the file extension does not.
Ignore the first filter field. I was trying to use a selector to get UploadAllowableFileExtensions, but the value returned includes a “.” in front of the extension. So I created a DDL with just the last three characters of the extension. I could not figure a way to trim off the “.” in the value of the selector.
When just the screen id is provided, it shows three files for that screen:
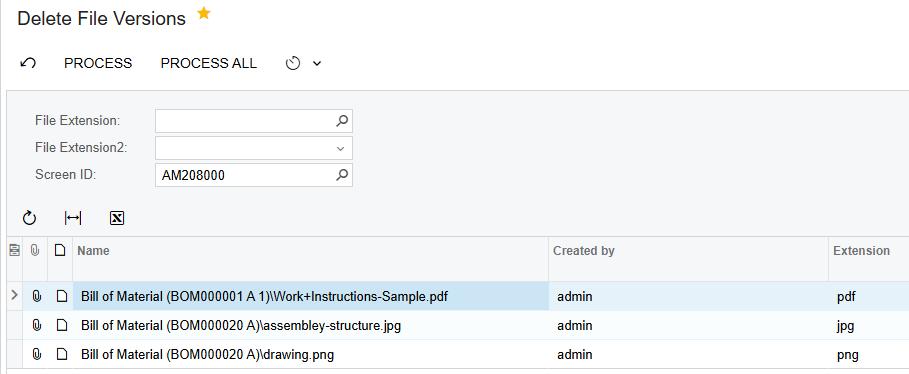
As soon as I choose PDF from the File Extension2 DDL, the view is refreshed and attempts to apply the file extension filter.
Here is the code for the applyfilters method:
protected virtual void ApplyFilters(PXSelectBase<UploadFile> shCmd, RecordsToProcessFilter filter)
{
if (filter.ScreenID != null)
shCmd.WhereAnd<Where<UploadFile.primaryScreenID.IsEqual<RecordsToProcessFilter.screenID.FromCurrent>>>();
if (filter.FileExtension2 != null)
{
//returns extansion = 'pdf'
UploadFile test = SelectFrom<UploadFile>.Where<UploadFile.primaryScreenID.IsEqual<@P.AsString>
.And<UploadFile.isSystem.IsEqual<False>>>.View.Select(this, "AM208000").FirstOrDefault();
//returns null
//using FirstOrDefault throws an error so I removed it
UploadFile test2 = SelectFrom<UploadFile>.Where<UploadFile.primaryScreenID.IsEqual<@P.AsString>
.And<UploadFile.extansion.IsEqual<@P.AsString>>
.And<UploadFile.isSystem.IsEqual<False>>>.View.Select(this, "AM208000", "pdf");
shCmd.WhereAnd<Where<UploadFile.extansion.IsEqual<RecordsToProcessFilter.fileExtension2.FromCurrent>>>();
}
}
The WhereAnd for the filter.FileExtension2 is intended to only show files from UploadFiles table where the “extansion” =”pdf”.
In debug, I did 2 manual selects to get a record that is being displayed in my grid. The first select returns a record in the grid and the value in the UploadFile.extansion is “pdf”.
If I hard code “pdf” in the second test, the record is null.
Note, “extansion” is not a typo in the code. That is the DAC field name.
Obviously, if my test2 select returns null, the whereand is going to cause a null result set.
To prove that the value for extansion in test1 is pdf, here is a screen shot:
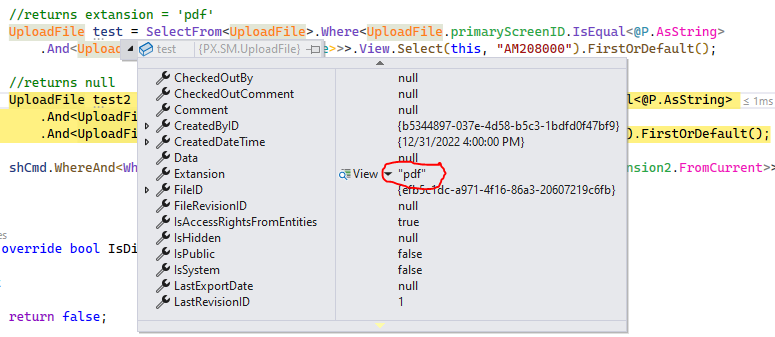
The FileExtension2 value in my Filter is also “pdf”
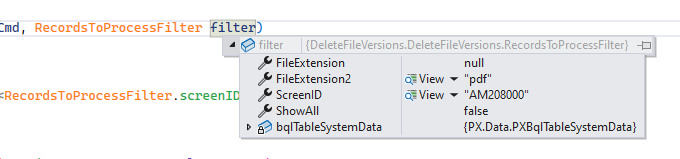
test2 returns null:

The real issue is why can’t I return a record in test2? If I can resolve that, the filter will probably work just fine.
Now that I have submitted this, I will probably figure it out in the next 3 minutes. 😏