Hi I have a custom checkbox created in the Stock Items screen under the manufacturing screen, when this is checked I need the "Allow Preassigning Lot/Serial Numbers" to be automatically checked as well in the Production Order Maintenance screen. My code works but when the event handler is triggered it clears out the other header fields such as the Inventory ID. How can I fix this?
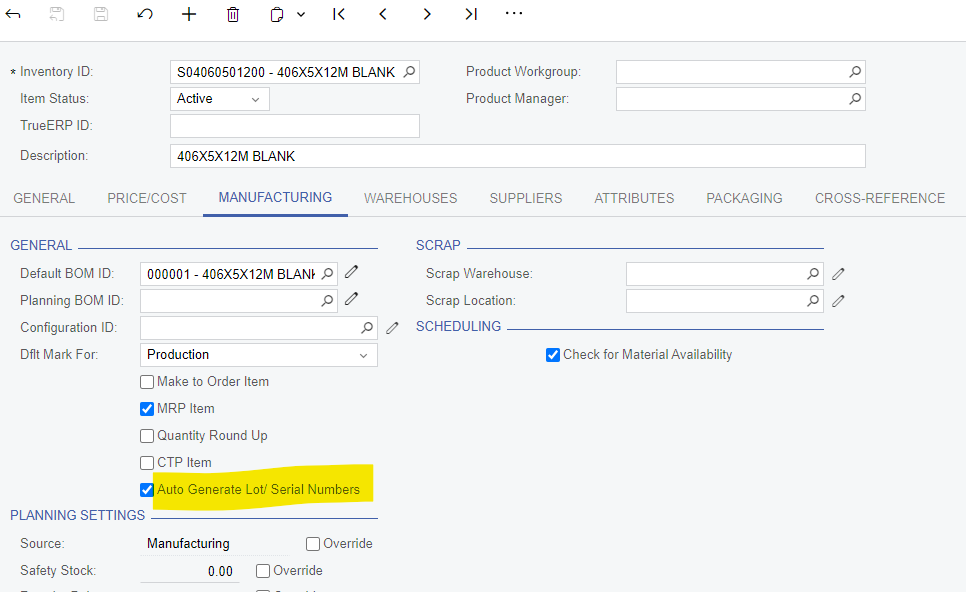
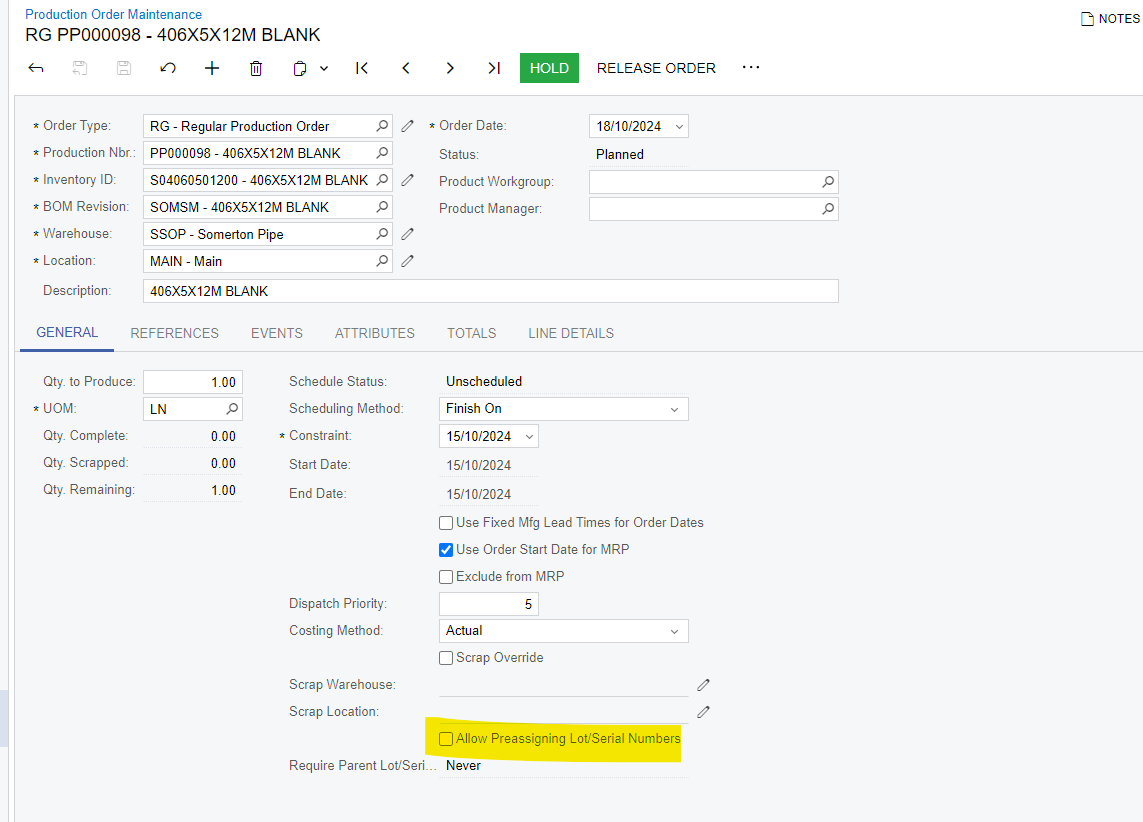
using PX.Objects.Common.Scopes;
using PX.Objects.PM;
using IQtyAllocated = PX.Objects.IN.Overrides.INDocumentRelease.IQtyAllocated;
using LocationStatus = PX.Objects.IN.Overrides.INDocumentRelease.LocationStatus;
using LotSerialStatus = PX.Objects.IN.Overrides.INDocumentRelease.LotSerialStatus;
using SiteLotSerial = PX.Objects.IN.Overrides.INDocumentRelease.SiteLotSerial;
using SiteStatus = PX.Objects.IN.Overrides.INDocumentRelease.SiteStatus;
using System;
using PX.Data;
using PX.Objects.IN;
using PX.Objects.CS;
using System.Collections;
using System.Collections.Generic;
using System.Text;
using PX.Objects.AM.GraphExtensions;
using PX.Common;
using PX.Objects.Common;
using PX.Objects.SO;
using PX.Objects.AM.Attributes;
using System.Linq;
using PX.Objects.AM.CacheExtensions;
using PX.Data.BQL.Fluent;
using PX.Data.BQL;
using PX.Objects.AR;
using PX.Objects.CR;
using PX.Objects.GL;
using PX.Objects;
using PX.Objects.AM;
using INInventoryItemExt = PX.Objects.IN.InventoryItemExt;
namespace PX.Objects.AM
{
public class ProdMaint_Extension : PXGraphExtension<ProdMaint.ItemLineSplittingExtension,PX.Objects.AM.ProdMaint>
{
protected virtual void AMProdItem_InventoryID_FieldUpdated(PXCache cache, PXFieldUpdatedEventArgs e)
{
var row = (AMProdItem)e.Row;
if (row?.InventoryID == null) return;
var item = InventoryItem.PK.Find(Base, row.InventoryID);
if (item == null) return;
var itemExt = PXCache<InventoryItem>.GetExtension<INInventoryItemExt>(item);
cache.SetValueExt<AMProdItem.preassignLotSerial>(row, itemExt.UsrAutoGenerateNumberChecker == true);
}
}
}