Hello,
I can update an inventory item with the code below. I’m not suggesting it’s the best way but it works, the description is updated and saved to the database.
public SelectFrom<InventoryItem>.View InventoryItemView;
public PXAction<InventoryItem> UpdateItem;
public virtual IEnumerable updateItem(PXAdapter adapter)
{
List<InventoryItem> l = InventoryItemView.Select().RowCast<InventoryItem>().ToList<InventoryItem>();
InventoryItemView.Current = l.First();
InventoryItemView.Current.Descr = String.Concat(InventoryItemView.Current.Descr, "X");
InventoryItemView.UpdateCurrent();
InventoryItemView.Cache.Persist(PXDBOperation.Update);
return adapter.Get();
}
Using the same method I can update a sales order line.
public SelectFrom<SOLine>.View SOLineUpdateView;
public PXAction<InventoryItem> UpdateSOLine;
public virtual IEnumerable updateSOLine(PXAdapter adapter)
{
List<SOLine> l = SOLineUpdateView.Select().RowCast<SOLine>().ToList<SOLine>();
SOLineUpdateView.Current = l.First();
SOLineUpdateView.Current.TranDesc = String.Concat(SOLineUpdateView.Current.TranDesc, "X");
SOLineUpdateView.UpdateCurrent();
SOLineUpdateView.Cache.Persist(PXDBOperation.Update);
return adapter.Get();
}
I’ve used similar code to update records for SOOrder, POOrder and ItemClass too, and they all update without any issue.
BUT, if I use the same method on purchase order lines I get an error. Here is the code.
public SelectFrom<POLine>.View POLineUpdateView;
public PXAction<InventoryItem> UpdatePOLine;
nPXButton]
oPXUIField(DisplayName = "Update POLine")]
public virtual IEnumerable updatePOLine(PXAdapter adapter)
{
List<POLine> l = POLineUpdateView.Select().RowCast<POLine>().ToList<POLine>();
POLineUpdateView.Current = l.First();
POLineUpdateView.Current.TranDesc = String.Concat(POLineUpdateView.Current.TranDesc, "X");
POLineUpdateView.UpdateCurrent();
POLineUpdateView.Cache.Persist(PXDBOperation.Update);
return adapter.Get();
}
The error says: Error: 'OrderDate' cannot be empty.
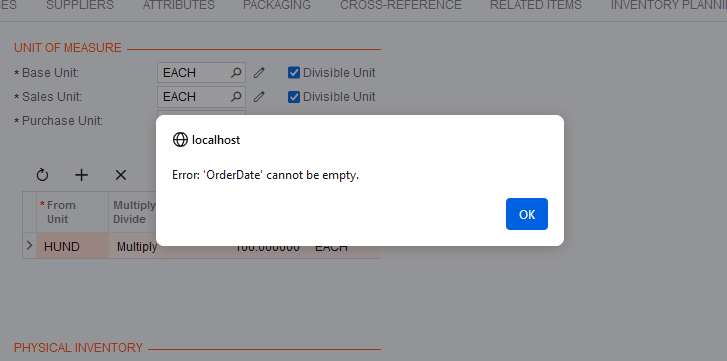
I can see that OrderDate has a value before the Persist method is called, but is null afterwards. I cannot understand why this is happening. I’ve checked the POLine DAC but cannot see how updating a record would affect the OrderDate field.
Can anyone explain this?